Centerline3d
Extracts the centerline of a binary three-dimensional image.
Access to parameter description
A centerline is a path with a tree topology that approximates the input shape. The extraction procedure enforces the path to be equidistant to its boundaries. A Euclidean distance map is computed to control the path centering.
This algorithm searches for a graph with tree topology (without loops). If objects contain loops, the algorithm does not fail; instead, it just ignores certain connectivity so that the tree topology of the graph is preserved.
The algorithm searches iteratively for the longest branch that can be added to the tree.
Secondary branches also can be detected, either by predefining the number of searched branches or by adding them automatically. In this last case, a new branch is added to the tree at a candidate crossing point, only if the length b is greater than a sensibility parameter multiplied by the distance a, where a and b are shown in Figure 1.
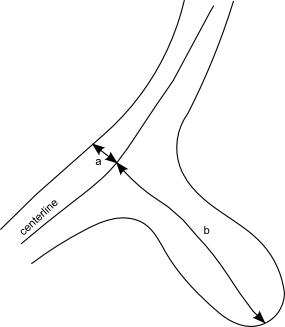
Figure 1. New branch sensibility
This algorithm generates two types of output:
Note: This algorithm must have the entire input data loaded in memory to be computed. Consequently, it cannot be used as part of an out-of-core processing recipe.
See also
Access to parameter description
A centerline is a path with a tree topology that approximates the input shape. The extraction procedure enforces the path to be equidistant to its boundaries. A Euclidean distance map is computed to control the path centering.
This algorithm searches for a graph with tree topology (without loops). If objects contain loops, the algorithm does not fail; instead, it just ignores certain connectivity so that the tree topology of the graph is preserved.
The algorithm searches iteratively for the longest branch that can be added to the tree.
Secondary branches also can be detected, either by predefining the number of searched branches or by adding them automatically. In this last case, a new branch is added to the tree at a candidate crossing point, only if the length b is greater than a sensibility parameter multiplied by the distance a, where a and b are shown in Figure 1.
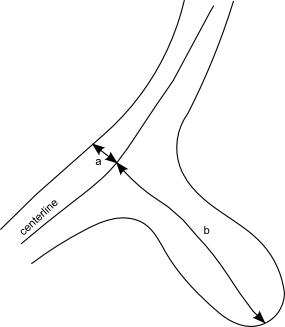
Figure 1. New branch sensibility
This algorithm generates two types of output:
- A binary or label image representing the detected centerlines by voxels having an intensity greater than or equal to 1.
- A geometric output modeling the centerlines by a set of polylines. This line set is provided by two output parameters: a list of vertices, and a list of indexes indicating how the vertices are connected.
Note: This algorithm must have the entire input data loaded in memory to be computed. Consequently, it cannot be used as part of an out-of-core processing recipe.
See also
Function Syntax
This function returns a Centerline3dOutput structure containing the outputIndices, outputVertices and outputObjectImage output parameters.
// Output structure. struct Centerline3dOutput { std::shared_ptr< iolink::ImageView > outputObjectImage; std::shared_ptr<iolink::ArrayXi64> outputIndices; std::shared_ptr<iolink::ArrayXd> outputVertices; }; // Function prototype. Centerline3dOutput centerline3d( std::shared_ptr< iolink::ImageView > inputObjectImage, bool autoMode, uint32_t numberOfBranches, double newBranchSensibility, double distanceMapSmoothing, uint32_t lineSetSmoothing, std::shared_ptr<iolink::ArrayXi64> outputIndices = NULL, std::shared_ptr<iolink::ArrayXd> outputVertices = NULL, std::shared_ptr< iolink::ImageView > outputObjectImage = NULL );
This function returns a tuple containing the output_indices, output_vertices and output_object_image output parameters.
// Function prototype. centerline_3d( input_object_image, auto_mode = True, number_of_branches = 3, new_branch_sensibility = 3, distance_map_smoothing = 0.3, line_set_smoothing = 3, output_indices = None, output_vertices = None, output_object_image = None )
This function returns a Centerline3dOutput structure containing the outputIndices, outputVertices and outputObjectImage output parameters.
/// Output structure of the Centerline3d function. public struct Centerline3dOutput { public IOLink.ImageView outputObjectImage; public IOLink.ArrayXi64 outputIndices; public IOLink.ArrayXd outputVertices; }; // Function prototype. public static Centerline3dOutput Centerline3d( IOLink.ImageView inputObjectImage, bool autoMode = true, UInt32 numberOfBranches = 3, double newBranchSensibility = 3, double distanceMapSmoothing = 0.3, UInt32 lineSetSmoothing = 3, IOLink.ArrayXi64 outputIndices = null, IOLink.ArrayXd outputVertices = null, IOLink.ImageView outputObjectImage = null );
Class Syntax
Parameters
Class Name | Centerline3d |
---|
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputObjectImage |
The binary or label input image. | Image | Binary or Label | nullptr | ||||
![]() |
autoMode |
The way to determine the number of branches.
