TopHat
Detects the compact dark or bright areas of a grayscale image.
Access to parameter description
The Top-Hat transformation allows detecting the dark areas of a grayscale image, or the valleys of function f, using the closing function.
By changing the size of the closing and the bounds of the threshold, one may choose the width and the depth of the selected valleys.
Similarly, this algorithm allows detecting the white areas of a grayscale image, or the narrow peaks of function f, using an opening.
By changing the values of the size of the opening and the bounds of the threshold, it is possible to choose the width and the height of the selected peaks.
It would be impossible to obtain the same result with a simple threshold.
The Top-Hat segmentation extracts small elements and details from given images. It detects the dark or the bright areas, corresponding to the valleys or the narrow peaks.
There are two types of Top-Hat transforms:
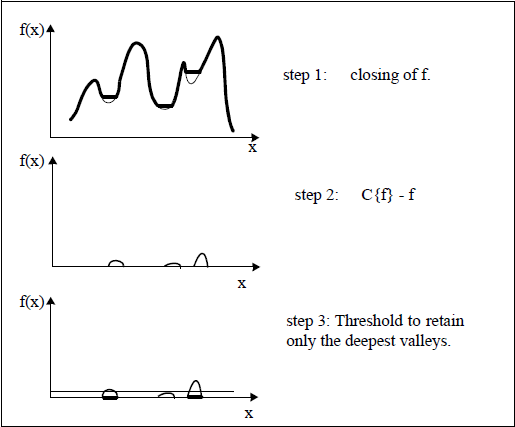
Figure 1. Black Top-Hat computation
The Top-Hat algorithm is divided into two phases:
Access to parameter description
The Top-Hat transformation allows detecting the dark areas of a grayscale image, or the valleys of function f, using the closing function.
By changing the size of the closing and the bounds of the threshold, one may choose the width and the depth of the selected valleys.
Similarly, this algorithm allows detecting the white areas of a grayscale image, or the narrow peaks of function f, using an opening.
By changing the values of the size of the opening and the bounds of the threshold, it is possible to choose the width and the height of the selected peaks.
It would be impossible to obtain the same result with a simple threshold.
The Top-Hat segmentation extracts small elements and details from given images. It detects the dark or the bright areas, corresponding to the valleys or the narrow peaks.
There are two types of Top-Hat transforms:
- The Black Top-Hat: it is defined as the difference between the closing with a particular kernel and the input image. The smaller the kernel, the smaller the elements detected in the Top-Hat image. A threshold allows selecting the darker elements of the Top-Hat result (the depth of the selected valleys), as shown in Figure 1.
- The White Top-Hat: it is defined as the difference between the input image and its opening (using a particular kernel). The threshold allows selecting the brighter elements of the Top-Hat result.
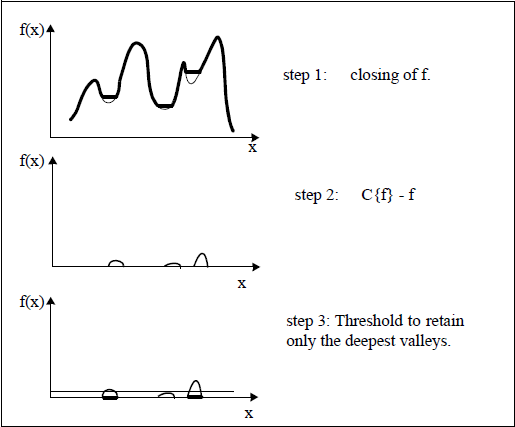
Figure 1. Black Top-Hat computation
The Top-Hat algorithm is divided into two phases:
- The Top-Hat image computation, depending on the Top-Hat type and the closing/opening parameters (steps 1 and 2 on Figure 1).
- The Thresholding, to select the depth of valleys or narrow peaks (step 3 on figure 1).
Function Syntax
This function returns the outputBinaryImage output parameter.
// Function prototype. std::shared_ptr< iolink::ImageView > topHat( std::shared_ptr< iolink::ImageView > inputGrayImage, int32_t kernelRadius, TopHat::ObjectLightness objectLightness, iolink::Vector2d thresholdRange, std::shared_ptr< iolink::ImageView > outputBinaryImage = NULL );
Class Syntax
Parameters
Class Name | TopHat |
---|
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputGrayImage |
The input gray level image. | Image | Binary, Label or Grayscale | nullptr | ||||
![]() |
kernelRadius |
The half size of the structuring element in pixels. A square structuring element always has an odd side length (3x3, 5x5, etc.) which is defined by twice the kernel radius + 1. | Int32 | >=1 | 3 | ||||
![]() |
objectLightness |
The lightness of objects to detect.
|
Enumeration | BRIGHT_OBJECTS | |||||
![]() |
thresholdRange |
The low and high threshold levels. | Vector2d | Any value | {128.f, 255.f} | ||||
![]() |
outputBinaryImage |
The output binary image. Its dimensions are forced to the same values as the input. | Image | nullptr |
Object Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); TopHat topHatAlgo; topHatAlgo.setInputGrayImage( foam ); topHatAlgo.setKernelRadius( 3 ); topHatAlgo.setObjectLightness( TopHat::ObjectLightness::BRIGHT_OBJECTS ); topHatAlgo.setThresholdRange( {128.0, 255.0} ); topHatAlgo.execute(); std::cout << "outputBinaryImage:" << topHatAlgo.outputBinaryImage()->toString();
Function Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto result = topHat( foam, 3, TopHat::ObjectLightness::BRIGHT_OBJECTS, {128.0, 255.0} ); std::cout << "outputBinaryImage:" << result->toString();