CudaCrossCorrelation2d
Performs a correlation by convolution between a two-dimensional grayscale image and a grayscale kernel. The calculations are performed on the GPU.
Access to parameter description
This command is experimental, his signature may be modified between now and his final version.
For an introduction: section Image Correlation.
This algorithm performs a cross-correlation, or correlation with a mutiplication metric, between a gray level image $I$ and a gray level kernel $K$, returning the correlation image $O$.
The different possibilities for selecting a correlation peak are presented below using a 1-D correlation between an image and kernel. In the image, the kernel appears 6 times with different contrast and luminosity.
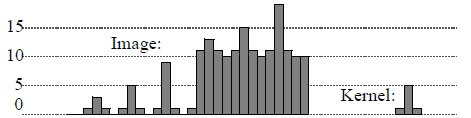
Figure 1. 1D image and kernel
The 6 examples show the kernel appearing with different contrast and luminosity.
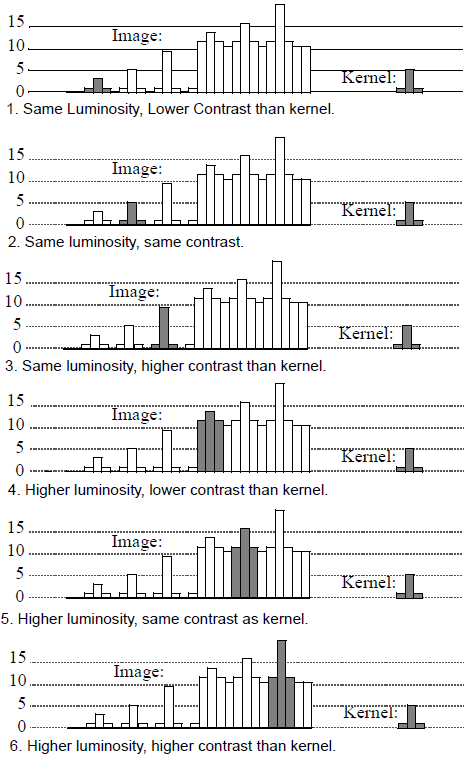
Figure 2. Examples of possible 1D correlation
The cross-correlation metric is computed in accordance with the CorrelationMode parameter.
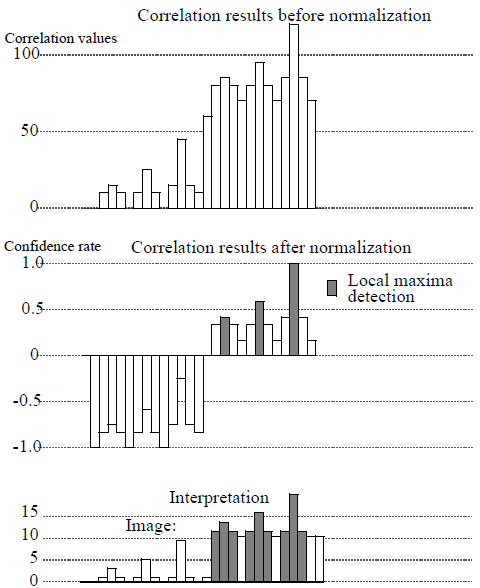
Figure 3. Example of 1D direct cross-correlation
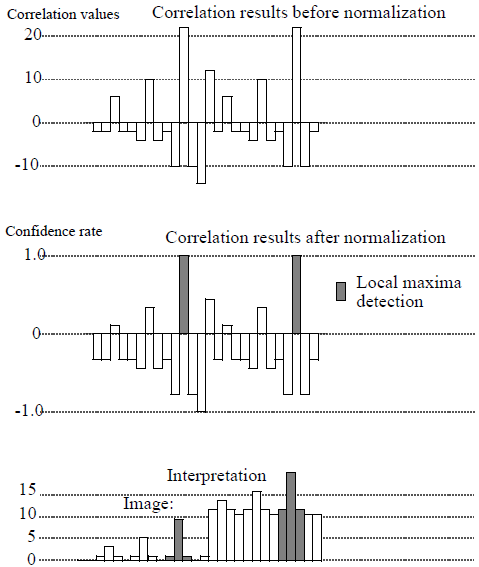
Figure 4. Example of 1D mean cross-correlation
Note: This algorithm returns the main correlation peak in the outputMeasurement object. More correlation peaks can be extracted from the outputImage correlation image with the LocalMaxima2d algorithm
See also
Access to parameter description
This command is experimental, his signature may be modified between now and his final version.
For an introduction: section Image Correlation.
This algorithm performs a cross-correlation, or correlation with a mutiplication metric, between a gray level image $I$ and a gray level kernel $K$, returning the correlation image $O$.
