Centroid2d
Computes the centroid of objects in two-dimensional binary image.
Access to parameter description
For an introduction: For an object without holes, the centroid is a unique point. For an object with holes, the centroid is made of rings surrounding each hole, as shown in Figure 1. This algorithm performs successive thinnings with rotations and until convergence, using the D configuration: $$ \begin{array}{ccc} 0 & \times & \times\\ 0 & \bullet & \times\\ 0 & 0 & \times \end{array} ~~~ \mbox{followed by} ~~~~ \begin{array}{ccc} \times & 0 & \times\\ 0 & \bullet & 1\\ \times & 0 & \times \end{array} $$ Where $\times$ means "don't care".
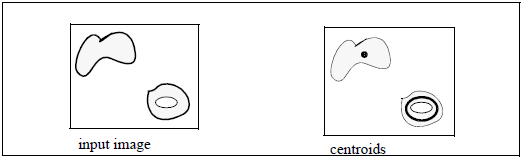
Figure 1. Centroids of objects with or without holes
Note: The centroid must not be confused with a classical center of gravity, which may be outside the object as in the case of a boomerang-shaped particle. However, for quasi-convex objects the centroid is very close to the center of gravity.
See also
Access to parameter description
For an introduction: For an object without holes, the centroid is a unique point. For an object with holes, the centroid is made of rings surrounding each hole, as shown in Figure 1. This algorithm performs successive thinnings with rotations and until convergence, using the D configuration: $$ \begin{array}{ccc} 0 & \times & \times\\ 0 & \bullet & \times\\ 0 & 0 & \times \end{array} ~~~ \mbox{followed by} ~~~~ \begin{array}{ccc} \times & 0 & \times\\ 0 & \bullet & 1\\ \times & 0 & \times \end{array} $$ Where $\times$ means "don't care".
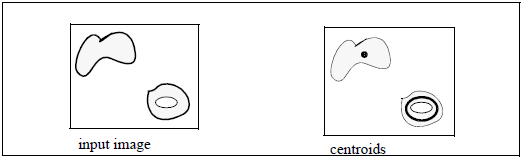
Figure 1. Centroids of objects with or without holes
Note: The centroid must not be confused with a classical center of gravity, which may be outside the object as in the case of a boomerang-shaped particle. However, for quasi-convex objects the centroid is very close to the center of gravity.
See also
Function Syntax
This function returns the outputBinaryImage output parameter.
// Function prototype. std::shared_ptr< iolink::ImageView > centroid2d( std::shared_ptr< iolink::ImageView > inputBinaryImage, std::shared_ptr< iolink::ImageView > outputBinaryImage = NULL );
This function returns the outputBinaryImage output parameter.
// Function prototype. centroid_2d( input_binary_image, output_binary_image = None )
This function returns the outputBinaryImage output parameter.
// Function prototype. public static IOLink.ImageView Centroid2d( IOLink.ImageView inputBinaryImage, IOLink.ImageView outputBinaryImage = null );
Class Syntax
Parameters
Class Name | Centroid2d |
---|
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputBinaryImage |
The binary input image. | Image | Binary | nullptr |
![]() |
outputBinaryImage |
The binary output image. Its size and type are forced to the same values as the input. | Image | nullptr |
Object Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); Centroid2d centroid2dAlgo; centroid2dAlgo.setInputBinaryImage( polystyrene_sep ); centroid2dAlgo.execute(); std::cout << "outputBinaryImage:" << centroid2dAlgo.outputBinaryImage()->toString();
polystyrene_sep = imagedev.read_vip_image(imagedev_data.get_image_path("polystyrene_sep.vip")) centroid_2d_algo = imagedev.Centroid2d() centroid_2d_algo.input_binary_image = polystyrene_sep centroid_2d_algo.execute() print( "output_binary_image:", str( centroid_2d_algo.output_binary_image ) );
ImageView polystyrene_sep = Data.ReadVipImage( @"Data/images/polystyrene_sep.vip" ); Centroid2d centroid2dAlgo = new Centroid2d { inputBinaryImage = polystyrene_sep }; centroid2dAlgo.Execute(); Console.WriteLine( "outputBinaryImage:" + centroid2dAlgo.outputBinaryImage.ToString() );
Function Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); auto result = centroid2d( polystyrene_sep ); std::cout << "outputBinaryImage:" << result->toString();
polystyrene_sep = imagedev.read_vip_image(imagedev_data.get_image_path("polystyrene_sep.vip")) result = imagedev.centroid_2d( polystyrene_sep ) print( "output_binary_image:", str( result ) );
ImageView polystyrene_sep = Data.ReadVipImage( @"Data/images/polystyrene_sep.vip" ); IOLink.ImageView result = Processing.Centroid2d( polystyrene_sep ); Console.WriteLine( "outputBinaryImage:" + result.ToString() );