LocalMaxima3d
Extracts the local maxima of an image using a three-dimensional neighborhood analysis.
Access to parameter description
This algorithm considers I(i,j,k) as a local maximum of I if the two conditions below are verified
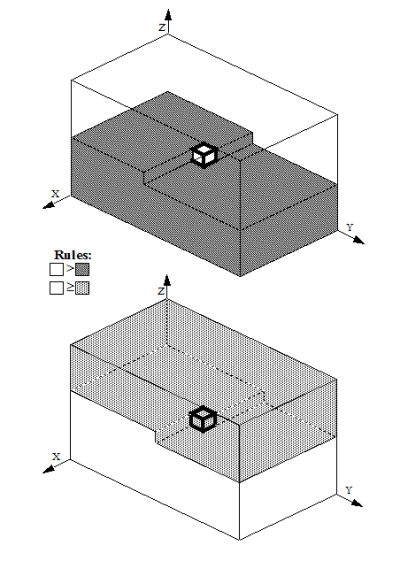
Figure 1. Graphic visualization of the 3D neighborhood analysis
See also
Access to parameter description
This algorithm considers I(i,j,k) as a local maximum of I if the two conditions below are verified
-
1. I(i,j,k) is a local maximum in a (2p+1)×(2q+1)\times(2r+1)neighborhood,i.e.,consideringl \in [1,p],m \in [1,q],n \in [1,r],sin[−p,p]andtin[−q,q],I(i,j)$
- I(i,j,k)>I(i+s,j+t,k−n)
- I(i,j,k)>I(i+s,j−m,k)
- I(i,j,k)>I(i−l,j,k)
- I(i,j,k)≥I(i+s,j,k)
- I(i,j,k)≥I(i+s,j+t,k+n)
- I(i,j,k)>threshold
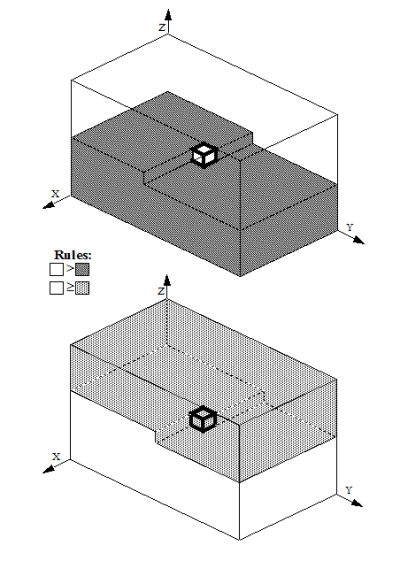
Figure 1. Graphic visualization of the 3D neighborhood analysis
See also
Function Syntax
This function returns outputMeasurement.
// Function prototype
LocalMaximaMsr::Ptr localMaxima3d( std::shared_ptr< iolink::ImageView > inputGrayImage, iolink::Vector3i32 localWindow, double thresholdRange, LocalMaxima3d::Precision precision, int32_t numberOfPatterns, LocalMaximaMsr::Ptr outputMeasurement = nullptr );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputGrayImage |
The input grayscale image | Image | Binary, Label, Grayscale or Multispectral | nullptr | ||||
![]() |
localWindow |
The neighborhood size in pixels, along the X, Y, and Z directions. | Vector3i32 | >=0 | {3, 3, 3} | ||||
![]() |
thresholdRange |
The threshold value higher than which maxima are retained. | Float64 | >=0 | 0 | ||||
![]() |
precision |
The precision of the maxima localization.
|
Enumeration | PIXEL | |||||
![]() |
numberOfPatterns |
The maximum number of patterns to be detected. | Int32 | >=1 | 1 | ||||
![]() |
outputMeasurement |
The result object containing the maxima positions. | LocalMaximaMsr | nullptr |
Object Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); LocalMaxima3d localMaxima3dAlgo; localMaxima3dAlgo.setInputGrayImage( foam ); localMaxima3dAlgo.setLocalWindow( {3, 3, 3} ); localMaxima3dAlgo.setThresholdRange( 0 ); localMaxima3dAlgo.setPrecision( LocalMaxima3d::Precision::PIXEL ); localMaxima3dAlgo.setNumberOfPatterns( 1 ); localMaxima3dAlgo.execute(); std::cout << "value: " << localMaxima3dAlgo.outputMeasurement()->value( ) ;
Function Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto result = localMaxima3d( foam, {3, 3, 3}, 0, LocalMaxima3d::Precision::PIXEL, 1 ); std::cout << "value: " << result->value( ) ;