The different possibilities for selecting a correlation peak are presented below using a 1-D correlation between an image and kernel. In the image, the kernel appears 6 times with different contrast and luminosity.
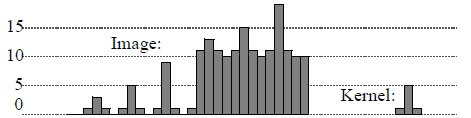
Figure 1. 1D image and kernel
The 6 examples show the kernel appearing with different contrast and luminosity.
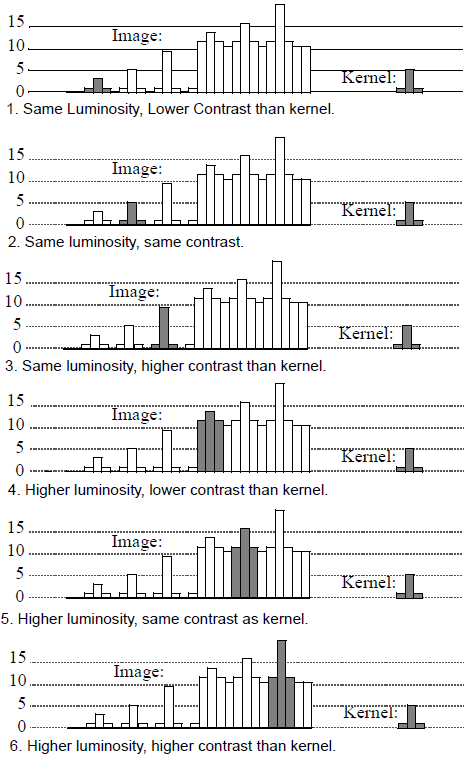
Figure 2. Examples of possible 1D correlation
The cross-correlation metric is computed in accordance with the CorrelationMode parameter.
DIRECT Correlation Mode
$$ O(n,m)=\sum_{i=1}^{kx} \sum_{j=1}^{ky} K(i,j)\times I\left(n+i-\frac{kx}{2},m+j-\frac{ky}{2}\right) $$ In this mode, 3 of the 6 patterns matching the kernel are detected. These are only high luminosity patterns. The best matching is obtained for the highest contrast and luminosity pattern.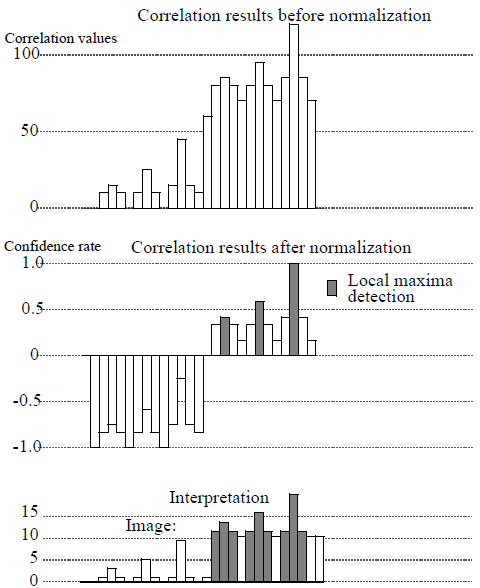
Figure 3. Example of 1D direct cross-correlation
MEAN Correlation Mode
$$ O(n,m)=\sum_{i=1}^{kx} \sum_{j=1}^{ky} \left(K(i,j)-\mu(K)\right)\times \left(I(n+i-\frac{kx}{2},m+j-\frac{ky}{2})-\mu(I)(n,m)\right) $$ In this mode, 2 of the 6 patterns matching the kernel are detected. These are only high contrast patterns. The confidence rate is the same for the two 2 patterns.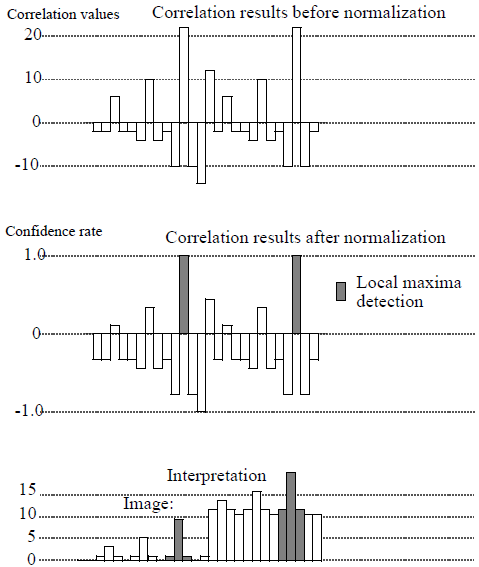
Figure 4. Example of 1D mean cross-correlation
Note: This algorithm returns the main correlation peak in the outputMeasurement object. More correlation peaks can be extracted from the outputImage correlation image with the LocalMaxima2d algorithm
See also
Function Syntax
This function returns a CudaCrossCorrelation2dOutput structure containing outputImage and outputMeasurement.
