OpeningDisk3d
Performs a three-dimensional opening using a structuring element matching with an oriented disk.
Access to parameter description
For an introduction:
The opening disk normal is oriented in the direction given by ($\theta$, $\varphi$) in spherical coordinates as often used in mathematics (azimuthal angle, $\theta$ and polar angle, $\varphi$). This direction is described with the following formula: $$V=\left[\begin{array}{c}; v_x\\ v_y\\ v_z\end{array}\right] = \left[\begin{array}{c}; \sin(\varphi)\cos(\theta)\\ \sin(\varphi)\sin(\theta)\\ \cos(\varphi)\end{array}\right]$$ This direction can be illustrated on the unit sphere:
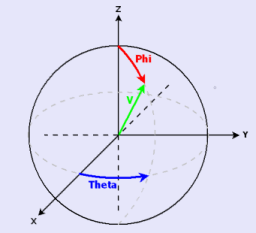
Figure 1. Azimuthal and polar angles on the unit sphere
With a classic implementation, morphological opening systematically considers areas out of the image as a replication of the image borders at each step of the algorithm. Therefore, when applying an opening, some thin object parts cut by the image borders may be removed at the erosion step and not be restored after the dilation, while one would expect to keep them. The borderPolicy parameter manages this case. The default mode, LIMITED, corresponds to the classic behavior. The EXTENDED mode manages properly image borders by extending them by a size equal to the structuring element's. This mode can be slower and more memory consuming, especially when the structuring element size is high.
This option is illustrated in the Opening2d documentation (Figure 2).
See also
Access to parameter description
For an introduction:
- section Mathematical Morphology
- section Introduction To Opening
The opening disk normal is oriented in the direction given by ($\theta$, $\varphi$) in spherical coordinates as often used in mathematics (azimuthal angle, $\theta$ and polar angle, $\varphi$). This direction is described with the following formula: $$V=\left[\begin{array}{c}; v_x\\ v_y\\ v_z\end{array}\right] = \left[\begin{array}{c}; \sin(\varphi)\cos(\theta)\\ \sin(\varphi)\sin(\theta)\\ \cos(\varphi)\end{array}\right]$$ This direction can be illustrated on the unit sphere:
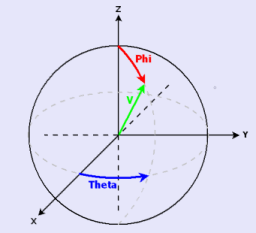
Figure 1. Azimuthal and polar angles on the unit sphere
With a classic implementation, morphological opening systematically considers areas out of the image as a replication of the image borders at each step of the algorithm. Therefore, when applying an opening, some thin object parts cut by the image borders may be removed at the erosion step and not be restored after the dilation, while one would expect to keep them. The borderPolicy parameter manages this case. The default mode, LIMITED, corresponds to the classic behavior. The EXTENDED mode manages properly image borders by extending them by a size equal to the structuring element's. This mode can be slower and more memory consuming, especially when the structuring element size is high.
This option is illustrated in the Opening2d documentation (Figure 2).
See also
Function Syntax
This function returns the outputImage output parameter.
// Function prototype. std::shared_ptr< iolink::ImageView > openingDisk3d( std::shared_ptr< iolink::ImageView > inputImage, double thetaAngle, double phiAngle, uint32_t kernelRadius, OpeningDisk3d::BorderPolicy borderPolicy, std::shared_ptr< iolink::ImageView > outputImage = NULL );
This function returns the outputImage output parameter.
// Function prototype. opening_disk_3d( input_image, theta_angle = 0, phi_angle = 0, kernel_radius = 3, border_policy = OpeningDisk3d.BorderPolicy.LIMITED, output_image = None )
This function returns the outputImage output parameter.
// Function prototype. public static IOLink.ImageView OpeningDisk3d( IOLink.ImageView inputImage, double thetaAngle = 0, double phiAngle = 0, UInt32 kernelRadius = 3, OpeningDisk3d.BorderPolicy borderPolicy = ImageDev.OpeningDisk3d.BorderPolicy.LIMITED, IOLink.ImageView outputImage = null );
Class Syntax
Parameters
Class Name | OpeningDisk3d |
---|
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input image. The image type can be integer or float. | Image | Binary, Label, Grayscale or Multispectral | nullptr | ||||
![]() |
borderPolicy |
The border policy to apply.
|
Enumeration | LIMITED | |||||
![]() |
thetaAngle |
The azimuthal angle in degrees. | Float64 | Any value | 0 | ||||
![]() |
phiAngle |
The polar angle in degrees. | Float64 | Any value | 0 | ||||
![]() |
kernelRadius |
The length of the disk radius in voxels. | UInt32 | >=1 | 3 | ||||
![]() |
outputImage |
The output image. Its dimensions and type are forced to the same values as the input image. | Image | nullptr |
Object Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); OpeningDisk3d openingDisk3dAlgo; openingDisk3dAlgo.setInputImage( foam ); openingDisk3dAlgo.setThetaAngle( 0 ); openingDisk3dAlgo.setPhiAngle( 0 ); openingDisk3dAlgo.setKernelRadius( 3 ); openingDisk3dAlgo.setBorderPolicy( OpeningDisk3d::BorderPolicy::EXTENDED ); openingDisk3dAlgo.execute(); std::cout << "outputImage:" << openingDisk3dAlgo.outputImage()->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) opening_disk_3d_algo = imagedev.OpeningDisk3d() opening_disk_3d_algo.input_image = foam opening_disk_3d_algo.theta_angle = 0 opening_disk_3d_algo.phi_angle = 0 opening_disk_3d_algo.kernel_radius = 3 opening_disk_3d_algo.border_policy = imagedev.OpeningDisk3d.EXTENDED opening_disk_3d_algo.execute() print( "output_image:", str( opening_disk_3d_algo.output_image ) );
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); OpeningDisk3d openingDisk3dAlgo = new OpeningDisk3d { inputImage = foam, thetaAngle = 0, phiAngle = 0, kernelRadius = 3, borderPolicy = OpeningDisk3d.BorderPolicy.EXTENDED }; openingDisk3dAlgo.Execute(); Console.WriteLine( "outputImage:" + openingDisk3dAlgo.outputImage.ToString() );
Function Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto result = openingDisk3d( foam, 0, 0, 3, OpeningDisk3d::BorderPolicy::EXTENDED ); std::cout << "outputImage:" << result->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) result = imagedev.opening_disk_3d( foam, 0, 0, 3, imagedev.OpeningDisk3d.EXTENDED ) print( "output_image:", str( result ) );
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); IOLink.ImageView result = Processing.OpeningDisk3d( foam, 0, 0, 3, OpeningDisk3d.BorderPolicy.EXTENDED ); Console.WriteLine( "outputImage:" + result.ToString() );