HysteresisThresholding
Performs a thresholding with a conditional propagation.
Access to parameter description
For an introduction:
Two gray level values $\lambda_1$ and and $\lambda_2$ are specified.
The output $O$ is given by:
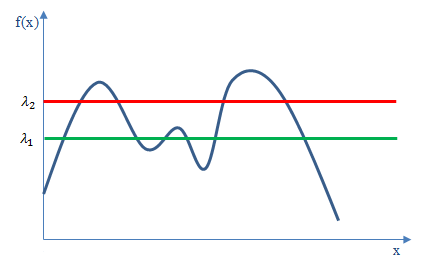
Figure 1. 1-D function with low and high thresholds
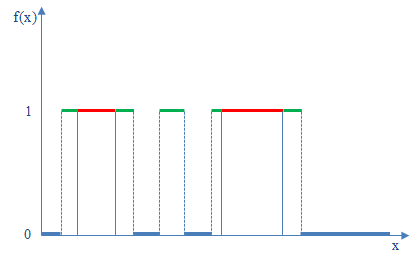
Figure 2. Retained area (red), rejected area (blue) and fuzzy area (green)
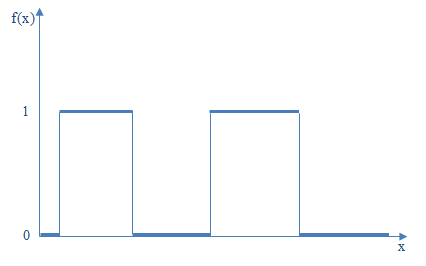
Figure 3. Hysteresis thresholding, unconnected fuzzy area rejected
This algorithm can be used after an edge detection, which generates, as well as edges, a lot of noise. True edges have a higher chance to be connected to a retained area than pixels corresponding to noise.
By the way, hysteresis thresholding is the last step of the Canny edge detector algorithm, following the non-maximum suppression step.
See also
Access to parameter description
For an introduction:
- section Image Gradient
- section Image Segmentation
- section Binarization
Two gray level values $\lambda_1$ and and $\lambda_2$ are specified.
The output $O$ is given by:
- If $I(n,m) > \lambda_2$, the point is in the retained area and is preserved: $O(n,m) = 1$
- If $I(n,m) <\lambda_1$, the point is in the rejected area: $O(n,m) = 0$
- If $ \lambda_1 \leq I(n,m) \leq \lambda_2 $, the point is in the fuzzy area.
- The point is preserved if connected to a retained area: $O(n,m) = 1$.
- The point is rejected if not connected to a retained area: $O(n,m) = 0$.
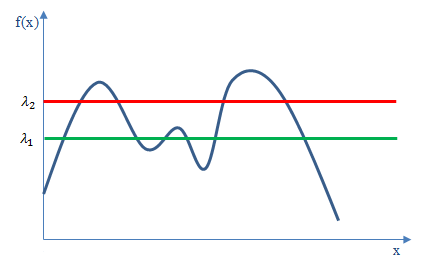
Figure 1. 1-D function with low and high thresholds
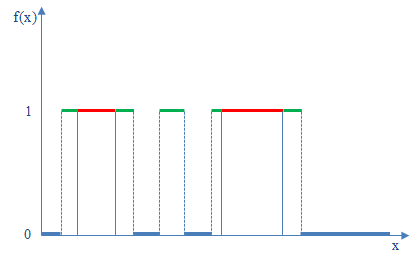
Figure 2. Retained area (red), rejected area (blue) and fuzzy area (green)
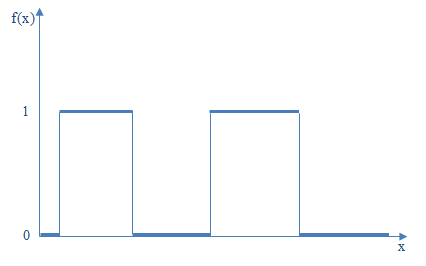
Figure 3. Hysteresis thresholding, unconnected fuzzy area rejected
This algorithm can be used after an edge detection, which generates, as well as edges, a lot of noise. True edges have a higher chance to be connected to a retained area than pixels corresponding to noise.
By the way, hysteresis thresholding is the last step of the Canny edge detector algorithm, following the non-maximum suppression step.
See also
Function Syntax
This function returns the outputBinaryImage output parameter.
// Function prototype. std::shared_ptr< iolink::ImageView > hysteresisThresholding( std::shared_ptr< iolink::ImageView > inputImage, iolink::Vector2d thresholdRange, int32_t length, std::shared_ptr< iolink::ImageView > outputBinaryImage = NULL );
This function returns the outputBinaryImage output parameter.
// Function prototype. hysteresis_thresholding( input_image, threshold_range = [255, 128], length = 1, output_binary_image = None )
This function returns the outputBinaryImage output parameter.
// Function prototype. public static IOLink.ImageView HysteresisThresholding( IOLink.ImageView inputImage, double[] thresholdRange = null, Int32 length = 1, IOLink.ImageView outputBinaryImage = null );
Class Syntax
Parameters
Class Name | HysteresisThresholding |
---|
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Grayscale | nullptr |
![]() |
thresholdRange |
The low and high threshold levels. | Vector2d | Any value | {255.f, 128.f} |
![]() |
length |
The maximum length in pixels allowed for considering points in the fuzzy zone (0:until convergence). | Int32 | >=0 | 1 |
![]() |
outputBinaryImage |
The output binary image. Its dimensions are forced to the same values as the input. | Image | nullptr |
Object Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); HysteresisThresholding hysteresisThresholdingAlgo; hysteresisThresholdingAlgo.setInputImage( foam ); hysteresisThresholdingAlgo.setThresholdRange( {128.0, 255.0} ); hysteresisThresholdingAlgo.setLength( 1 ); hysteresisThresholdingAlgo.execute(); std::cout << "outputBinaryImage:" << hysteresisThresholdingAlgo.outputBinaryImage()->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) hysteresis_thresholding_algo = imagedev.HysteresisThresholding() hysteresis_thresholding_algo.input_image = foam hysteresis_thresholding_algo.threshold_range = [128.0, 255.0] hysteresis_thresholding_algo.length = 1 hysteresis_thresholding_algo.execute() print( "output_binary_image:", str( hysteresis_thresholding_algo.output_binary_image ) );
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); HysteresisThresholding hysteresisThresholdingAlgo = new HysteresisThresholding { inputImage = foam, thresholdRange = new double[]{128.0, 255.0}, length = 1 }; hysteresisThresholdingAlgo.Execute(); Console.WriteLine( "outputBinaryImage:" + hysteresisThresholdingAlgo.outputBinaryImage.ToString() );
Function Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto result = hysteresisThresholding( foam, {128.0, 255.0}, 1 ); std::cout << "outputBinaryImage:" << result->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) result = imagedev.hysteresis_thresholding( foam, [128.0, 255.0], 1 ) print( "output_binary_image:", str( result ) );
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); IOLink.ImageView result = Processing.HysteresisThresholding( foam, new double[]{128.0, 255.0}, 1 ); Console.WriteLine( "outputBinaryImage:" + result.ToString() );