Create 2D Image
This example illustrates how to create a data buffer corresponding to a 2D image, and copy it in an object connectable to an ImageDev algorithm.
To invoke an ImageDev algorithm, the data to process needs to be previously imported into an ImageView object
of the IOLink library.
The first part of this example simply creates a buffer representing a 768x1027 pixel image with a filled square drawn inside. This step is for demonstration purposes only. In practice, you should already have the content of the image to process in a buffer stored in the data model used by your application.
Then some IOLink instructions create a new ImageView object. The buffer previously created is copied in this image with the WriteRegion method.
Two ImageDev algorithms are applied afterward to show that it is now possible to process this image with ImageDev.
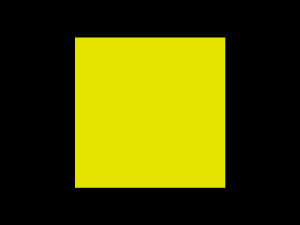
Figure 1. The 2D image generated by this example
See also
The first part of this example simply creates a buffer representing a 768x1027 pixel image with a filled square drawn inside. This step is for demonstration purposes only. In practice, you should already have the content of the image to process in a buffer stored in the data model used by your application.
Then some IOLink instructions create a new ImageView object. The buffer previously created is copied in this image with the WriteRegion method.
Two ImageDev algorithms are applied afterward to show that it is now possible to process this image with ImageDev.
- ResetImage reinitializes all pixel intensities to 0 and generates a new empty image.
- GrayscaleToColor combines the generated images in the 3 channels of an RGB image. The image representing the square is set in the red and green channels, the empty image is set into the blue channel. The result image is a color image representing a yellow square.
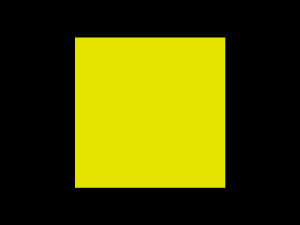
Figure 1. The 2D image generated by this example
#include <ImageDev/ImageDev.h> #include <ioformat/IOFormat.h> #include <iolink/view/ImageViewFactory.h> #include <iolink/view/ImageViewProvider.h> #include <string.h> using namespace imagedev; using namespace ioformat; using namespace iolink; int main( int argc, char* argv[] ) { int status = 0; try { // ImageDev library initialization if ( imagedev::isInitialized() == false ) imagedev::init(); // Initialize an unsigned 8-bit array storing data of a 2D image const uint64_t rowCount = 768; const uint64_t colCount = 1024; std::vector< uint8_t > imageData( rowCount * colCount ); // Define a synthetic square in this array const uint64_t side = colCount / 2; // side in pixels of the square to draw // Loop on image rows for ( uint64_t i = 0; i < rowCount; ++i ) { // Loop on image columns for ( uint64_t j = 0; j < colCount; ++j ) { if ( ( i >= ( rowCount - side ) / 2 ) && ( i <= ( rowCount + side ) / 2 ) && ( j >= ( colCount - side ) / 2 ) && ( j <= ( colCount + side ) / 2 ) ) imageData[i * colCount + j] = 228; // Value inside the square else imageData[i * colCount + j] = 0; // Background value } } // Create an image view of same dimensions VectorXu64 imageShape{ colCount, rowCount }; auto image = ImageViewFactory::allocate( imageShape, DataTypeId::UINT8 ); image->setAxesInterpretation( ImageTypeId::IMAGE ); // Define the region where to write the data VectorXu64 imageOrig{ 0, 0 }; RegionXu64 imageRegion( imageOrig, imageShape ); // Copy the data in the image view image->writeRegion( imageRegion, imageData.data() ); // This image can now be processed by any ImageDev algorithm, for instance for building a color image auto imageVoid = resetImage( image, 0.0f ); auto imageRgb = grayscaleToColor( image, image, imageVoid ); // Save the created image with IOFormat writeView( imageRgb, "T02_01_output.png" ); std::cout << "This example ran successfully." << std::endl; } catch ( const imagedev::Exception& error ) { // Print potential exception in the standard output std::cerr << "ImageDev exception: " << error.what() << std::endl; status = -1; } // ImageDev library finalization imagedev::finish(); // Check if we must ask for an enter key to close the program if ( !( ( argc == 2 ) && strcmp( argv[1], "--no-stop-at-end" ) == 0 ) ) std::cout << "Press Enter key to close this window." << std::endl, getchar(); return status; }
