ImageCurvature2d
Computes local gray level curvature in a two-dimensional image.
Access to parameter description
This algorithm computes, for each pixel of an input image, a local texture curvature attribute. The curvature estimation takes the pixel size into account.
The curvature is extracted from the gradient structure tensor of the input image. For each pixel, the curvature parameter $\kappa$ is linked to the radius of curvature $R$ by the formula :
$$ \kappa = \frac{1}{R} $$
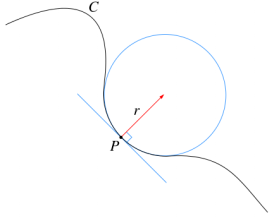
Figure 1. The Local radius of curvature
Reference:
L.J. van Vliet, P.W. Verbeek. "Curvature and Bending Energy in Digitized 2D and 3D Images". SCIA 93, Proc. 8th Scandinavian Conference on Image Analysis, Tromso, Norway, pp. 1403-1410, 1993.
See also
Access to parameter description
This algorithm computes, for each pixel of an input image, a local texture curvature attribute. The curvature estimation takes the pixel size into account.
The curvature is extracted from the gradient structure tensor of the input image. For each pixel, the curvature parameter $\kappa$ is linked to the radius of curvature $R$ by the formula :
$$ \kappa = \frac{1}{R} $$
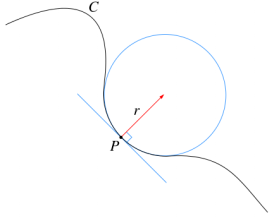
Figure 1. The Local radius of curvature
Reference:
L.J. van Vliet, P.W. Verbeek. "Curvature and Bending Energy in Digitized 2D and 3D Images". SCIA 93, Proc. 8th Scandinavian Conference on Image Analysis, Tromso, Norway, pp. 1403-1410, 1993.
See also
Function Syntax
This function returns outputRealImage.
// Function prototype
std::shared_ptr< iolink::ImageView > imageCurvature2d( std::shared_ptr< iolink::ImageView > inputImage, std::shared_ptr< iolink::ImageView > inputMaskImage, double standardDeviation, std::shared_ptr< iolink::ImageView > outputRealImage = nullptr );
This function returns outputRealImage.
// Function prototype. image_curvature_2d(input_image: idt.ImageType, input_mask_image: idt.ImageType, standard_deviation: float = 4, output_real_image: idt.ImageType = None) -> idt.ImageType
This function returns outputRealImage.
// Function prototype. public static IOLink.ImageView ImageCurvature2d( IOLink.ImageView inputImage, IOLink.ImageView inputMaskImage, double standardDeviation = 4, IOLink.ImageView outputRealImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | nullptr |
![]() |
inputMaskImage |
The binary image designating the area to process. If it equals null, the algorithm is applied on the whole image. This image must have the same dimensions as the input image. | Image | Binary | nullptr |
![]() |
standardDeviation |
The standard deviation for Gaussian filter, expressed in world coordinates of the input image. | Float64 | >0 | 4 |
![]() |
outputRealImage |
The output image. Each pixel value represents the inverse of the local curvature radius in the world coordinates system. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
input_image |
The input image. | image | Binary, Label, Grayscale or Multispectral | None |
![]() |
input_mask_image |
The binary image designating the area to process. If it equals null, the algorithm is applied on the whole image. This image must have the same dimensions as the input image. | image | Binary | None |
![]() |
standard_deviation |
The standard deviation for Gaussian filter, expressed in world coordinates of the input image. | float64 | >0 | 4 |
![]() |
output_real_image |
The output image. Each pixel value represents the inverse of the local curvature radius in the world coordinates system. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | null |
![]() |
inputMaskImage |
The binary image designating the area to process. If it equals null, the algorithm is applied on the whole image. This image must have the same dimensions as the input image. | Image | Binary | null |
![]() |
standardDeviation |
The standard deviation for Gaussian filter, expressed in world coordinates of the input image. | Float64 | >0 | 4 |
![]() |
outputRealImage |
The output image. Each pixel value represents the inverse of the local curvature radius in the world coordinates system. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. | Image | null |
Object Examples
auto polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); ImageCurvature2d imageCurvature2dAlgo; imageCurvature2dAlgo.setInputImage( polystyrene ); imageCurvature2dAlgo.setInputMaskImage( polystyrene_sep ); imageCurvature2dAlgo.setStandardDeviation( 4.0 ); imageCurvature2dAlgo.execute(); std::cout << "outputRealImage:" << imageCurvature2dAlgo.outputRealImage()->toString();
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) polystyrene_sep = imagedev.read_vip_image(imagedev_data.get_image_path("polystyrene_sep.vip")) image_curvature_2d_algo = imagedev.ImageCurvature2d() image_curvature_2d_algo.input_image = polystyrene image_curvature_2d_algo.input_mask_image = polystyrene_sep image_curvature_2d_algo.standard_deviation = 4.0 image_curvature_2d_algo.execute() print("output_real_image:", str(image_curvature_2d_algo.output_real_image))
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); ImageView polystyrene_sep = Data.ReadVipImage( @"Data/images/polystyrene_sep.vip" ); ImageCurvature2d imageCurvature2dAlgo = new ImageCurvature2d { inputImage = polystyrene, inputMaskImage = polystyrene_sep, standardDeviation = 4.0 }; imageCurvature2dAlgo.Execute(); Console.WriteLine( "outputRealImage:" + imageCurvature2dAlgo.outputRealImage.ToString() );
Function Examples
auto polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); auto result = imageCurvature2d( polystyrene, polystyrene_sep, 4.0 ); std::cout << "outputRealImage:" << result->toString();
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) polystyrene_sep = imagedev.read_vip_image(imagedev_data.get_image_path("polystyrene_sep.vip")) result = imagedev.image_curvature_2d(polystyrene, polystyrene_sep, 4.0) print("output_real_image:", str(result))
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); ImageView polystyrene_sep = Data.ReadVipImage( @"Data/images/polystyrene_sep.vip" ); IOLink.ImageView result = Processing.ImageCurvature2d( polystyrene, polystyrene_sep, 4.0 ); Console.WriteLine( "outputRealImage:" + result.ToString() );