DobFilter3d
Appproximates a Laplacian as the difference of two box filters applied on the same three-dimensional image.
Access to parameter description
For an introduction:
DobFilter3d calculates the difference between two local averages computed over square neighborhoods of different kernel sizes (Difference Of Boxes) applied on the same image. The operation consists of the same filter performed with two different kernel sizes.
The size of the kernels must be an odd number.
This operation applied to a 1D step edge is shown in Figure 1.
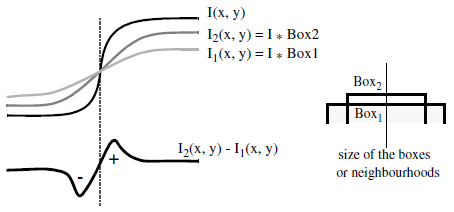
Figure 1. DOB approximation
DobFilter3d does not compute the Laplacian of an image, but is a good approximation and has the main advantage of not generating isolated points.
Typical ratios of the neighborhood sizes (width of Box1 / width of Box2) have a range between 1.5 and 2.5. Box1 and Box2 are respectively parametrized by the parameters largeBoxSize and smallBoxSize.
For example, kernels of size 5x5x5/3x3x3 or 9x9x9/5x5x5 can be used.
To keep a correct representation of the edges and a maximum of detail, using too large a kernel is not recommended (3, 5 and 7 are the most frequent values).
The deviation between the two masks has a direct effect on the edges. If the difference is too large, the edges are very thick and all small details disappear. If the difference is small (less than 4), the edges are very thin and details more precise.
See also
Access to parameter description
For an introduction:
- section Edge Detection
- section Laplacian
- section Images Filters
DobFilter3d calculates the difference between two local averages computed over square neighborhoods of different kernel sizes (Difference Of Boxes) applied on the same image. The operation consists of the same filter performed with two different kernel sizes.
The size of the kernels must be an odd number.
This operation applied to a 1D step edge is shown in Figure 1.
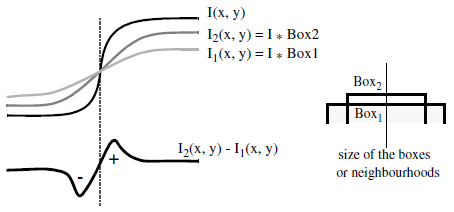
Figure 1. DOB approximation
DobFilter3d does not compute the Laplacian of an image, but is a good approximation and has the main advantage of not generating isolated points.
Typical ratios of the neighborhood sizes (width of Box1 / width of Box2) have a range between 1.5 and 2.5. Box1 and Box2 are respectively parametrized by the parameters largeBoxSize and smallBoxSize.
For example, kernels of size 5x5x5/3x3x3 or 9x9x9/5x5x5 can be used.
To keep a correct representation of the edges and a maximum of detail, using too large a kernel is not recommended (3, 5 and 7 are the most frequent values).
The deviation between the two masks has a direct effect on the edges. If the difference is too large, the edges are very thick and all small details disappear. If the difference is small (less than 4), the edges are very thin and details more precise.
See also
Function Syntax
This function returns the outputImage output parameter.
// Function prototype. std::shared_ptr< iolink::ImageView > dobFilter3d( std::shared_ptr< iolink::ImageView > inputImage, int32_t smallBoxSize, int32_t largeBoxSize, std::shared_ptr< iolink::ImageView > outputImage = NULL );
This function returns the outputImage output parameter.
// Function prototype. dob_filter_3d( input_image, small_box_size = 3, large_box_size = 7, output_image = None )
This function returns the outputImage output parameter.
// Function prototype. public static IOLink.ImageView DobFilter3d( IOLink.ImageView inputImage, Int32 smallBoxSize = 3, Int32 largeBoxSize = 7, IOLink.ImageView outputImage = null );
Class Syntax
Parameters
Class Name | DobFilter3d |
---|
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | nullptr |
![]() |
smallBoxSize |
The size of the small box. | Int32 | >=1 | 3 |
![]() |
largeBoxSize |
The size of the large box. | Int32 | >=1 | 7 |
![]() |
outputImage |
The output image. | Image | nullptr |
Object Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); DobFilter3d dobFilter3dAlgo; dobFilter3dAlgo.setInputImage( foam ); dobFilter3dAlgo.setSmallBoxSize( 3 ); dobFilter3dAlgo.setLargeBoxSize( 7 ); dobFilter3dAlgo.execute(); std::cout << "outputImage:" << dobFilter3dAlgo.outputImage()->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) dob_filter_3d_algo = imagedev.DobFilter3d() dob_filter_3d_algo.input_image = foam dob_filter_3d_algo.small_box_size = 3 dob_filter_3d_algo.large_box_size = 7 dob_filter_3d_algo.execute() print( "output_image:", str( dob_filter_3d_algo.output_image ) );
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); DobFilter3d dobFilter3dAlgo = new DobFilter3d { inputImage = foam, smallBoxSize = 3, largeBoxSize = 7 }; dobFilter3dAlgo.Execute(); Console.WriteLine( "outputImage:" + dobFilter3dAlgo.outputImage.ToString() );
Function Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto result = dobFilter3d( foam, 3, 7 ); std::cout << "outputImage:" << result->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) result = imagedev.dob_filter_3d( foam, 3, 7 ) print( "output_image:", str( result ) );
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); IOLink.ImageView result = Processing.DobFilter3d( foam, 3, 7 ); Console.WriteLine( "outputImage:" + result.ToString() );