using System; using ImageDev; using IOLink; using IOFormat; namespace T02_01_CreateImage2D { class Program { static void Main(string[] args) { int status = 0; try { // Initialize the ImageDev library if not done if (Initialization.IsInitialized() == false) Initialization.Init(); // Initialize an unsigned 8-bit array storing data of a 2D image ulong rowCount = 768; ulong colCount = 1024; byte[] imageData = new byte[rowCount * colCount]; // Define a synthetic square in this array ulong side = colCount / 2; // side in pixels of the square to draw // Loop on image rows for (ulong i = 0; i < rowCount; ++i) { // Loop on image columns for (ulong j = 0; j < colCount; ++j) { if ((i >= (rowCount - side) / 2) && (i <= (rowCount + side) / 2) && (j >= (colCount - side) / 2) && (j <= (colCount + side) / 2)) imageData[i * colCount + j] = 228; // Value inside the square else imageData[i * colCount + j] = 0; // Background value } } // Create an image view of same dimensions VectorXu64 imageShape = new VectorXu64(colCount, rowCount); ImageView image = ImageViewFactory.Allocate(imageShape, DataTypeId.UINT8); image.AxesInterpretation = ImageTypeId.IMAGE; // Define the region where to write the data VectorXu64 imageOrig = new VectorXu64(0, 0); RegionXu64 imageRegion = new RegionXu64(imageOrig, imageShape); // Copy the data in the image view image.WriteRegion(imageRegion, imageData); // This image can now be processed by any ImageDev algorithm, for instance for building a color image ImageView imageVoid = Processing.ResetImage(image, 0.0f); ImageView imageRgb = Processing.GrayscaleToColor(image, image, imageVoid); // Save the created image with IOFormat ViewIO.WriteView(imageRgb, "T02_01_output.png"); } catch (Exception error) { // Print potential exception in the standard output System.Console.WriteLine("HelloImageDev exception: " + error.ToString()); status = -1; } // ImageDev library finalization Initialization.Finish(); // Check if we must ask for an enter key to close the program if (!((args.Length >= 1) && (args[0] == "--no-stop-at-end"))) { System.Console.WriteLine("Press Enter key to close this window."); System.Console.ReadKey(); } System.Environment.Exit(status); } } }
import imagedev import iolink import ioformat import numpy # Initialize an unsigned 8 - bit array storing data of a 3D image row_count = 768 col_count = 1024 image_data = numpy.zeros((row_count , col_count), numpy.uint8) # Define a synthetic square in this array side = int(col_count / 2) # side in pixels of the square to draw image_data[int((row_count - side) / 2):int((row_count + side) / 2), int((col_count - side) / 2):int((col_count + side) / 2)] = 228 # Create an image view of same dimensions VectorXu64 image_shape = iolink.VectorXu64(col_count, row_count) image = iolink.ImageViewFactory.allocate(image_shape, iolink.DataTypeId_UINT8) image.axes_interpretation = iolink.ImageTypeId.IMAGE # Define the region where to write the data image_orig = iolink.VectorXu64(0, 0) image_region = iolink.RegionXu64(image_orig, image_shape) # Copy the data in the image view image.write_region(image_region, image_data) # This image can now be processed by any ImageDev algorithm, for instance for building a color image image_void = imagedev.reset_image(image, 0.0) image_rgb = imagedev.grayscale_to_color(image, image, image_void) # Save the created image with IOFormat ioformat.write_view(image_rgb, "T02_01_output.png")
See also