GrayscaleReconstruction2d
Performs a two-dimensional numerical reconstruction, starting from a grayscale marker image, into a grayscale input image.
Access to parameter description
For an introduction:
This algorithm is based on a recursive method whereby the minimum between the dilated or eroded image and the mask image is retained and used as the marker image for the next step.
At each step, the marker image is dilated or eroded by a structuring element of size 1.
This operation is repeated until stability. Therefore, the number of iterations depends on the input image and the marker image.
As shown in Figure 1, peaks and ridges are levelled down with a reconstruction by dilation, which results in a much more homogeneous output image.
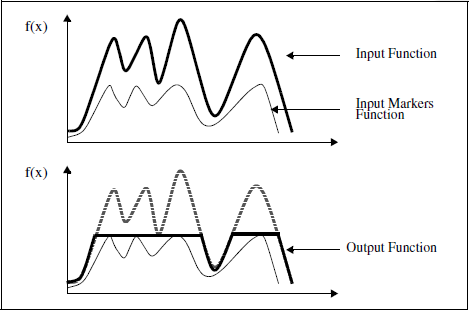
Figure 1. One-dimensional example of a reconstruction by dilation
As shown in Figure 2, the valleys are leveled up with a reconstruction by erosion, which results in a much more homogeneous output image.
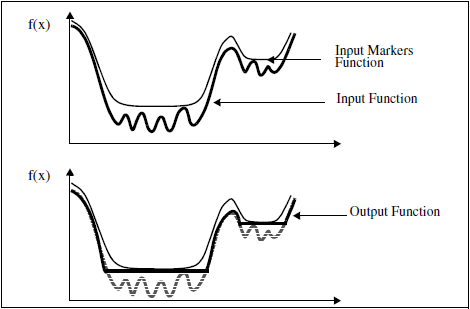
Figure 2. One-dimensional example of a reconstruction by erosion
See also
Access to parameter description
For an introduction:
- section Mathematical Morphology
- section Geodesic Transformations
This algorithm is based on a recursive method whereby the minimum between the dilated or eroded image and the mask image is retained and used as the marker image for the next step.
At each step, the marker image is dilated or eroded by a structuring element of size 1.
This operation is repeated until stability. Therefore, the number of iterations depends on the input image and the marker image.
As shown in Figure 1, peaks and ridges are levelled down with a reconstruction by dilation, which results in a much more homogeneous output image.
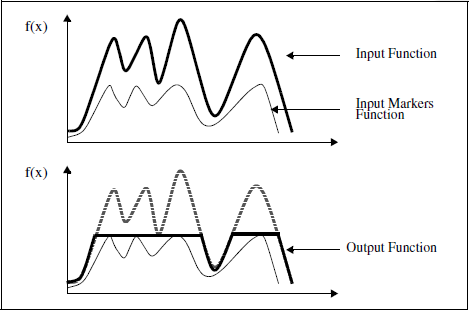
Figure 1. One-dimensional example of a reconstruction by dilation
As shown in Figure 2, the valleys are leveled up with a reconstruction by erosion, which results in a much more homogeneous output image.
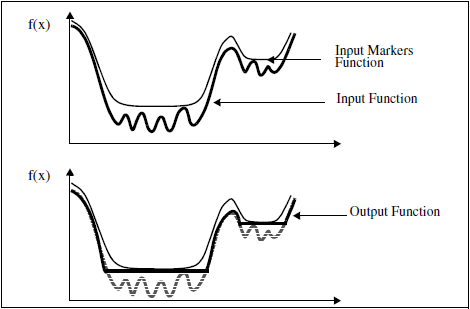
Figure 2. One-dimensional example of a reconstruction by erosion
See also
Function Syntax
This function returns the outputImage output parameter.
// Function prototype. std::shared_ptr< iolink::ImageView > grayscaleReconstruction2d( std::shared_ptr< iolink::ImageView > inputImage, std::shared_ptr< iolink::ImageView > inputMarkerImage, GrayscaleReconstruction2d::ReconstructionType reconstructionType, GrayscaleReconstruction2d::Neighborhood neighborhood, std::shared_ptr< iolink::ImageView > outputImage = NULL );
This function returns the outputImage output parameter.
