DilationLine2d
Performs a two-dimensional dilation using a structuring element matching with a line.
Access to parameter description
For an introduction:
The dilation is performed by using a linear structuring element. The direction of 0 degrees is horizontal and to the left, and the angles are calculated counter-clockwise.
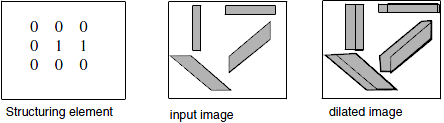
Figure 1. Linear structuring element and dilated image
See also
Access to parameter description
For an introduction:
- section Mathematical Morphology
- section Introduction To Dilation
The dilation is performed by using a linear structuring element. The direction of 0 degrees is horizontal and to the left, and the angles are calculated counter-clockwise.
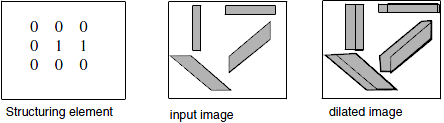
Figure 1. Linear structuring element and dilated image
See also
Function Syntax
This function returns outputImage.
// Function prototype
std::shared_ptr< iolink::ImageView > dilationLine2d( std::shared_ptr< iolink::ImageView > inputImage, double orientationAngle, uint32_t kernelRadius, std::shared_ptr< iolink::ImageView > outputImage = nullptr );
This function returns outputImage.
// Function prototype. dilation_line_2d(input_image: idt.ImageType, orientation_angle: float = 10, kernel_radius: int = 3, output_image: idt.ImageType = None) -> idt.ImageType
This function returns outputImage.
// Function prototype. public static IOLink.ImageView DilationLine2d( IOLink.ImageView inputImage, double orientationAngle = 10, UInt32 kernelRadius = 3, IOLink.ImageView outputImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputImage |
The input image. The image type can be integer or float. | Image | Binary, Label, Grayscale or Multispectral | nullptr |
![]() |
orientationAngle |
The angle of orientation in degrees. | Float64 | Any value | 10 |
![]() |
kernelRadius |
The length of the linear structuring element in pixels. | UInt32 | >=1 | 3 |
![]() |
outputImage |
The output image. Its dimensions and type are forced to the same values as the input image. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
input_image |
The input image. The image type can be integer or float. | image | Binary, Label, Grayscale or Multispectral | None |
![]() |
orientation_angle |
The angle of orientation in degrees. | float64 | Any value | 10 |
![]() |
kernel_radius |
The length of the linear structuring element in pixels. | uint32 | >=1 | 3 |
![]() |
output_image |
The output image. Its dimensions and type are forced to the same values as the input image. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputImage |
The input image. The image type can be integer or float. | Image | Binary, Label, Grayscale or Multispectral | null |
![]() |
orientationAngle |
The angle of orientation in degrees. | Float64 | Any value | 10 |
![]() |
kernelRadius |
The length of the linear structuring element in pixels. | UInt32 | >=1 | 3 |
![]() |
outputImage |
The output image. Its dimensions and type are forced to the same values as the input image. | Image | null |
Object Examples
auto polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); DilationLine2d dilationLine2dAlgo; dilationLine2dAlgo.setInputImage( polystyrene ); dilationLine2dAlgo.setOrientationAngle( 10 ); dilationLine2dAlgo.setKernelRadius( 3 ); dilationLine2dAlgo.execute(); std::cout << "outputImage:" << dilationLine2dAlgo.outputImage()->toString();
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) dilation_line_2d_algo = imagedev.DilationLine2d() dilation_line_2d_algo.input_image = polystyrene dilation_line_2d_algo.orientation_angle = 10 dilation_line_2d_algo.kernel_radius = 3 dilation_line_2d_algo.execute() print("output_image:", str(dilation_line_2d_algo.output_image))
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); DilationLine2d dilationLine2dAlgo = new DilationLine2d { inputImage = polystyrene, orientationAngle = 10, kernelRadius = 3 }; dilationLine2dAlgo.Execute(); Console.WriteLine( "outputImage:" + dilationLine2dAlgo.outputImage.ToString() );
Function Examples
auto polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); auto result = dilationLine2d( polystyrene, 10, 3 ); std::cout << "outputImage:" << result->toString();
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) result = imagedev.dilation_line_2d(polystyrene, 10, 3) print("output_image:", str(result))
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); IOLink.ImageView result = Processing.DilationLine2d( polystyrene, 10, 3 ); Console.WriteLine( "outputImage:" + result.ToString() );