FillHoles2d
Fills the holes inside particles of a two-dimensional binary image.
Access to parameter description
For an introduction:
Holes inside objects are filled using a numerical reconstruction applied on the complement of the input image, from markers made of pixels of the image frame.
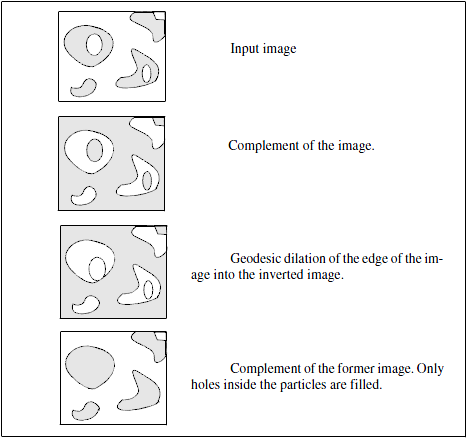
Figure 1. Illustration of the hole filling algorithm
Note: Since the reconstruction is applied on the input image complement, selecting a 4-neighbor connectivity fills holes connected to the background with a 8-neighbor connectivity.
See also
Access to parameter description
For an introduction:
- section Mathematical Morphology
- section Reconstruction From Markers
Holes inside objects are filled using a numerical reconstruction applied on the complement of the input image, from markers made of pixels of the image frame.
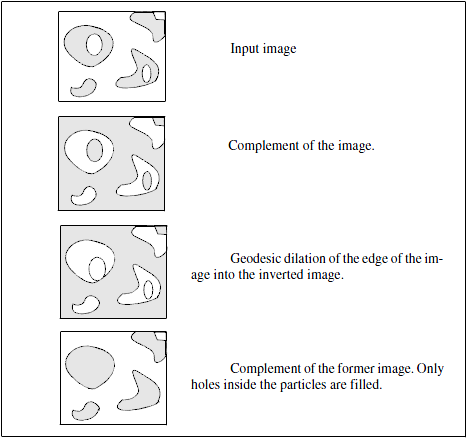
Figure 1. Illustration of the hole filling algorithm
Note: Since the reconstruction is applied on the input image complement, selecting a 4-neighbor connectivity fills holes connected to the background with a 8-neighbor connectivity.
See also
Function Syntax
This function returns the outputObjectImage output parameter.
// Function prototype. std::shared_ptr< iolink::ImageView > fillHoles2d( std::shared_ptr< iolink::ImageView > inputObjectImage, FillHoles2d::Neighborhood neighborhood, std::shared_ptr< iolink::ImageView > outputObjectImage = NULL );
Class Syntax
Parameters
Class Name | FillHoles2d |
---|
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputObjectImage |
The input binary or label image. | Image | Binary or Label | nullptr | ||||
![]() |
neighborhood |
The 2D neighborhood configuration for performing numerical reconstruction.
|
Enumeration | CONNECTIVITY_8 | |||||
![]() |
outputObjectImage |
The output binary or label image. Its dimensions and type are forced to the same values as the input image. | Image | nullptr |
Object Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); FillHoles2d fillHoles2dAlgo; fillHoles2dAlgo.setInputObjectImage( polystyrene_sep ); fillHoles2dAlgo.setNeighborhood( FillHoles2d::Neighborhood::CONNECTIVITY_8 ); fillHoles2dAlgo.execute(); std::cout << "outputObjectImage:" << fillHoles2dAlgo.outputObjectImage()->toString();
Function Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); auto result = fillHoles2d( polystyrene_sep, FillHoles2d::Neighborhood::CONNECTIVITY_8 ); std::cout << "outputObjectImage:" << result->toString();