OrientationMapFourier3d
Determines a block-wise orientation of structures contained in a three-dimensional grayscale image.
Access to parameter description
For an introduction:
The image is first divided into blocks of a user-defined size. The blocks may overlap. Then the Fourier transform is computed on each block to extract the amplitude.
The covariance matrix is computed on each Fourier transformed blocks. The matrix is symmetric, thus, the Jacobi iterative method can be used to extract the eigenvalues and the eigenvectors. $$ M= \left[ \begin{array}{ccc}; M_{2x} & M_{2xy} & M_{2xz}\\ M_{2xy} & M_{2y} & M_{2yz}\\ M_{2xz} & M_{2yz} & M_{2z}\end{array}\right] $$ The main structure orientation is given by the eigenvector associated with the lowest eigenvalue. The confidence $C$ of the estimated main orientation is calculated as follows: $$ C=\frac{\lambda_1+\lambda_2-\lambda_3}{\lambda_1+\lambda_2+\lambda_3}$$ with $\lambda_1$ being the first eigenvalue (i.e., the maximum of eigenvalues), $\lambda_2$ being the second and $\lambda_3$ the third (the minimum of eigenvalues).
With this formula, the confidence is between 0.33 and 1, then, a normalization adapts the range between 0 and 1. This algorithm does not compute local orientation in a block in two cases:
Finally, this algorithm provides two outputs:
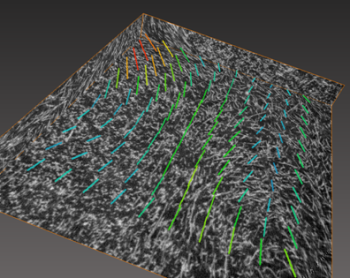
Figure 1. Local orientation map of a 3D image with a rainbow LUT (red for high confidence)
See also
Access to parameter description
For an introduction:
- section Image Analysis
- section Moment And Orientation
The image is first divided into blocks of a user-defined size. The blocks may overlap. Then the Fourier transform is computed on each block to extract the amplitude.
The covariance matrix is computed on each Fourier transformed blocks. The matrix is symmetric, thus, the Jacobi iterative method can be used to extract the eigenvalues and the eigenvectors. $$ M= \left[ \begin{array}{ccc}; M_{2x} & M_{2xy} & M_{2xz}\\ M_{2xy} & M_{2y} & M_{2yz}\\ M_{2xz} & M_{2yz} & M_{2z}\end{array}\right] $$ The main structure orientation is given by the eigenvector associated with the lowest eigenvalue. The confidence $C$ of the estimated main orientation is calculated as follows: $$ C=\frac{\lambda_1+\lambda_2-\lambda_3}{\lambda_1+\lambda_2+\lambda_3}$$ with $\lambda_1$ being the first eigenvalue (i.e., the maximum of eigenvalues), $\lambda_2$ being the second and $\lambda_3$ the third (the minimum of eigenvalues).
With this formula, the confidence is between 0.33 and 1, then, a normalization adapts the range between 0 and 1. This algorithm does not compute local orientation in a block in two cases:
- Density of structures is lower than 5
- Center of inertia is too far from the center of the block (for instance, the block is on a border of the object).
Finally, this algorithm provides two outputs:
- An image that, for each block, shows the detected structure orientation with an intensity equal to the confidence multiplied by 100
- A measurement object that, for each block, provides all computed features (orientation, confidence, moments, eigenvalues, ...)
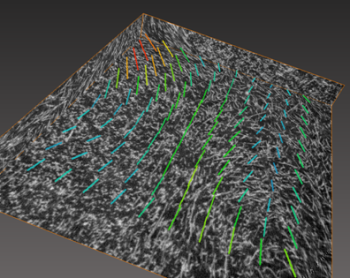
Figure 1. Local orientation map of a 3D image with a rainbow LUT (red for high confidence)
See also
Function Syntax
This function returns a OrientationMapFourier3dOutput structure containing the outputImage and outputMeasurement output parameters.
// Output structure. struct OrientationMapFourier3dOutput { std::shared_ptr< iolink::ImageView > outputImage; LocalOrientation3dMsr::Ptr outputMeasurement; }; // Function prototype. OrientationMapFourier3dOutput orientationMapFourier3d( std::shared_ptr< iolink::ImageView > inputImage, int32_t blockSize, OrientationMapFourier3d::BlockOverlap blockOverlap, int32_t minThreshold, int32_t maxThreshold, std::shared_ptr< iolink::ImageView > outputImage = NULL, LocalOrientation3dMsr::Ptr outputMeasurement = NULL );
This function returns a tuple containing the output_image and output_measurement output parameters.
// Function prototype. orientation_map_fourier_3d( input_image, block_size = 64, block_overlap = OrientationMapFourier3d.BlockOverlap.YES, min_threshold = 100, max_threshold = 200, output_image = None, output_measurement = None )
This function returns a OrientationMapFourier3dOutput structure containing the outputImage and outputMeasurement output parameters.
