ComplexCenteredFft
Computes the centered Fast Fourier Transform of an image.
Access to parameter description
For an introduction: see section Frequency Domain.
This algorithm is used to visualize the modulus of the FFT centered in the middle of image rather than in the upper left corner. The image is centered by a translation in circular mode by a vector equal to half the diagonal of the image. In the following figure, to visualize the image, it is divided in four equal quadrants.
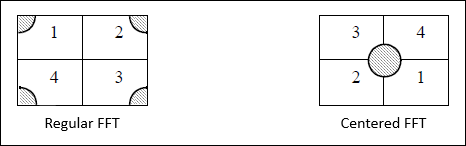
Figure 1. Results of a regular and centered FFT
See also
Access to parameter description
For an introduction: see section Frequency Domain.
This algorithm is used to visualize the modulus of the FFT centered in the middle of image rather than in the upper left corner. The image is centered by a translation in circular mode by a vector equal to half the diagonal of the image. In the following figure, to visualize the image, it is divided in four equal quadrants.
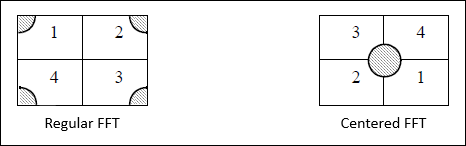
Figure 1. Results of a regular and centered FFT
See also
Function Syntax
This function returns a ComplexCenteredFftOutput structure containing the outputRealImage and outputImaginaryImage output parameters.
// Output structure. struct ComplexCenteredFftOutput { std::shared_ptr< iolink::ImageView > outputRealImage; std::shared_ptr< iolink::ImageView > outputImaginaryImage; }; // Function prototype. ComplexCenteredFftOutput complexCenteredFft( std::shared_ptr< iolink::ImageView > inputImage, std::shared_ptr< iolink::ImageView > outputRealImage = NULL, std::shared_ptr< iolink::ImageView > outputImaginaryImage = NULL );
This function returns a tuple containing the output_real_image and output_imaginary_image output parameters.
// Function prototype. complex_centered_fft( input_image, output_real_image = None, output_imaginary_image = None )
This function returns a ComplexCenteredFftOutput structure containing the outputRealImage and outputImaginaryImage output parameters.
/// Output structure of the ComplexCenteredFft function. public struct ComplexCenteredFftOutput { public IOLink.ImageView outputRealImage; public IOLink.ImageView outputImaginaryImage; }; // Function prototype. public static ComplexCenteredFftOutput ComplexCenteredFft( IOLink.ImageView inputImage, IOLink.ImageView outputRealImage = null, IOLink.ImageView outputImaginaryImage = null );
Class Syntax
Parameters
Class Name | ComplexCenteredFft |
---|
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | nullptr |
![]() |
outputRealImage |
The output real part image. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. | Image | nullptr | |
![]() |
outputImaginaryImage |
The output imaginary part image. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. | Image | nullptr |
Object Examples
auto polystyrene_float = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_float.vip" ); ComplexCenteredFft complexCenteredFftAlgo; complexCenteredFftAlgo.setInputImage( polystyrene_float ); complexCenteredFftAlgo.execute(); std::cout << "outputRealImage:" << complexCenteredFftAlgo.outputRealImage()->toString(); std::cout << "outputImaginaryImage:" << complexCenteredFftAlgo.outputImaginaryImage()->toString();
polystyrene_float = imagedev.read_vip_image(imagedev_data.get_image_path("polystyrene_float.vip")) complex_centered_fft_algo = imagedev.ComplexCenteredFft() complex_centered_fft_algo.input_image = polystyrene_float complex_centered_fft_algo.execute() print( "output_real_image:", str( complex_centered_fft_algo.output_real_image ) ); print( "output_imaginary_image:", str( complex_centered_fft_algo.output_imaginary_image ) );
ImageView polystyrene_float = Data.ReadVipImage( @"Data/images/polystyrene_float.vip" ); ComplexCenteredFft complexCenteredFftAlgo = new ComplexCenteredFft { inputImage = polystyrene_float }; complexCenteredFftAlgo.Execute(); Console.WriteLine( "outputRealImage:" + complexCenteredFftAlgo.outputRealImage.ToString() ); Console.WriteLine( "outputImaginaryImage:" + complexCenteredFftAlgo.outputImaginaryImage.ToString() );
Function Examples
auto polystyrene_float = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_float.vip" ); auto result = complexCenteredFft( polystyrene_float ); std::cout << "outputRealImage:" << result.outputRealImage->toString(); std::cout << "outputImaginaryImage:" << result.outputImaginaryImage->toString();
polystyrene_float = imagedev.read_vip_image(imagedev_data.get_image_path("polystyrene_float.vip")) result_output_real_image, result_output_imaginary_image = imagedev.complex_centered_fft( polystyrene_float ) print( "output_real_image:", str( result_output_real_image ) ); print( "output_imaginary_image:", str( result_output_imaginary_image ) );
ImageView polystyrene_float = Data.ReadVipImage( @"Data/images/polystyrene_float.vip" ); Processing.ComplexCenteredFftOutput result = Processing.ComplexCenteredFft( polystyrene_float ); Console.WriteLine( "outputRealImage:" + result.outputRealImage.ToString() ); Console.WriteLine( "outputImaginaryImage:" + result.outputImaginaryImage.ToString() );