EndPoints2d
Point detector selecting end points of a two-dimensional binary image.
Access to parameter description
For an introduction:
This algorithm is a point detector that selects end points of a skeleton (that is, all object pixels having only one neighbor).
The skeleton is first detected, and then this algorithm performs a hit-or-miss transform (HMT) with the following configuration and its 7 associated rotations: 00×010000 Where × means "don't care".
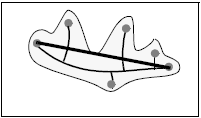
Figure 1. Skeleton and its end points
See also
Access to parameter description
For an introduction:
- section Mathematical Morphology
- section Point Detectors
This algorithm is a point detector that selects end points of a skeleton (that is, all object pixels having only one neighbor).
The skeleton is first detected, and then this algorithm performs a hit-or-miss transform (HMT) with the following configuration and its 7 associated rotations: 00×010000 Where × means "don't care".
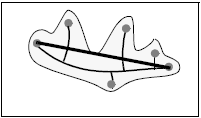
Figure 1. Skeleton and its end points
See also
Function Syntax
This function returns outputBinaryImage.
// Function prototype
std::shared_ptr< iolink::ImageView > endPoints2d( std::shared_ptr< iolink::ImageView > inputBinaryImage, EndPoints2d::BorderCondition borderCondition, EndPoints2d::Neighborhood neighborhood, std::shared_ptr< iolink::ImageView > outputBinaryImage = nullptr );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputBinaryImage |
The binary input image. | Image | Binary | nullptr | ||||
![]() |
borderCondition |
The way to consider pixels out of image borders.
|
Enumeration | ZERO | |||||
![]() |
neighborhood |
The 2D neighborhood configuration for performing dilations or erosions.
|
Enumeration | CONNECTIVITY_8 | |||||
![]() |
outputBinaryImage |
The binary output image. Its size and type are forced to the same values as the input. | Image | nullptr |
Object Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); EndPoints2d endPoints2dAlgo; endPoints2dAlgo.setInputBinaryImage( polystyrene_sep ); endPoints2dAlgo.setBorderCondition( EndPoints2d::BorderCondition::ZERO ); endPoints2dAlgo.setNeighborhood( EndPoints2d::Neighborhood::CONNECTIVITY_8 ); endPoints2dAlgo.execute(); std::cout << "outputBinaryImage:" << endPoints2dAlgo.outputBinaryImage()->toString();
Function Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); auto result = endPoints2d( polystyrene_sep, EndPoints2d::BorderCondition::ZERO, EndPoints2d::Neighborhood::CONNECTIVITY_8 ); std::cout << "outputBinaryImage:" << result->toString();