/// Output structure of the OrientationMapFourier3d function. public struct OrientationMapFourier3dOutput { public IOLink.ImageView outputImage; public LocalOrientation3dMsr outputMeasurement; }; // Function prototype. public static OrientationMapFourier3dOutput OrientationMapFourier3d( IOLink.ImageView inputImage, Int32 blockSize = 64, OrientationMapFourier3d.BlockOverlap blockOverlap = ImageDev.OrientationMapFourier3d.BlockOverlap.YES, Int32 minThreshold = 100, Int32 maxThreshold = 200, IOLink.ImageView outputImage = null, LocalOrientation3dMsr outputMeasurement = null );
Class Syntax
Parameters
Class Name | OrientationMapFourier3d |
---|
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The grayscale input image. | Image | Grayscale | nullptr | ||||
![]() |
blockSize |
The side size in pixels of the cubic blocks. | Int32 | >=1 | 64 | ||||
![]() |
blockOverlap |
The policy for splitting the image into blocks.
|
Enumeration | YES | |||||
![]() |
minThreshold |
The minimum threshold value applied to the input grayscale image. Voxels having an intensity lower than this threshold are not considered by the algorithm. | Int32 | >=1 | 100 | ||||
![]() |
maxThreshold |
The maximum threshold value applied to the input grayscale image. Voxels having an intensity greater than this threshold are not considered by the algorithm. | Int32 | >=1 | 200 | ||||
![]() |
outputImage |
The grayscale output image representing the oriented sticks computed in each block. Intensities correspond to the confidence of the estimated orientation multplied by 100. Image dimensions are forced to the same values as the input. Its type is unsigned 8-bit integer. | Image | nullptr | |||||
![]() |
outputMeasurement |
The output measurement result for each block. | LocalOrientation3dMsr | nullptr |
Object Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); OrientationMapFourier3d orientationMapFourier3dAlgo; orientationMapFourier3dAlgo.setInputImage( foam ); orientationMapFourier3dAlgo.setBlockSize( 10 ); orientationMapFourier3dAlgo.setBlockOverlap( OrientationMapFourier3d::BlockOverlap::YES ); orientationMapFourier3dAlgo.setMinThreshold( 100 ); orientationMapFourier3dAlgo.setMaxThreshold( 200 ); orientationMapFourier3dAlgo.execute(); std::cout << "outputImage:" << orientationMapFourier3dAlgo.outputImage()->toString(); std::cout << "blockOriginX: " << orientationMapFourier3dAlgo.outputMeasurement()->blockOriginX( 0 ) ;
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) orientation_map_fourier_3d_algo = imagedev.OrientationMapFourier3d() orientation_map_fourier_3d_algo.input_image = foam orientation_map_fourier_3d_algo.block_size = 10 orientation_map_fourier_3d_algo.block_overlap = imagedev.OrientationMapFourier3d.YES orientation_map_fourier_3d_algo.min_threshold = 100 orientation_map_fourier_3d_algo.max_threshold = 200 orientation_map_fourier_3d_algo.execute() print( "output_image:", str( orientation_map_fourier_3d_algo.output_image ) ); print( print("blockOriginX: ", orientation_map_fourier_3d_algo.output_measurement.block_origin_x( 0 ) ) );
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); OrientationMapFourier3d orientationMapFourier3dAlgo = new OrientationMapFourier3d { inputImage = foam, blockSize = 10, blockOverlap = OrientationMapFourier3d.BlockOverlap.YES, minThreshold = 100, maxThreshold = 200 }; orientationMapFourier3dAlgo.Execute(); Console.WriteLine( "outputImage:" + orientationMapFourier3dAlgo.outputImage.ToString() ); Console.WriteLine( "blockOriginX: " + orientationMapFourier3dAlgo.outputMeasurement.blockOriginX( 0 ) );
Function Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto result = orientationMapFourier3d( foam, 10, OrientationMapFourier3d::BlockOverlap::YES, 100, 200 ); std::cout << "outputImage:" << result.outputImage->toString(); std::cout << "blockOriginX: " << result.outputMeasurement->blockOriginX( 0 ) ;
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) result_output_image, result_output_measurement = imagedev.orientation_map_fourier_3d( foam, 10, imagedev.OrientationMapFourier3d.YES, 100, 200 ) print( "output_image:", str( result_output_image ) ); print( "blockOriginX: ", result_output_measurement.block_origin_x( 0 ) );
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); Processing.OrientationMapFourier3dOutput result = Processing.OrientationMapFourier3d( foam, 10, OrientationMapFourier3d.BlockOverlap.YES, 100, 200 ); Console.WriteLine( "outputImage:" + result.outputImage.ToString() ); Console.WriteLine( "blockOriginX: " + result.outputMeasurement.blockOriginX( 0 ) );