ImageFormula
Computes a new image by applying a user-defined formula on one, two, or three input images.
Access to parameter description
The formula used by this algorithm must be written in accordance with the custom formula syntax.
Some of the examples below can be implemented with an existing algorithm. In these cases, using the appropriate native algorithm will be more efficient in terms of performance.
For instance, the formula "if(I2==25, I1, 0)" masks an input gray level image I1 with the blob of intensity 25 in a label image I2, as shown in Figure 1.
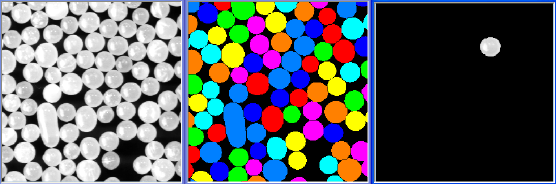
Figure 1. Extraction of a labeled blob in a graylevel image.
Where:
For instance, the formula "avg(I1[7, 7])" filters an image I1 by a box filter of size 7x7, as shown in Figure 2.
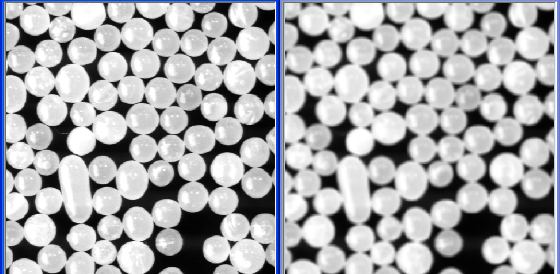
Figure 2. Box filter processing.
Where:
These offsets can be used to compute operations depending on pixels surrounding the current pixel to process.
For instance, a basic horizontal gradient operation can be written "I1(1, 0) - I1(-1, 0)", as shown in Figure 3.
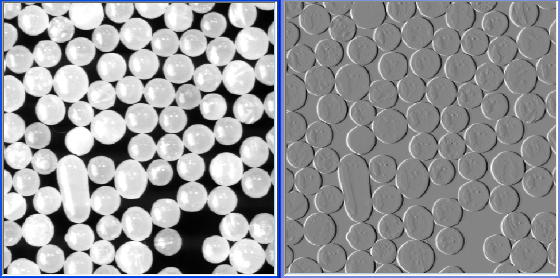
Figure 3. Basic horizontal gradient operation.
For instance, these coordinates can be used to generate synthetic images. A basic line separation can be written as "if (3x-100 < y, 100, 0)", as shown in Figure 4.
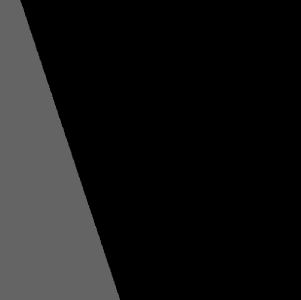
Figure 4. Generation of a synthetic image.
Coordinates also can be used to handle more complex cases such as "if ((x-(ox+gx)/2)*(x-(ox+gx)/2)+(y-(oy+gy)/2)*(y-(oy+gy)/2) < 5050, I1, I2)", as shown in Figure 5.
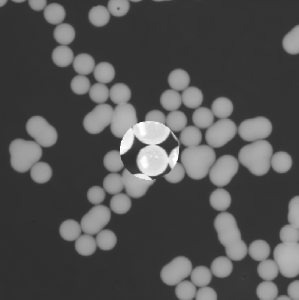
Figure 5. Custom combination of 2 images.
See also
Access to parameter description
The formula used by this algorithm must be written in accordance with the custom formula syntax.
Some of the examples below can be implemented with an existing algorithm. In these cases, using the appropriate native algorithm will be more efficient in terms of performance.
Basic usage
This algorithm accepts three input images that are referenced as I1, I2 and I3 in the formula. Images two and three are optional and must be provided only if referenced in the formula.For instance, the formula "if(I2==25, I1, 0)" masks an input gray level image I1 with the blob of intensity 25 in a label image I2, as shown in Figure 1.