// Output structure of the cudaCrossCorrelation2d function. struct CudaCrossCorrelation2dOutput { /// The output correlation image. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. std::shared_ptr< iolink::ImageView > outputImage; /// The correlation matching results. CrossCorrelation2dMsr::Ptr outputMeasurement; }; // Function prototype
CudaCrossCorrelation2dOutput cudaCrossCorrelation2d( std::shared_ptr< iolink::ImageView > inputImage, std::shared_ptr< iolink::ImageView > inputKernelImage, CudaCrossCorrelation2d::CorrelationMode correlationMode, CudaCrossCorrelation2d::TilingMode tilingMode, iolink::Vector2u32 tileSize, CudaContext::Ptr cudaContext, std::shared_ptr< iolink::ImageView > outputImage = nullptr, CrossCorrelation2dMsr::Ptr outputMeasurement = nullptr );
This function returns a tuple containing output_image and output_measurement.
// Function prototype. cuda_cross_correlation_2d(input_image: idt.ImageType, input_kernel_image: idt.ImageType, correlation_mode: CudaCrossCorrelation2d.CorrelationMode = CudaCrossCorrelation2d.CorrelationMode.DIRECT, tiling_mode: CudaCrossCorrelation2d.TilingMode = CudaCrossCorrelation2d.TilingMode.NONE, tile_size: Iterable[int] = [1024, 1024], cuda_context: Union[CudaContext, None] = None, output_image: idt.ImageType = None, output_measurement: Union[Any, None] = None) -> Tuple[idt.ImageType, CrossCorrelation2dMsr]
This function returns a CudaCrossCorrelation2dOutput structure containing outputImage and outputMeasurement.
/// Output structure of the CudaCrossCorrelation2d function. public struct CudaCrossCorrelation2dOutput { /// /// The output correlation image. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. /// public IOLink.ImageView outputImage; /// The correlation matching results. public CrossCorrelation2dMsr outputMeasurement; }; // Function prototype. public static CudaCrossCorrelation2dOutput CudaCrossCorrelation2d( IOLink.ImageView inputImage, IOLink.ImageView inputKernelImage, CudaCrossCorrelation2d.CorrelationMode correlationMode = ImageDev.CudaCrossCorrelation2d.CorrelationMode.DIRECT, CudaCrossCorrelation2d.TilingMode tilingMode = ImageDev.CudaCrossCorrelation2d.TilingMode.NONE, uint[] tileSize = null, Data.CudaContext cudaContext = null, IOLink.ImageView outputImage = null, CrossCorrelation2dMsr outputMeasurement = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input grayscale image. | Image | Grayscale | nullptr | ||||
![]() |
inputKernelImage |
The correlation kernel. | Image | Grayscale | nullptr | ||||
![]() |
correlationMode |
The normalization mode for correlation.
|
Enumeration | DIRECT | |||||
![]() |
tilingMode |
The way to manage the GPU memory.
|
Enumeration | NONE | |||||
![]() |
tileSize |
The tile width and height in pixels. They must be greater than or equal to the correlation kernel width and height. This parameter is used only in USER_DEFINED tiling mode. | Vector2u32 | Any value | {1024, 1024} | ||||
![]() |
cudaContext |
CUDA context information. | CudaContext | nullptr | |||||
![]() |
outputImage |
The output correlation image. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. | Image | nullptr | |||||
![]() |
outputMeasurement |
The correlation matching results. | CrossCorrelation2dMsr | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
input_image |
The input grayscale image. | image | Grayscale | None | ||||
![]() |
input_kernel_image |
The correlation kernel. | image | Grayscale | None | ||||
![]() |
correlation_mode |
The normalization mode for correlation.
|
enumeration | DIRECT | |||||
![]() |
tiling_mode |
The way to manage the GPU memory.
|
enumeration | NONE | |||||
![]() |
tile_size |
The tile width and height in pixels. They must be greater than or equal to the correlation kernel width and height. This parameter is used only in USER_DEFINED tiling mode. | vector2u32 | Any value | [1024, 1024] | ||||
![]() |
cuda_context |
CUDA context information. | cuda_context | None | |||||
![]() |
output_image |
The output correlation image. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. | image | None | |||||
![]() |
output_measurement |
The correlation matching results. | CrossCorrelation2dMsr | None |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input grayscale image. | Image | Grayscale | null | ||||
![]() |
inputKernelImage |
The correlation kernel. | Image | Grayscale | null | ||||
![]() |
correlationMode |
The normalization mode for correlation.
|
Enumeration | DIRECT | |||||
![]() |
tilingMode |
The way to manage the GPU memory.