|
Bool | true | |||||
![]() |
numberOfBranches |
The expected number of branches. This parameter is ignored if autoMode is set to YES. | UInt32 | >=1 | 3 | ||||
![]() |
newBranchSensibility |
The minimum length of a branch added automatically. This parameter is ignored if autoMode is set to NO. | Float64 | >=1 | 3 | ||||
![]() |
distanceMapSmoothing |
The smoothing level of the distance map. It controls the standard deviation of a Gaussian filter on the distance map, which is computed internally to make the tree centered. Smoothing the distance map can remove artifacts in the tree, like oscillations. | Float64 | >=0.01 | 0.3 | ||||
![]() |
lineSetSmoothing |
The number of smoothing iterations applied to the output polyline set. Each smoothing iteration applies a mean filter of size 3 on the vertices of the polylines. | UInt32 | Any value | 3 | ||||
![]() |
outputVertices |
The list of vertices of the output line set.
The indexation of the generated arrayX is {coordinate, index}, where coordinate is the axis index (0 for X, 1 for Y, and 2 for Z) and index is a label identifying the vertex. |
ArrayXd | nullptr | |||||
![]() |
outputIndices |
The list of indices of the output line set.
The vertices corresponding to two consecutive indices of this list are connected. An index that equals -1 indicates that the current polyline has ended and the next one begins. |
ArrayXi64 | nullptr | |||||
![]() |
outputObjectImage |
The output image. Its size and type are forced to the same values as the input. | Image | nullptr |
Object Examples
auto foam_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam_sep.vip" ); Centerline3d centerline3dAlgo; centerline3dAlgo.setInputObjectImage( foam_sep ); centerline3dAlgo.setAutoMode( true ); centerline3dAlgo.setNumberOfBranches( 1 ); centerline3dAlgo.setNewBranchSensibility( 3.0 ); centerline3dAlgo.setDistanceMapSmoothing( 1.0 ); centerline3dAlgo.setLineSetSmoothing( 3 ); centerline3dAlgo.execute(); std::cout << "outputIndices:" << centerline3dAlgo.outputIndices()->shape(); std::cout << "outputVertices:" << centerline3dAlgo.outputVertices()->shape(); std::cout << "outputObjectImage:" << centerline3dAlgo.outputObjectImage()->toString();
foam_sep = imagedev.read_vip_image(imagedev_data.get_image_path("foam_sep.vip")) centerline_3d_algo = imagedev.Centerline3d() centerline_3d_algo.input_object_image = foam_sep centerline_3d_algo.auto_mode = True centerline_3d_algo.number_of_branches = 1 centerline_3d_algo.new_branch_sensibility = 3.0 centerline_3d_algo.distance_map_smoothing = 1.0 centerline_3d_algo.line_set_smoothing = 3 centerline_3d_algo.execute() print( "output_indices:", str( centerline_3d_algo.output_indices ) ); print( "output_vertices:", str( centerline_3d_algo.output_vertices ) ); print( "output_object_image:", str( centerline_3d_algo.output_object_image ) );
ImageView foam_sep = Data.ReadVipImage( @"Data/images/foam_sep.vip" ); Centerline3d centerline3dAlgo = new Centerline3d { inputObjectImage = foam_sep, autoMode = true, numberOfBranches = 1, newBranchSensibility = 3.0, distanceMapSmoothing = 1.0, lineSetSmoothing = 3 }; centerline3dAlgo.Execute(); Console.WriteLine( "outputIndices:" + centerline3dAlgo.outputIndices.ToString() ); Console.WriteLine( "outputVertices:" + centerline3dAlgo.outputVertices.ToString() ); Console.WriteLine( "outputObjectImage:" + centerline3dAlgo.outputObjectImage.ToString() );
Function Examples
auto foam_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam_sep.vip" ); auto result = centerline3d( foam_sep, true, 1, 3.0, 1.0, 3 ); std::cout << "outputIndices:" << result.outputIndices->shape(); std::cout << "outputVertices:" << result.outputVertices->shape(); std::cout << "outputObjectImage:" << result.outputObjectImage->toString();
foam_sep = imagedev.read_vip_image(imagedev_data.get_image_path("foam_sep.vip")) result_output_indices, result_output_vertices, result_output_object_image = imagedev.centerline_3d( foam_sep, True, 1, 3.0, 1.0, 3 ) print( "output_indices:", str( result_output_indices ) ); print( "output_vertices:", str( result_output_vertices ) ); print( "output_object_image:", str( result_output_object_image ) );
ImageView foam_sep = Data.ReadVipImage( @"Data/images/foam_sep.vip" ); Processing.Centerline3dOutput result = Processing.Centerline3d( foam_sep, true, 1, 3.0, 1.0, 3 ); Console.WriteLine( "outputIndices:" + result.outputIndices.ToString() ); Console.WriteLine( "outputVertices:" + result.outputVertices.ToString() ); Console.WriteLine( "outputObjectImage:" + result.outputObjectImage.ToString() );