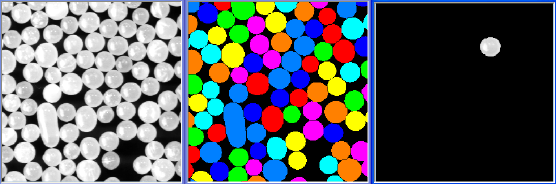
Figure 1. Extraction of a labeled blob in a graylevel image.
Usage with kernels
Functions with a variable number of arguments such as min, max, avg, median, sum and product can be used in two ways :- To combine images, for instance "min(I1, I2)"
- To compute kernel operations : "min(I1[7, 7])"
Where:
- N is the index of the related image (N $\in$ [1 .. 3]).
- x,y and z correspond to the expected kernel size.
For instance, the formula "avg(I1[7, 7])" filters an image I1 by a box filter of size 7x7, as shown in Figure 2.
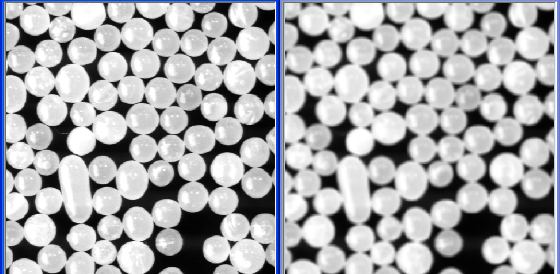
Figure 2. Box filter processing.
Usage with offsets
Image offset can be defined in formula respecting the following syntax : IN(x,y) in the 2D case and IN(x,y,z) in the 3D case.Where:
- N is the index of the related image (N $\in$ [1 .. 3]).
- x,y and z correspond to the offsets from the central current pixel.
These offsets can be used to compute operations depending on pixels surrounding the current pixel to process.
For instance, a basic horizontal gradient operation can be written "I1(1, 0) - I1(-1, 0)", as shown in Figure 3.
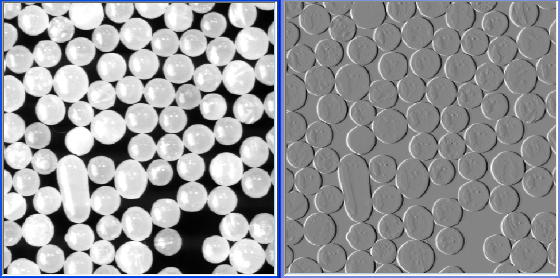
Figure 3. Basic horizontal gradient operation.
Usage of image coordinates
Image coordinates can be used in a formula: x, y and z represent the current pixel coordinates, ox, oy and oz the image origin and gx, gy and gz the image dimensions.For instance, these coordinates can be used to generate synthetic images. A basic line separation can be written as "if (3x-100 < y, 100, 0)", as shown in Figure 4.
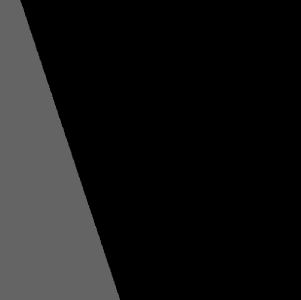
Figure 4. Generation of a synthetic image.
Coordinates also can be used to handle more complex cases such as "if ((x-(ox+gx)/2)*(x-(ox+gx)/2)+(y-(oy+gy)/2)*(y-(oy+gy)/2) < 5050, I1, I2)", as shown in Figure 5.
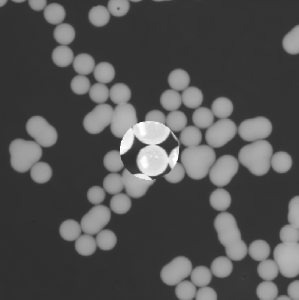
Figure 5. Custom combination of 2 images.
See also
Function Syntax
This function returns the outputImage output parameter.
