NagaoFilter2d
Performs an edge-preserving smoothing of a two-dimensional image by selecting a mean value from different neighborhood configurations.
Access to parameter description
For an introduction to image filters: see Images Filtering.
This algorithm uses an adaptive 5x5 neighborhood geometry to compute a mean neighbors value around a central pixel.
The mean values of different neighborhood configurations around the considered pixel are computed. The pixel is replaced by the mean value of the configuration presenting:
Four neighborhood geometry types are supported by this filter:
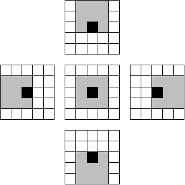
Figure 1. The Tsuji-Tomita neighborhoods.
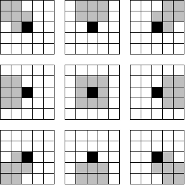
Figure 2. The Nagao 7-pixel neighborhoods.
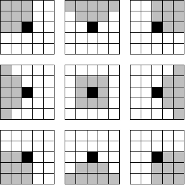
Figure 3. The Nagao 9-pixel neighborhoods.
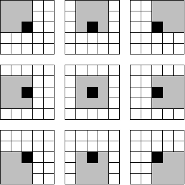
Figure 4. The Nagmod neighborhoods.
Reference:
M.Nagao, T.Matsuyama. "Edge preserving smoothing". Computer Graphics and Image Processing, vol. 9, pp. 394-407, 1979.
See also
Access to parameter description
For an introduction to image filters: see Images Filtering.
This algorithm uses an adaptive 5x5 neighborhood geometry to compute a mean neighbors value around a central pixel.
The mean values of different neighborhood configurations around the considered pixel are computed. The pixel is replaced by the mean value of the configuration presenting:
- either the minimum variance,
- or the minimum range (difference between maximum and minimum values)
Four neighborhood geometry types are supported by this filter:
- The Tsuji-Tomita neighborhoods: a set of five 3x3 sub neighborhoods among the 5x5 main neighborhoods.
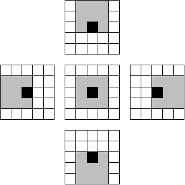
Figure 1. The Tsuji-Tomita neighborhoods.
- The 7-pixel Nagao neighborhood: a set of nine 7-pixel sub neighborhoods among the 5x5 main neighborhoods.
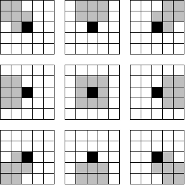
Figure 2. The Nagao 7-pixel neighborhoods.
- The 9-pixel Nagao neighborhood: a set of nine 9-pixel sub neighborhoods among the 5x5 main neighborhoods.
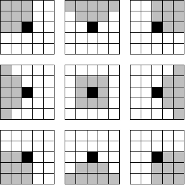
Figure 3. The Nagao 9-pixel neighborhoods.
- The Nagmod neighborhood: a set of nine 3x3 sub neighborhoods among the 5x5 main neighborhoods.
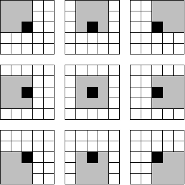
Figure 4. The Nagmod neighborhoods.
Reference:
M.Nagao, T.Matsuyama. "Edge preserving smoothing". Computer Graphics and Image Processing, vol. 9, pp. 394-407, 1979.
See also
Function Syntax
This function returns the outputImage output parameter.
// Function prototype. std::shared_ptr< iolink::ImageView > nagaoFilter2d( std::shared_ptr< iolink::ImageView > inputImage, NagaoFilter2d::FilterType filterType, NagaoFilter2d::FilterMethod filterMethod, std::shared_ptr< iolink::ImageView > outputImage = NULL );
This function returns the outputImage output parameter.
// Function prototype. nagao_filter_2d( input_image, filter_type = NagaoFilter2d.FilterType.TSUJI, filter_method = NagaoFilter2d.FilterMethod.VARIANCE, output_image = None )
This function returns the outputImage output parameter.
// Function prototype. public static IOLink.ImageView NagaoFilter2d( IOLink.ImageView inputImage, NagaoFilter2d.FilterType filterType = ImageDev.NagaoFilter2d.FilterType.TSUJI, NagaoFilter2d.FilterMethod filterMethod = ImageDev.NagaoFilter2d.FilterMethod.VARIANCE, IOLink.ImageView outputImage = null );
Class Syntax
Parameters
Class Name | NagaoFilter2d |
---|
Parameter Name | Description | Type | Supported Values | Default Value | |||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | nullptr | ||||||||
![]() |
filterType |
The neighborhood geometry type.
|
Enumeration | TSUJI | |||||||||
![]() |
filterMethod |
The neighborhood selection criterion.
|
Enumeration | VARIANCE | |||||||||
![]() |
outputImage |
The output image. Its dimensions, type, and calibration are forced to the same values as the input. | Image | nullptr |
Object Examples
std::shared_ptr< iolink::ImageView > polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); NagaoFilter2d nagaoFilter2dAlgo; nagaoFilter2dAlgo.setInputImage( polystyrene ); nagaoFilter2dAlgo.setFilterType( NagaoFilter2d::FilterType::TSUJI ); nagaoFilter2dAlgo.setFilterMethod( NagaoFilter2d::FilterMethod::VARIANCE ); nagaoFilter2dAlgo.execute(); std::cout << "outputImage:" << nagaoFilter2dAlgo.outputImage()->toString();
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) nagao_filter_2d_algo = imagedev.NagaoFilter2d() nagao_filter_2d_algo.input_image = polystyrene nagao_filter_2d_algo.filter_type = imagedev.NagaoFilter2d.TSUJI nagao_filter_2d_algo.filter_method = imagedev.NagaoFilter2d.VARIANCE nagao_filter_2d_algo.execute() print( "output_image:", str( nagao_filter_2d_algo.output_image ) );
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); NagaoFilter2d nagaoFilter2dAlgo = new NagaoFilter2d { inputImage = polystyrene, filterType = NagaoFilter2d.FilterType.TSUJI, filterMethod = NagaoFilter2d.FilterMethod.VARIANCE }; nagaoFilter2dAlgo.Execute(); Console.WriteLine( "outputImage:" + nagaoFilter2dAlgo.outputImage.ToString() );
Function Examples
std::shared_ptr< iolink::ImageView > polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); auto result = nagaoFilter2d( polystyrene, NagaoFilter2d::FilterType::TSUJI, NagaoFilter2d::FilterMethod::VARIANCE ); std::cout << "outputImage:" << result->toString();
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) result = imagedev.nagao_filter_2d( polystyrene, imagedev.NagaoFilter2d.TSUJI, imagedev.NagaoFilter2d.VARIANCE ) print( "output_image:", str( result ) );
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); IOLink.ImageView result = Processing.NagaoFilter2d( polystyrene, NagaoFilter2d.FilterType.TSUJI, NagaoFilter2d.FilterMethod.VARIANCE ); Console.WriteLine( "outputImage:" + result.ToString() );