RegionalExtrema2d
Computes the regional maxima or minima of a two-dimensional grayscale image and marks them in a binary image.
Access to parameter description
For an introduction:
A regional maximum (resp. minimum) C is a set of connected pixels such that:
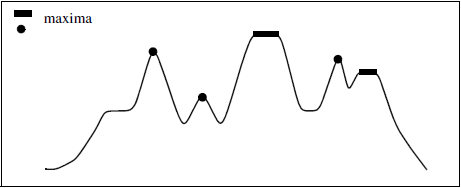
Figure 1. One-dimensional example of a regional maxima detection
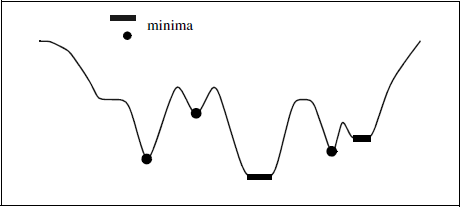
Figure 2. One-dimensional example of a regional minima detection
This algorithm uses a recursive method combined with a geodesic propagation.
To avoid getting too many regions in the output image, the input should be smoothed first with a low-pass filter or with the numerical reconstruction algorithm.
Figure 3. Original image (left) and regional maxima in red (right)
Reference:
P. Soille, Morphological Image Analysis. Principles and Applications, Second Edition, Springer-Verlag, Berlin, pp.201-203, 2003.
See also
Access to parameter description
For an introduction:
- section Mathematical Morphology
- section Geodesic Transformations
A regional maximum (resp. minimum) C is a set of connected pixels such that:
- Pixels belonging to C have the same intensity IC.
- Pixels connected to C, but not belonging to C (neighbors), have an intensity strictly lower (resp. greater) than IC.
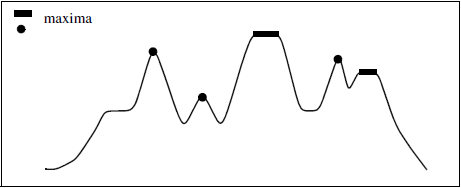
Figure 1. One-dimensional example of a regional maxima detection
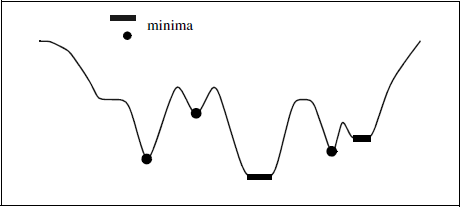
Figure 2. One-dimensional example of a regional minima detection
This algorithm uses a recursive method combined with a geodesic propagation.
To avoid getting too many regions in the output image, the input should be smoothed first with a low-pass filter or with the numerical reconstruction algorithm.
![]() |
![]() |
Reference:
P. Soille, Morphological Image Analysis. Principles and Applications, Second Edition, Springer-Verlag, Berlin, pp.201-203, 2003.
See also
Function Syntax
This function returns outputBinaryImage.
// Function prototype
std::shared_ptr< iolink::ImageView > regionalExtrema2d( std::shared_ptr< iolink::ImageView > inputImage, RegionalExtrema2d::ExtremaType extremaType, RegionalExtrema2d::Neighborhood neighborhood, std::shared_ptr< iolink::ImageView > outputBinaryImage = nullptr );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input grayscale image. | Image | Grayscale | nullptr | ||||
![]() |
extremaType |
The type of extrema to detect.
|
Enumeration | MAXIMA | |||||
![]() |
neighborhood |
The 2D neighborhood configuration used for geodesic propagation.
|
Enumeration | CONNECTIVITY_8 | |||||
![]() |
outputBinaryImage |
The output binary image. Its dimensions are forced to the same values as the input. | Image | nullptr |
Object Examples
auto polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); RegionalExtrema2d regionalExtrema2dAlgo; regionalExtrema2dAlgo.setInputImage( polystyrene ); regionalExtrema2dAlgo.setExtremaType( RegionalExtrema2d::ExtremaType::MAXIMA ); regionalExtrema2dAlgo.setNeighborhood( RegionalExtrema2d::Neighborhood::CONNECTIVITY_8 ); regionalExtrema2dAlgo.execute(); std::cout << "outputBinaryImage:" << regionalExtrema2dAlgo.outputBinaryImage()->toString();
Function Examples
auto polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); auto result = regionalExtrema2d( polystyrene, RegionalExtrema2d::ExtremaType::MAXIMA, RegionalExtrema2d::Neighborhood::CONNECTIVITY_8 ); std::cout << "outputBinaryImage:" << result->toString();