SwapQuadrants
Performs a quadrant-wise translation in circular mode.
Access to parameter description
This algorithm performs a quadrant-wise translation in circular mode by a vector equal to half the diagonal of the image, as shown in Figure 1. In case of an odd image size, the center is rounded up or down according to the centerPolicy value.
It is generally used to visualize the modulus of the FFT centered in the middle of image rather than in the upper left corner. The image is centered by a translation in circular mode by a vector equal to half the diagonal of the image.
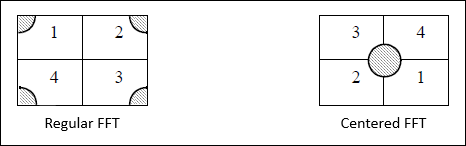
Figure 1. The results of a regular and a centered FFT
See also
Access to parameter description
This algorithm performs a quadrant-wise translation in circular mode by a vector equal to half the diagonal of the image, as shown in Figure 1. In case of an odd image size, the center is rounded up or down according to the centerPolicy value.
It is generally used to visualize the modulus of the FFT centered in the middle of image rather than in the upper left corner. The image is centered by a translation in circular mode by a vector equal to half the diagonal of the image.
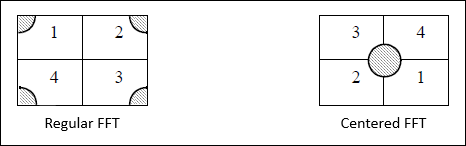
Figure 1. The results of a regular and a centered FFT
See also
Function Syntax
This function returns the outputImage output parameter.
// Function prototype. std::shared_ptr< iolink::ImageView > swapQuadrants( std::shared_ptr< iolink::ImageView > inputImage, SwapQuadrants::CenterPolicy centerPolicy, std::shared_ptr< iolink::ImageView > outputImage = NULL );
Class Syntax
Parameters
Class Name | SwapQuadrants |
---|
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | nullptr | ||||
![]() |
centerPolicy |
The way to round the center position in case of odd image dimensions.
|
Enumeration | CEIL | |||||
![]() |
outputImage |
The output image. Its dimensions and type are forced to the same values as the input. | Image | nullptr |
Object Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); SwapQuadrants swapQuadrantsAlgo; swapQuadrantsAlgo.setInputImage( foam ); swapQuadrantsAlgo.setCenterPolicy( SwapQuadrants::CenterPolicy::CEIL ); swapQuadrantsAlgo.execute(); std::cout << "outputImage:" << swapQuadrantsAlgo.outputImage()->toString();
Function Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto result = swapQuadrants( foam, SwapQuadrants::CenterPolicy::CEIL ); std::cout << "outputImage:" << result->toString();