ImageCurvature3d
Computes local gray level curvature in a two-dimensional image.
Access to parameter description
This algorithm computes, for each voxel of an input image, two local texture curvature attributes. The curvature estimation takes the voxel size into account.
The curvature is extracted from the gradient structure tensor of the input image.
Each voxel is then associated with two principle curvatures : $\kappa_{Max}$ and $\kappa_{Min}$.
The $\kappa_{Max}$ stands for maximum local curvature, whereas $\kappa_{Min}$ stands for minimum curvature.
Two important indicators can be extracted from these values:
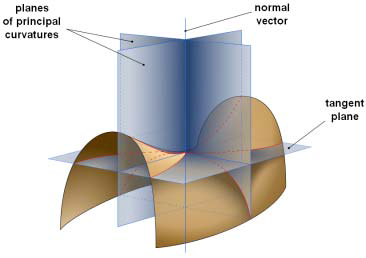
Figure 1. Saddle-shaped 3D curvature (image: Eric Gaba)
References:
See also
Access to parameter description
This algorithm computes, for each voxel of an input image, two local texture curvature attributes. The curvature estimation takes the voxel size into account.
The curvature is extracted from the gradient structure tensor of the input image.
Each voxel is then associated with two principle curvatures : $\kappa_{Max}$ and $\kappa_{Min}$.
The $\kappa_{Max}$ stands for maximum local curvature, whereas $\kappa_{Min}$ stands for minimum curvature.
Two important indicators can be extracted from these values:
- Gaussian curvature : $K=\kappa_{Max} \times \kappa_{Min}$
- Mean curvature : $H=\frac{1}{2}(\kappa_{Max} + \kappa_{Min})$
- If Gaussian curvature is positive, both principle curvatures have same sign and local surface is dome-like (which means that it is locally lying on one side of its tangent plane). In this case, a positive curvature stands for a convex surface, whereas a negative value stands for a concave surface.
- If Gaussian curvature is negative, principle curvatures have opposite signs and the local surface is saddle-shaped (see figure 1).
- If Gaussian curvature is null, the surface is locally parabolic. If $\kappa_{Max}=0$, surface profile is locally valley-like. If $\kappa_{Min}=0$, surface profile is locally crest-like.
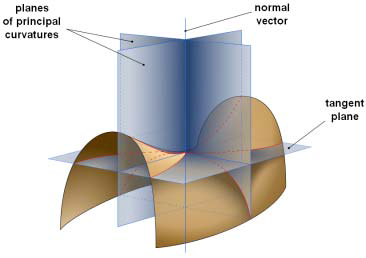
Figure 1. Saddle-shaped 3D curvature (image: Eric Gaba)
References:
- O. Monga, S. Benayou. "Using Partial Derivatives of 3D Images to Extract Typical Surface Features". Computer Vision and Image Understanding, CVIU. 61, pp. 225-236, 1995.
- B. Rieger, F.J. Timmermans, L.J. van Vliet, P.W. Verbeek. "On Curvature Estimation of ISO Surfaces in 3D Gray-Value Images and the Computation of Shape Descriptors". IEEE TRANSACTIONS ON PATTERN ANALYSIS AND MACHINE INTELLIGENCE, vol.26, no. 8, pp. 1088-1094, Aug. 2004.
See also
Function Syntax
This function returns a ImageCurvature3dOutput structure containing outputMaximumImage and outputMinimumImage.
// Output structure of the imageCurvature3d function. struct ImageCurvature3dOutput { /// The maximum curvature output image. Each pixel value represents the maximum curvature in the world coordinates system. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. std::shared_ptr< iolink::ImageView > outputMaximumImage; /// The minimum curvature output image. Each pixel value represents the minimum curvature in the world coordinates system. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. std::shared_ptr< iolink::ImageView > outputMinimumImage; }; // Function prototype
ImageCurvature3dOutput imageCurvature3d( std::shared_ptr< iolink::ImageView > inputImage, std::shared_ptr< iolink::ImageView > inputMaskImage, double standardDeviation, std::shared_ptr< iolink::ImageView > outputMaximumImage = nullptr, std::shared_ptr< iolink::ImageView > outputMinimumImage = nullptr );
This function returns a tuple containing output_maximum_image and output_minimum_image.
// Function prototype. image_curvature_3d(input_image: idt.ImageType, input_mask_image: idt.ImageType, standard_deviation: float = 4, output_maximum_image: idt.ImageType = None, output_minimum_image: idt.ImageType = None) -> Tuple[idt.ImageType, idt.ImageType]
This function returns a ImageCurvature3dOutput structure containing outputMaximumImage and outputMinimumImage.
