BitShift
Shifts the intensities of an image by a given number of bits.
Access to parameter description
Each intensity of the input image $I$ is shifted as shown in the example from the following figure.
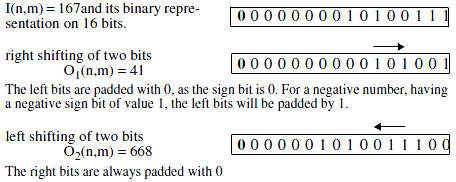
Figure 1. Shifting a 16-bit integer value by 2 bits
To summarize:
See also
Access to parameter description
Each intensity of the input image $I$ is shifted as shown in the example from the following figure.
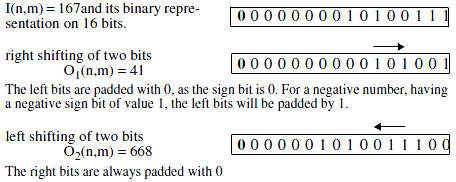
Figure 1. Shifting a 16-bit integer value by 2 bits
To summarize:
- i bits right <B>shifting</B> $ O(n,m)=I(n,m) / 2^i$
- i bits left <B>shifting</B> $ O(n,m)=I(n,m)\times 2^i$
See also
Function Syntax
This function returns outputImage.
// Function prototype
std::shared_ptr< iolink::ImageView > bitShift( std::shared_ptr< iolink::ImageView > inputImage, int32_t bitNumber, BitShift::ShiftDirection shiftDirection, std::shared_ptr< iolink::ImageView > outputImage = nullptr );
This function returns outputImage.
// Function prototype. bit_shift(input_image: idt.ImageType, bit_number: int = 1, shift_direction: BitShift.ShiftDirection = BitShift.ShiftDirection.LEFT, output_image: idt.ImageType = None) -> idt.ImageType
This function returns outputImage.
// Function prototype. public static IOLink.ImageView BitShift( IOLink.ImageView inputImage, Int32 bitNumber = 1, BitShift.ShiftDirection shiftDirection = ImageDev.BitShift.ShiftDirection.LEFT, IOLink.ImageView outputImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | nullptr | ||||
![]() |
bitNumber |
The number of bits to shift. | Int32 | >=0 | 1 | ||||
![]() |
shiftDirection |
The shifting direction.
|
Enumeration | LEFT | |||||
![]() |
outputImage |
The output image, size and type are forced to the same values as the input. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
input_image |
The input image. | image | Binary, Label, Grayscale or Multispectral | None | ||||
![]() |
bit_number |
The number of bits to shift. | int32 | >=0 | 1 | ||||
![]() |
shift_direction |
The shifting direction.
|
enumeration | LEFT | |||||
![]() |
output_image |
The output image, size and type are forced to the same values as the input. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | null | ||||
![]() |
bitNumber |
The number of bits to shift. | Int32 | >=0 | 1 | ||||
![]() |
shiftDirection |
The shifting direction.
|
Enumeration | LEFT | |||||
![]() |
outputImage |
The output image, size and type are forced to the same values as the input. | Image | null |
Object Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); BitShift bitShiftAlgo; bitShiftAlgo.setInputImage( foam ); bitShiftAlgo.setBitNumber( 1 ); bitShiftAlgo.setShiftDirection( BitShift::ShiftDirection::LEFT ); bitShiftAlgo.execute(); std::cout << "outputImage:" << bitShiftAlgo.outputImage()->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) bit_shift_algo = imagedev.BitShift() bit_shift_algo.input_image = foam bit_shift_algo.bit_number = 1 bit_shift_algo.shift_direction = imagedev.BitShift.LEFT bit_shift_algo.execute() print("output_image:", str(bit_shift_algo.output_image))
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); BitShift bitShiftAlgo = new BitShift { inputImage = foam, bitNumber = 1, shiftDirection = BitShift.ShiftDirection.LEFT }; bitShiftAlgo.Execute(); Console.WriteLine( "outputImage:" + bitShiftAlgo.outputImage.ToString() );
Function Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto result = bitShift( foam, 1, BitShift::ShiftDirection::LEFT ); std::cout << "outputImage:" << result->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) result = imagedev.bit_shift(foam, 1, imagedev.BitShift.LEFT) print("output_image:", str(result))
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); IOLink.ImageView result = Processing.BitShift( foam, 1, BitShift.ShiftDirection.LEFT ); Console.WriteLine( "outputImage:" + result.ToString() );