CylindricalIntensityProfile3d
Computes a radial intensity profile on a 3D image by performing a projection along an axis.
Access to parameter description
This algorithm computes an intensity profile that consists in the average intensity at a distance r from a given center. It is computed on a 2D projection of the input volume performed by averaging intensity values along a user-defined axis.
The output is a 2-column spreadsheet indicating, for each radius, the corresponding average intensity value.
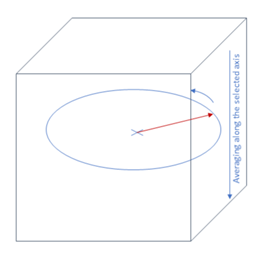
Figure 1. Cylindrical intensity profile along a given axis
See also
Access to parameter description
This algorithm computes an intensity profile that consists in the average intensity at a distance r from a given center. It is computed on a 2D projection of the input volume performed by averaging intensity values along a user-defined axis.
The output is a 2-column spreadsheet indicating, for each radius, the corresponding average intensity value.
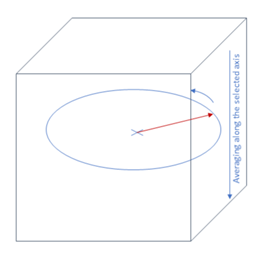
Figure 1. Cylindrical intensity profile along a given axis
See also
Function Syntax
This function returns the outputMeasurement output parameter.
// Function prototype. CylindricalIntensityProfileMsr::Ptr cylindricalIntensityProfile3d( std::shared_ptr< iolink::ImageView > inputImage, CylindricalIntensityProfile3d::AxisDirection axisDirection, CylindricalIntensityProfile3d::CenterMode centerMode, iolink::Vector3u32 center, uint32_t maximumRadius, CylindricalIntensityProfileMsr::Ptr outputMeasurement = NULL );
This function returns the outputMeasurement output parameter.
// Function prototype. cylindrical_intensity_profile_3d( input_image, axis_direction = CylindricalIntensityProfile3d.AxisDirection.Z_AXIS, center_mode = CylindricalIntensityProfile3d.CenterMode.IMAGE_CENTER, center = [0, 0, 0], maximum_radius = 0, output_measurement = None )
This function returns the outputMeasurement output parameter.
// Function prototype. public static CylindricalIntensityProfileMsr CylindricalIntensityProfile3d( IOLink.ImageView inputImage, CylindricalIntensityProfile3d.AxisDirection axisDirection = ImageDev.CylindricalIntensityProfile3d.AxisDirection.Z_AXIS, CylindricalIntensityProfile3d.CenterMode centerMode = ImageDev.CylindricalIntensityProfile3d.CenterMode.IMAGE_CENTER, uint[] center = null, UInt32 maximumRadius = 0, CylindricalIntensityProfileMsr outputMeasurement = null );
Class Syntax
Parameters
Class Name | CylindricalIntensityProfile3d |
---|
Parameter Name | Description | Type | Supported Values | Default Value | |||||||
---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input image. Any type of image is accepted. | Image | Grayscale or Multispectral | nullptr | ||||||
![]() |
centerMode |
The way to define the origin of the radii.
|
Enumeration | IMAGE_CENTER | |||||||
![]() |
axisDirection |
The axis along which slices are projected.
If axis direction is set to X, YZ slices are projected.
|
Enumeration | Z_AXIS | |||||||
![]() |
center |
The origin of the radii in pixel coordinates. It is only used in OTHER mode. | Vector3u32 | Any value | {0, 0, 0} | ||||||
![]() |
maximumRadius |
The radius in voxels up to which intensities are included in the profile. | UInt32 | Any value | 0 | ||||||
![]() |
outputMeasurement |
The output object containing the computed radii and averaged intensities. | CylindricalIntensityProfileMsr | nullptr |
Object Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); CylindricalIntensityProfile3d cylindricalIntensityProfile3dAlgo; cylindricalIntensityProfile3dAlgo.setInputImage( foam ); cylindricalIntensityProfile3dAlgo.setAxisDirection( CylindricalIntensityProfile3d::AxisDirection::Z_AXIS ); cylindricalIntensityProfile3dAlgo.setCenterMode( CylindricalIntensityProfile3d::CenterMode::IMAGE_CENTER ); cylindricalIntensityProfile3dAlgo.setCenter( {0, 0, 0} ); cylindricalIntensityProfile3dAlgo.setMaximumRadius( 255 ); cylindricalIntensityProfile3dAlgo.execute(); std::cout << "mean: " << cylindricalIntensityProfile3dAlgo.outputMeasurement()->mean( 0 , 0 , 0 ) ;
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) cylindrical_intensity_profile_3d_algo = imagedev.CylindricalIntensityProfile3d() cylindrical_intensity_profile_3d_algo.input_image = foam cylindrical_intensity_profile_3d_algo.axis_direction = imagedev.CylindricalIntensityProfile3d.Z_AXIS cylindrical_intensity_profile_3d_algo.center_mode = imagedev.CylindricalIntensityProfile3d.IMAGE_CENTER cylindrical_intensity_profile_3d_algo.center = [0, 0, 0] cylindrical_intensity_profile_3d_algo.maximum_radius = 255 cylindrical_intensity_profile_3d_algo.execute() print( print("mean: ", cylindrical_intensity_profile_3d_algo.output_measurement.mean( 0 , 0 , 0 ) ) );
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); CylindricalIntensityProfile3d cylindricalIntensityProfile3dAlgo = new CylindricalIntensityProfile3d { inputImage = foam, axisDirection = CylindricalIntensityProfile3d.AxisDirection.Z_AXIS, centerMode = CylindricalIntensityProfile3d.CenterMode.IMAGE_CENTER, center = new uint[]{0, 0, 0}, maximumRadius = 255 }; cylindricalIntensityProfile3dAlgo.Execute(); Console.WriteLine( "mean: " + cylindricalIntensityProfile3dAlgo.outputMeasurement.mean( 0 , 0 , 0 ) );
Function Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto result = cylindricalIntensityProfile3d( foam, CylindricalIntensityProfile3d::AxisDirection::Z_AXIS, CylindricalIntensityProfile3d::CenterMode::IMAGE_CENTER, {0, 0, 0}, 255 ); std::cout << "mean: " << result->mean( 0 , 0 , 0 ) ;
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) result = imagedev.cylindrical_intensity_profile_3d( foam, imagedev.CylindricalIntensityProfile3d.Z_AXIS, imagedev.CylindricalIntensityProfile3d.IMAGE_CENTER, [0, 0, 0], 255 ) print( "mean: ", result.mean( 0 , 0 , 0 ) );
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); CylindricalIntensityProfileMsr result = Processing.CylindricalIntensityProfile3d( foam, CylindricalIntensityProfile3d.AxisDirection.Z_AXIS, CylindricalIntensityProfile3d.CenterMode.IMAGE_CENTER, new uint[]{0, 0, 0}, 255 ); Console.WriteLine( "mean: " + result.mean( 0 , 0 , 0 ) );