// Function prototype. std::shared_ptr< iolink::ImageView > imageFormula( std::shared_ptr< iolink::ImageView > inputImage1, std::shared_ptr< iolink::ImageView > inputImage2, std::shared_ptr< iolink::ImageView > inputImage3, std::string formula, ImageFormula::OutputType outputType, std::shared_ptr< iolink::ImageView > outputImage = NULL );
This function returns the outputImage output parameter.
// Function prototype. image_formula( input_image1, input_image2, input_image3, formula = "", output_type = ImageFormula.OutputType.UNSIGNED_INTEGER_8_BIT, output_image = None )
This function returns the outputImage output parameter.
// Function prototype. public static IOLink.ImageView ImageFormula( IOLink.ImageView inputImage1, IOLink.ImageView inputImage2, IOLink.ImageView inputImage3, string formula = "", ImageFormula.OutputType outputType = ImageDev.ImageFormula.OutputType.UNSIGNED_INTEGER_8_BIT, IOLink.ImageView outputImage = null );
Class Syntax
Parameters
Class Name | ImageFormula |
---|
Parameter Name | Description | Type | Supported Values | Default Value | |||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage1 |
The first input image. | Image | Binary, Label, Grayscale or Multispectral | nullptr | ||||||||||||||||
![]() |
inputImage2 |
The second input image (optional). | Image | Binary, Label, Grayscale or Multispectral | nullptr | ||||||||||||||||
![]() |
inputImage3 |
The third input image (optional). | Image | Binary, Label, Grayscale or Multispectral | nullptr | ||||||||||||||||
![]() |
formula |
The formula to apply on image(s). | ImageFormula | "" | |||||||||||||||||
![]() |
outputType |
The output image data type.
|
Enumeration | UNSIGNED_INTEGER_8_BIT | |||||||||||||||||
![]() |
outputImage |
The output image. Its dimensions are forced to the same values as the input.Its data type is user-defined. | Image | nullptr |
Object Examples
std::shared_ptr< iolink::ImageView > polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); ImageFormula imageFormulaAlgo; imageFormulaAlgo.setInputImage1( polystyrene ); imageFormulaAlgo.setInputImage2( polystyrene ); imageFormulaAlgo.setInputImage3( polystyrene ); imageFormulaAlgo.setFormula( "I1+I2+I3" ); imageFormulaAlgo.setOutputType( ImageFormula::OutputType::FLOAT_32_BIT ); imageFormulaAlgo.execute(); std::cout << "outputImage:" << imageFormulaAlgo.outputImage()->toString();
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) image_formula_algo = imagedev.ImageFormula() image_formula_algo.input_image1 = polystyrene image_formula_algo.input_image2 = polystyrene image_formula_algo.input_image3 = polystyrene image_formula_algo.formula = "I1+I2+I3" image_formula_algo.output_type = imagedev.ImageFormula.FLOAT_32_BIT image_formula_algo.execute() print( "output_image:", str( image_formula_algo.output_image ) );
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); ImageFormula imageFormulaAlgo = new ImageFormula { inputImage1 = polystyrene, inputImage2 = polystyrene, inputImage3 = polystyrene, formula = "I1+I2+I3", outputType = ImageFormula.OutputType.FLOAT_32_BIT }; imageFormulaAlgo.Execute(); Console.WriteLine( "outputImage:" + imageFormulaAlgo.outputImage.ToString() );
Function Examples
std::shared_ptr< iolink::ImageView > polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); auto result = imageFormula( polystyrene, polystyrene, polystyrene, "I1+I2+I3", ImageFormula::OutputType::FLOAT_32_BIT ); std::cout << "outputImage:" << result->toString();
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) result = imagedev.image_formula( polystyrene, polystyrene, polystyrene, "I1+I2+I3", imagedev.ImageFormula.FLOAT_32_BIT ) print( "output_image:", str( result ) );
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); IOLink.ImageView result = Processing.ImageFormula( polystyrene, polystyrene, polystyrene, "I1+I2+I3", ImageFormula.OutputType.FLOAT_32_BIT ); Console.WriteLine( "outputImage:" + result.ToString() );