|
Enumeration | NONE | |||||
![]() |
tileSize |
The tile width and height in pixels. They must be greater than or equal to the correlation kernel width and height. This parameter is used only in USER_DEFINED tiling mode. | Vector2u32 | Any value | {1024, 1024} | ||||
![]() |
cudaContext |
CUDA context information. | CudaContext | null | |||||
![]() |
outputImage |
The output correlation image. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. | Image | null | |||||
![]() |
outputMeasurement |
The correlation matching results. | CrossCorrelation2dMsr | null |
Object Examples
auto polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); CudaCrossCorrelation2d cudaCrossCorrelation2dAlgo; cudaCrossCorrelation2dAlgo.setInputImage( polystyrene ); cudaCrossCorrelation2dAlgo.setInputKernelImage( polystyrene ); cudaCrossCorrelation2dAlgo.setCorrelationMode( CudaCrossCorrelation2d::CorrelationMode::DIRECT ); cudaCrossCorrelation2dAlgo.setTilingMode( CudaCrossCorrelation2d::TilingMode::NONE ); cudaCrossCorrelation2dAlgo.setTileSize( {264, 264} ); cudaCrossCorrelation2dAlgo.setCudaContext( nullptr ); cudaCrossCorrelation2dAlgo.setOutputImage( iolink::ImageViewFactory::allocate( iolink::VectorXu64( { 1, 1 } ), iolink::DataTypeId::UINT8 ) ); cudaCrossCorrelation2dAlgo.execute(); std::cout << "outputImage:" << cudaCrossCorrelation2dAlgo.outputImage()->toString(); std::cout << "matchingPositionX: " << cudaCrossCorrelation2dAlgo.outputMeasurement()->matchingPositionX( 0 ) ;
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) cuda_cross_correlation_2d_algo = imagedev.CudaCrossCorrelation2d() cuda_cross_correlation_2d_algo.input_image = polystyrene cuda_cross_correlation_2d_algo.input_kernel_image = polystyrene cuda_cross_correlation_2d_algo.correlation_mode = imagedev.CudaCrossCorrelation2d.DIRECT cuda_cross_correlation_2d_algo.tiling_mode = imagedev.CudaCrossCorrelation2d.NONE cuda_cross_correlation_2d_algo.tile_size = [264, 264] cuda_cross_correlation_2d_algo.cuda_context = None cuda_cross_correlation_2d_algo.execute() print("output_image:", str(cuda_cross_correlation_2d_algo.output_image)) print("matchingPositionX: ", str(cuda_cross_correlation_2d_algo.output_measurement.matching_position_x(0)))
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); CudaCrossCorrelation2d cudaCrossCorrelation2dAlgo = new CudaCrossCorrelation2d { inputImage = polystyrene, inputKernelImage = polystyrene, correlationMode = CudaCrossCorrelation2d.CorrelationMode.DIRECT, tilingMode = CudaCrossCorrelation2d.TilingMode.NONE, tileSize = new uint[]{264, 264}, cudaContext = null }; cudaCrossCorrelation2dAlgo.Execute(); Console.WriteLine( "outputImage:" + cudaCrossCorrelation2dAlgo.outputImage.ToString() ); Console.WriteLine( "matchingPositionX: " + cudaCrossCorrelation2dAlgo.outputMeasurement.matchingPositionX( 0 ) );
Function Examples
auto polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); auto result = cudaCrossCorrelation2d( polystyrene, polystyrene, CudaCrossCorrelation2d::CorrelationMode::DIRECT, CudaCrossCorrelation2d::TilingMode::NONE, {264, 264}, nullptr , iolink::ImageViewFactory::allocate( iolink::VectorXu64( { 1, 1 } ), iolink::DataTypeId::UINT8 )); std::cout << "outputImage:" << result.outputImage->toString(); std::cout << "matchingPositionX: " << result.outputMeasurement->matchingPositionX( 0 ) ;
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) result_output_image, result_output_measurement = imagedev.cuda_cross_correlation_2d(polystyrene, polystyrene, imagedev.CudaCrossCorrelation2d.DIRECT, imagedev.CudaCrossCorrelation2d.NONE, [264, 264], None) print("output_image:", str(result_output_image)) print("matchingPositionX: ", str(result_output_measurement.matching_position_x(0)))
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); Processing.CudaCrossCorrelation2dOutput result = Processing.CudaCrossCorrelation2d( polystyrene, polystyrene, CudaCrossCorrelation2d.CorrelationMode.DIRECT, CudaCrossCorrelation2d.TilingMode.NONE, new uint[]{264, 264}, null ); Console.WriteLine( "outputImage:" + result.outputImage.ToString() ); Console.WriteLine( "matchingPositionX: " + result.outputMeasurement.matchingPositionX( 0 ) );