BinaryCorrelation2d
Performs a logical correlation between a binary two-dimensional image and a binary kernel.
Access to parameter description
For an introduction: section Image Correlation.
The binary correlation O between image I and kernel K is defined as: O(n,m)=O1(n,m)+O2(n,m) O1(n,m)=kx∑i=1ky∑j=1(K(i,j)∧I(n+i−kx2,m+j−ky2)) O2(n,m)=kx∑i=1ky∑j=1¯(K(i,j)∨I(n+i−kx2,m+j−ky2)) where:
At the end of the process, the correlation image is normalized between -1 and 1.
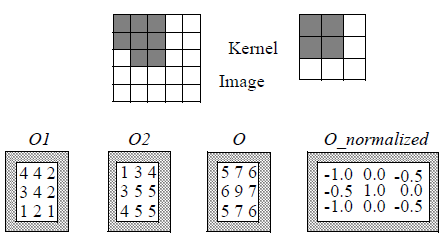
Figure 1 Example of BinaryCorrelation2d
When a part of the kernel lies beyond the edge of the image, the correlation is not computed on the border. In such cases, the values are set to -1E10.
See also
Access to parameter description
For an introduction: section Image Correlation.
The binary correlation O between image I and kernel K is defined as: O(n,m)=O1(n,m)+O2(n,m) O1(n,m)=kx∑i=1ky∑j=1(K(i,j)∧I(n+i−kx2,m+j−ky2)) O2(n,m)=kx∑i=1ky∑j=1¯(K(i,j)∨I(n+i−kx2,m+j−ky2)) where:
- A∧B⇔A AND B
- A∨B⇔A OR B
- ¯A⇔NOT A
At the end of the process, the correlation image is normalized between -1 and 1.
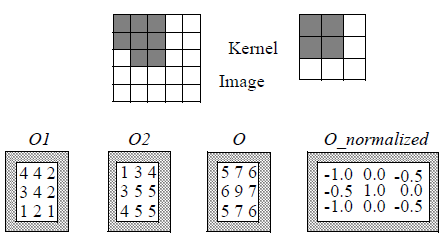
Figure 1 Example of BinaryCorrelation2d
When a part of the kernel lies beyond the edge of the image, the correlation is not computed on the border. In such cases, the values are set to -1E10.
See also
Function Syntax
This function returns a BinaryCorrelation2dOutput structure containing outputImage and outputMeasurement.
// Output structure of the binaryCorrelation2d function. struct BinaryCorrelation2dOutput { /// The output correlation image. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. std::shared_ptr< iolink::ImageView > outputImage; /// The correlation matching results. CorrelationMsr::Ptr outputMeasurement; }; // Function prototype
BinaryCorrelation2dOutput binaryCorrelation2d( std::shared_ptr< iolink::ImageView > inputBinaryImage, std::shared_ptr< iolink::ImageView > inputKernelImage, BinaryCorrelation2d::OffsetMode offsetMode, std::shared_ptr< iolink::ImageView > outputImage = nullptr, CorrelationMsr::Ptr outputMeasurement = nullptr );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
inputBinaryImage |
The input binary image. | Image | Binary | nullptr | ||||||||
![]() |
inputKernelImage |
The correlation kernel. | Image | Binary | nullptr | ||||||||
![]() |
offsetMode |
The calculation offset, in pixels. The greater this value, computation is faster but detection is less precise.
|
Enumeration | OFFSET_1 | |||||||||
![]() |
outputImage |
The output correlation image. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. | Image | nullptr | |||||||||
![]() |
outputMeasurement |
The correlation matching results. | CorrelationMsr | nullptr |
Object Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); BinaryCorrelation2d binaryCorrelation2dAlgo; binaryCorrelation2dAlgo.setInputBinaryImage( polystyrene_sep ); binaryCorrelation2dAlgo.setInputKernelImage( polystyrene_sep ); binaryCorrelation2dAlgo.setOffsetMode( BinaryCorrelation2d::OffsetMode::OFFSET_1 ); binaryCorrelation2dAlgo.execute(); std::cout << "outputImage:" << binaryCorrelation2dAlgo.outputImage()->toString(); std::cout << "minComputed: " << binaryCorrelation2dAlgo.outputMeasurement()->minComputed( 0 ) ;
Function Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); auto result = binaryCorrelation2d( polystyrene_sep, polystyrene_sep, BinaryCorrelation2d::OffsetMode::OFFSET_1 ); std::cout << "outputImage:" << result.outputImage->toString(); std::cout << "minComputed: " << result.outputMeasurement->minComputed( 0 ) ;