Thresholding
Transforms a grayscale image into a binary image where all pixels with an initial gray level value lying between two user-defined bounds are set to 1 and all others are set to 0.
Access to parameter description
For an introduction:
Threshold with 2 bounds is the default behavior, but a threshold with a single bound may be achieved by setting λ1 or λ2 to the minimum or maximum value of the input data range (for example, 0 or 255, respectively for 8-bit unsigned data).
The definition is: O(n,m)={1if λ1≤I(n,m)≤λ20if I(n,m) <λ1 or I(n,m)>λ2
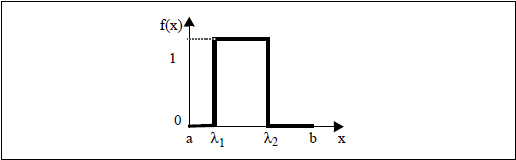
Figure 1. Thresholding a grayscale image
Note: This algorithm is dedicated to be applied on grayscale images. However, for convenience, it does not return an exception when applied on a color image. It simply processes the last color channel; for instance, the blue channel in the case of an RGB image.
See also
See related example
Access to parameter description
For an introduction:
- section Image Segmentation
- section Binarization
Threshold with 2 bounds is the default behavior, but a threshold with a single bound may be achieved by setting λ1 or λ2 to the minimum or maximum value of the input data range (for example, 0 or 255, respectively for 8-bit unsigned data).
The definition is: O(n,m)={1if λ1≤I(n,m)≤λ20if I(n,m) <λ1 or I(n,m)>λ2
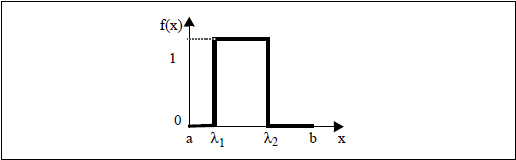
Figure 1. Thresholding a grayscale image
Note: This algorithm is dedicated to be applied on grayscale images. However, for convenience, it does not return an exception when applied on a color image. It simply processes the last color channel; for instance, the blue channel in the case of an RGB image.
See also
See related example
Function Syntax
This function returns outputBinaryImage.
// Function prototype
std::shared_ptr< iolink::ImageView > thresholding( std::shared_ptr< iolink::ImageView > inputImage, iolink::Vector2d thresholdRange, std::shared_ptr< iolink::ImageView > outputBinaryImage = nullptr );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | nullptr |
![]() |
thresholdRange |
The low and high threshold levels. | Vector2d | Any value | {128.f, 255.f} |
![]() |
outputBinaryImage |
The output binary image. Its dimensions are forced to the same values as the input. | Image | nullptr |
Object Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); Thresholding thresholdingAlgo; thresholdingAlgo.setInputImage( foam ); thresholdingAlgo.setThresholdRange( {128.0, 255.0} ); thresholdingAlgo.execute(); std::cout << "outputBinaryImage:" << thresholdingAlgo.outputBinaryImage()->toString();
Function Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto result = thresholding( foam, {128.0, 255.0} ); std::cout << "outputBinaryImage:" << result->toString();