/// Output structure of the ImageCurvature3d function. public struct ImageCurvature3dOutput { /// /// The maximum curvature output image. Each pixel value represents the maximum curvature in the world coordinates system. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. /// public IOLink.ImageView outputMaximumImage; /// /// The minimum curvature output image. Each pixel value represents the minimum curvature in the world coordinates system. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. /// public IOLink.ImageView outputMinimumImage; }; // Function prototype. public static ImageCurvature3dOutput ImageCurvature3d( IOLink.ImageView inputImage, IOLink.ImageView inputMaskImage, double standardDeviation = 4, IOLink.ImageView outputMaximumImage = null, IOLink.ImageView outputMinimumImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | nullptr |
![]() |
inputMaskImage |
The binary image designating the area to process. If it equals null, the algorithm is applied on the whole image. This image must have same dimensions as the input image. | Image | Binary | nullptr |
![]() |
standardDeviation |
The standard deviation for Gaussian filter, expressed in world coordinates of the input image. | Float64 | >0 | 4 |
![]() |
outputMaximumImage |
The maximum curvature output image. Each pixel value represents the maximum curvature in the world coordinates system. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. | Image | nullptr | |
![]() |
outputMinimumImage |
The minimum curvature output image. Each pixel value represents the minimum curvature in the world coordinates system. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
input_image |
The input image. | image | Binary, Label, Grayscale or Multispectral | None |
![]() |
input_mask_image |
The binary image designating the area to process. If it equals null, the algorithm is applied on the whole image. This image must have same dimensions as the input image. | image | Binary | None |
![]() |
standard_deviation |
The standard deviation for Gaussian filter, expressed in world coordinates of the input image. | float64 | >0 | 4 |
![]() |
output_maximum_image |
The maximum curvature output image. Each pixel value represents the maximum curvature in the world coordinates system. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. | image | None | |
![]() |
output_minimum_image |
The minimum curvature output image. Each pixel value represents the minimum curvature in the world coordinates system. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | null |
![]() |
inputMaskImage |
The binary image designating the area to process. If it equals null, the algorithm is applied on the whole image. This image must have same dimensions as the input image. | Image | Binary | null |
![]() |
standardDeviation |
The standard deviation for Gaussian filter, expressed in world coordinates of the input image. | Float64 | >0 | 4 |
![]() |
outputMaximumImage |
The maximum curvature output image. Each pixel value represents the maximum curvature in the world coordinates system. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. | Image | null | |
![]() |
outputMinimumImage |
The minimum curvature output image. Each pixel value represents the minimum curvature in the world coordinates system. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. | Image | null |
Object Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto foam_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam_sep.vip" ); ImageCurvature3d imageCurvature3dAlgo; imageCurvature3dAlgo.setInputImage( foam ); imageCurvature3dAlgo.setInputMaskImage( foam_sep ); imageCurvature3dAlgo.setStandardDeviation( 4.0 ); imageCurvature3dAlgo.execute(); std::cout << "outputMaximumImage:" << imageCurvature3dAlgo.outputMaximumImage()->toString(); std::cout << "outputMinimumImage:" << imageCurvature3dAlgo.outputMinimumImage()->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) foam_sep = imagedev.read_vip_image(imagedev_data.get_image_path("foam_sep.vip")) image_curvature_3d_algo = imagedev.ImageCurvature3d() image_curvature_3d_algo.input_image = foam image_curvature_3d_algo.input_mask_image = foam_sep image_curvature_3d_algo.standard_deviation = 4.0 image_curvature_3d_algo.execute() print("output_maximum_image:", str(image_curvature_3d_algo.output_maximum_image)) print("output_minimum_image:", str(image_curvature_3d_algo.output_minimum_image))
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); ImageView foam_sep = Data.ReadVipImage( @"Data/images/foam_sep.vip" ); ImageCurvature3d imageCurvature3dAlgo = new ImageCurvature3d { inputImage = foam, inputMaskImage = foam_sep, standardDeviation = 4.0 }; imageCurvature3dAlgo.Execute(); Console.WriteLine( "outputMaximumImage:" + imageCurvature3dAlgo.outputMaximumImage.ToString() ); Console.WriteLine( "outputMinimumImage:" + imageCurvature3dAlgo.outputMinimumImage.ToString() );
Function Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto foam_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam_sep.vip" ); auto result = imageCurvature3d( foam, foam_sep, 4.0 ); std::cout << "outputMaximumImage:" << result.outputMaximumImage->toString(); std::cout << "outputMinimumImage:" << result.outputMinimumImage->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) foam_sep = imagedev.read_vip_image(imagedev_data.get_image_path("foam_sep.vip")) result_output_maximum_image, result_output_minimum_image = imagedev.image_curvature_3d(foam, foam_sep, 4.0) print("output_maximum_image:", str(result_output_maximum_image)) print("output_minimum_image:", str(result_output_minimum_image))
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); ImageView foam_sep = Data.ReadVipImage( @"Data/images/foam_sep.vip" ); Processing.ImageCurvature3dOutput result = Processing.ImageCurvature3d( foam, foam_sep, 4.0 ); Console.WriteLine( "outputMaximumImage:" + result.outputMaximumImage.ToString() ); Console.WriteLine( "outputMinimumImage:" + result.outputMinimumImage.ToString() );