// Function prototype. grayscale_reconstruction_2d( input_image, input_marker_image, reconstruction_type = GrayscaleReconstruction2d.ReconstructionType.DILATION, neighborhood = GrayscaleReconstruction2d.Neighborhood.CONNECTIVITY_8, output_image = None )
This function returns the outputImage output parameter.
// Function prototype. public static IOLink.ImageView GrayscaleReconstruction2d( IOLink.ImageView inputImage, IOLink.ImageView inputMarkerImage, GrayscaleReconstruction2d.ReconstructionType reconstructionType = ImageDev.GrayscaleReconstruction2d.ReconstructionType.DILATION, GrayscaleReconstruction2d.Neighborhood neighborhood = ImageDev.GrayscaleReconstruction2d.Neighborhood.CONNECTIVITY_8, IOLink.ImageView outputImage = null );
Class Syntax
Parameters
Class Name | GrayscaleReconstruction2d |
---|
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The grayscale input mask image constraining reconstruction. | Image | Binary, Label or Grayscale | nullptr | ||||
![]() |
inputMarkerImage |
The grayscale input marker image containing seeds for reconstruction. It must have the same dimensions and type as the input image. | Image | Binary, Label, Grayscale or Multispectral | nullptr | ||||
![]() |
reconstructionType |
The type of reconstruction algorithm to apply.
|
Enumeration | DILATION | |||||
![]() |
neighborhood |
The 2D neighborhood configuration for performing dilations or erosions.
|
Enumeration | CONNECTIVITY_8 | |||||
![]() |
outputImage |
The output image. Its dimensions and type are forced to the same values as the input. | Image | nullptr |
Object Examples
std::shared_ptr< iolink::ImageView > polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); GrayscaleReconstruction2d grayscaleReconstruction2dAlgo; grayscaleReconstruction2dAlgo.setInputImage( polystyrene ); grayscaleReconstruction2dAlgo.setInputMarkerImage( polystyrene ); grayscaleReconstruction2dAlgo.setReconstructionType( GrayscaleReconstruction2d::ReconstructionType::DILATION ); grayscaleReconstruction2dAlgo.setNeighborhood( GrayscaleReconstruction2d::Neighborhood::CONNECTIVITY_8 ); grayscaleReconstruction2dAlgo.execute(); std::cout << "outputImage:" << grayscaleReconstruction2dAlgo.outputImage()->toString();
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) grayscale_reconstruction_2d_algo = imagedev.GrayscaleReconstruction2d() grayscale_reconstruction_2d_algo.input_image = polystyrene grayscale_reconstruction_2d_algo.input_marker_image = polystyrene grayscale_reconstruction_2d_algo.reconstruction_type = imagedev.GrayscaleReconstruction2d.DILATION grayscale_reconstruction_2d_algo.neighborhood = imagedev.GrayscaleReconstruction2d.CONNECTIVITY_8 grayscale_reconstruction_2d_algo.execute() print( "output_image:", str( grayscale_reconstruction_2d_algo.output_image ) );
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); GrayscaleReconstruction2d grayscaleReconstruction2dAlgo = new GrayscaleReconstruction2d { inputImage = polystyrene, inputMarkerImage = polystyrene, reconstructionType = GrayscaleReconstruction2d.ReconstructionType.DILATION, neighborhood = GrayscaleReconstruction2d.Neighborhood.CONNECTIVITY_8 }; grayscaleReconstruction2dAlgo.Execute(); Console.WriteLine( "outputImage:" + grayscaleReconstruction2dAlgo.outputImage.ToString() );
Function Examples
std::shared_ptr< iolink::ImageView > polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); auto result = grayscaleReconstruction2d( polystyrene, polystyrene, GrayscaleReconstruction2d::ReconstructionType::DILATION, GrayscaleReconstruction2d::Neighborhood::CONNECTIVITY_8 ); std::cout << "outputImage:" << result->toString();
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) result = imagedev.grayscale_reconstruction_2d( polystyrene, polystyrene, imagedev.GrayscaleReconstruction2d.DILATION, imagedev.GrayscaleReconstruction2d.CONNECTIVITY_8 ) print( "output_image:", str( result ) );
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); IOLink.ImageView result = Processing.GrayscaleReconstruction2d( polystyrene, polystyrene, GrayscaleReconstruction2d.ReconstructionType.DILATION, GrayscaleReconstruction2d.Neighborhood.CONNECTIVITY_8 ); Console.WriteLine( "outputImage:" + result.ToString() );