Skeleton
Computes the morphological skeleton of objects contained in a binary image.
Access to parameter description
For an introduction:
Imagining that the set X is a dry herb prairie which starts to burn from all the points of its boundary, the skeleton is the line where the fires meet. Using the same analogy, the quench function can be defined as the time for the fire to reach this line.
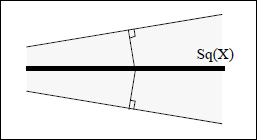
Figure 1. Sq(X) is the quench function
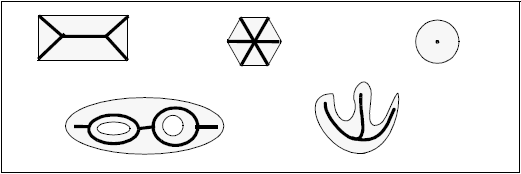
Figure 2. Some shapes and their skeletons
This algorithm performs a sequential homotopic thinning. The input image is iteratively thinned by an homotopic structuring element until idempotence. This structuring element is the L configuration of Golay's alphabet and its 8 associated rotations: 111×1×000 Where × means "don't care".
Note: This algorithm is described here in 2D but also can be launched on 3D images. Nevertheless, the skeleton definition given above is not always verified in the 3D case. Indeed the 3D skeleton is not systematically well centered. The Centerline3d algorithm can be used to get a more centered 3D skeleton.
See also
Access to parameter description
For an introduction:
- section Mathematical Morphology
- section Skeletonization
Imagining that the set X is a dry herb prairie which starts to burn from all the points of its boundary, the skeleton is the line where the fires meet. Using the same analogy, the quench function can be defined as the time for the fire to reach this line.
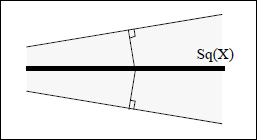
Figure 1. Sq(X) is the quench function
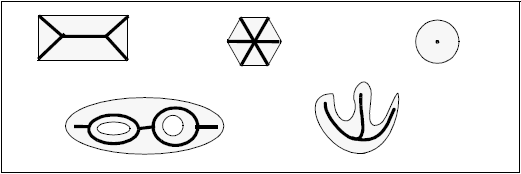
Figure 2. Some shapes and their skeletons
This algorithm performs a sequential homotopic thinning. The input image is iteratively thinned by an homotopic structuring element until idempotence. This structuring element is the L configuration of Golay's alphabet and its 8 associated rotations: 111×1×000 Where × means "don't care".
Note: This algorithm is described here in 2D but also can be launched on 3D images. Nevertheless, the skeleton definition given above is not always verified in the 3D case. Indeed the 3D skeleton is not systematically well centered. The Centerline3d algorithm can be used to get a more centered 3D skeleton.
See also
Function Syntax
This function returns the outputBinaryImage output parameter.
// Function prototype. std::shared_ptr< iolink::ImageView > skeleton( std::shared_ptr< iolink::ImageView > inputBinaryImage, std::shared_ptr< iolink::ImageView > outputBinaryImage = NULL );
Class Syntax
Parameters
Class Name | Skeleton |
---|
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputBinaryImage |
The binary input image. | Image | Binary | nullptr |
![]() |
outputBinaryImage |
The binary output image. Its size and type are forced to the same values as the input. | Image | nullptr |
Object Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); Skeleton skeletonAlgo; skeletonAlgo.setInputBinaryImage( polystyrene_sep ); skeletonAlgo.execute(); std::cout << "outputBinaryImage:" << skeletonAlgo.outputBinaryImage()->toString();
Function Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); auto result = skeleton( polystyrene_sep ); std::cout << "outputBinaryImage:" << result->toString();