ErosionLine3d
Performs a three-dimensional erosion using a structuring element matching with a line.
Access to parameter description
For an introduction:
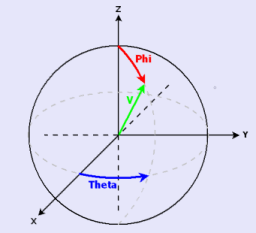
Figure 1. Azimuthal and polar angles on the unit sphere
See also
Access to parameter description
For an introduction:
- section Mathematical Morphology
- section Introduction To Erosion
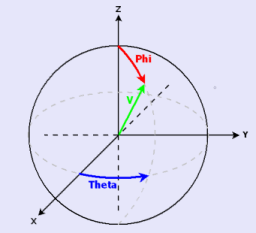
Figure 1. Azimuthal and polar angles on the unit sphere
See also
Function Syntax
This function returns outputImage.
// Function prototype
std::shared_ptr< iolink::ImageView > erosionLine3d( std::shared_ptr< iolink::ImageView > inputImage, double thetaAngle, double phiAngle, uint32_t kernelRadius, std::shared_ptr< iolink::ImageView > outputImage = nullptr );
This function returns outputImage.
// Function prototype. erosion_line_3d(input_image: idt.ImageType, theta_angle: float = 0, phi_angle: float = 0, kernel_radius: int = 3, output_image: idt.ImageType = None) -> idt.ImageType
This function returns outputImage.
// Function prototype. public static IOLink.ImageView ErosionLine3d( IOLink.ImageView inputImage, double thetaAngle = 0, double phiAngle = 0, UInt32 kernelRadius = 3, IOLink.ImageView outputImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputImage |
The input image. The image type can be integer or float. | Image | Binary, Label, Grayscale or Multispectral | nullptr |
![]() |
thetaAngle |
The azimuthal angle in degrees. | Float64 | Any value | 0 |
![]() |
phiAngle |
The polar angle in degrees. | Float64 | Any value | 0 |
![]() |
kernelRadius |
The length of the linear structuring element in pixels. | UInt32 | >=1 | 3 |
![]() |
outputImage |
The output image. Its dimensions and type are forced to the same values as the input image. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
input_image |
The input image. The image type can be integer or float. | image | Binary, Label, Grayscale or Multispectral | None |
![]() |
theta_angle |
The azimuthal angle in degrees. | float64 | Any value | 0 |
![]() |
phi_angle |
The polar angle in degrees. | float64 | Any value | 0 |
![]() |
kernel_radius |
The length of the linear structuring element in pixels. | uint32 | >=1 | 3 |
![]() |
output_image |
The output image. Its dimensions and type are forced to the same values as the input image. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputImage |
The input image. The image type can be integer or float. | Image | Binary, Label, Grayscale or Multispectral | null |
![]() |
thetaAngle |
The azimuthal angle in degrees. | Float64 | Any value | 0 |
![]() |
phiAngle |
The polar angle in degrees. | Float64 | Any value | 0 |
![]() |
kernelRadius |
The length of the linear structuring element in pixels. | UInt32 | >=1 | 3 |
![]() |
outputImage |
The output image. Its dimensions and type are forced to the same values as the input image. | Image | null |
Object Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); ErosionLine3d erosionLine3dAlgo; erosionLine3dAlgo.setInputImage( foam ); erosionLine3dAlgo.setThetaAngle( 0 ); erosionLine3dAlgo.setPhiAngle( 0 ); erosionLine3dAlgo.setKernelRadius( 3 ); erosionLine3dAlgo.execute(); std::cout << "outputImage:" << erosionLine3dAlgo.outputImage()->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) erosion_line_3d_algo = imagedev.ErosionLine3d() erosion_line_3d_algo.input_image = foam erosion_line_3d_algo.theta_angle = 0 erosion_line_3d_algo.phi_angle = 0 erosion_line_3d_algo.kernel_radius = 3 erosion_line_3d_algo.execute() print("output_image:", str(erosion_line_3d_algo.output_image))
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); ErosionLine3d erosionLine3dAlgo = new ErosionLine3d { inputImage = foam, thetaAngle = 0, phiAngle = 0, kernelRadius = 3 }; erosionLine3dAlgo.Execute(); Console.WriteLine( "outputImage:" + erosionLine3dAlgo.outputImage.ToString() );
Function Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto result = erosionLine3d( foam, 0, 0, 3 ); std::cout << "outputImage:" << result->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) result = imagedev.erosion_line_3d(foam, 0, 0, 3) print("output_image:", str(result))
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); IOLink.ImageView result = Processing.ErosionLine3d( foam, 0, 0, 3 ); Console.WriteLine( "outputImage:" + result.ToString() );