BayerToRgb2d
Applies a demosaicing algorithm on grayscale image with color meaning acquired with a Bayer filter camera.
Access to parameter description
This algorithm creates an RGB image from a Bayer pattern image.
A Bayer pattern image is a grayscale image where each pixel contains information about only one color component. The filter pattern is 50% green, 25% red and 25% blue and follows one of the four arrangements shown in Figure 1.
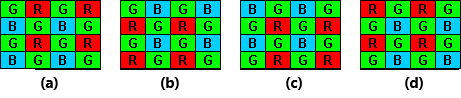
Figure 1. Four possible arrangements of a Bayer sensor: GR/BG (a), GB/RG (b), BG/GR (c) and RG/GB (d).
To convert a Bayer grayscale image into an RGB image, several methods are available. The formulas given below are based on a 5x5 area of a Bayer image with red, green, and blue pixels arranged as shown in Figure 2.
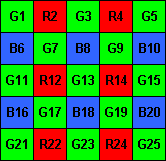
Figure 2. Identification of pixels belonging to a 5x5 window of a GR/BG Bayer image.
See also
Access to parameter description
This algorithm creates an RGB image from a Bayer pattern image.
A Bayer pattern image is a grayscale image where each pixel contains information about only one color component. The filter pattern is 50% green, 25% red and 25% blue and follows one of the four arrangements shown in Figure 1.
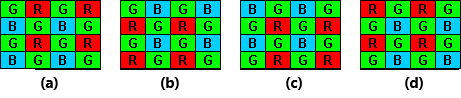
Figure 1. Four possible arrangements of a Bayer sensor: GR/BG (a), GB/RG (b), BG/GR (c) and RG/GB (d).
To convert a Bayer grayscale image into an RGB image, several methods are available. The formulas given below are based on a 5x5 area of a Bayer image with red, green, and blue pixels arranged as shown in Figure 2.
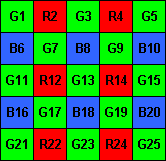
Figure 2. Identification of pixels belonging to a 5x5 window of a GR/BG Bayer image.
Bilinear interpolation
The calculation of the missing intensities is carried out with the following formulas:- $ G_8=\frac{G_3+G_7+G_9+G_{13}}{4} $
- $ B_7=\frac{B_3+B_8}{2}, B_{12}=\frac{B_6+B_8+B_{16}+B_{18}}{4} $
- $ R_7=\frac{R_2+R_{12}}{2}, R_8=\frac{R_2+R_4+R_{12}+G_{14}}{4} $
Interpolation of color ratios
The calculation of the missing intensities is carried out with the following formulas:- $ G_8=\frac{G_3+G_7+G_9+G_{13}}{4} $
- $ B_7=\frac{G_7}{2}+\left (\frac{B_8}{G_8}\right ), B_{12}=\frac{G_{12}}{4}+ \left (\frac{B_6}{G_6}+\frac{B_8}{G_8}+\frac{B_{16}}{G_{16}}+\frac{B_{18}}{G_{18}} \right ) $
- $ R_7=\frac{G_7}{2}+\left (\frac{R_2}{G_2}+\frac{R_{12}}{G_{12}}\right ), R_8=\frac{G_8}{4}+ \left (\frac{R_2}{G_2}+\frac{R_4}{G_4}+\frac{R_{12}}{G_{12}}+\frac{R_{14}}{G_{14}} \right ) $
Improved interpolation of color ratios
To improve the results, we carry out a mixing of the two preceding methods on the blue and red interpolated pixels.- $I_1$: Intensity of the bilinear interpolation
- $I_2$: Intensity of the interpolation of color ratios
- $M$: Maximum possible intensity of the image
- $G_m$: Minimal intensity of the neighbour green pixels
Interpolation by gradient and color ratios
This method consists in improving the interpolated values for the green pixels and therefore improving the previous interpolation of the red and blue pixels. $$ \Delta H_{G8}=\left |G_7-G_9 \right | ~~~~ \Delta V_{G8}=|G_3-G_{13}| $$ $$ \left\{\begin{matrix} if (\Delta H_{G8} < \Delta V_{G8}) & G_8=\frac{G_7+G_9}{2} \\ if (\Delta H_{G8} > \Delta V_{G8}) & G_8=\frac{G_3+G_{13}}{2} \\ elsewhere & G_8=\frac{G_7+G_9+G_3+G_{13}}{4} \end{matrix}\right. $$See also
Function Syntax
This function returns outputColorImage.
// Function prototype
std::shared_ptr< iolink::ImageView > bayerToRgb2d( std::shared_ptr< iolink::ImageView > inputGrayImage, BayerToRgb2d::FilterMode filterMode, BayerToRgb2d::InterpolationMode interpolationMode, std::shared_ptr< iolink::ImageView > outputColorImage = nullptr );
This function returns outputColorImage.
// Function prototype. bayer_to_rgb_2d(input_gray_image: idt.ImageType, filter_mode: BayerToRgb2d.FilterMode = BayerToRgb2d.FilterMode.GR_BG, interpolation_mode: BayerToRgb2d.InterpolationMode = BayerToRgb2d.InterpolationMode.BILINEAR, output_color_image: idt.ImageType = None) -> idt.ImageType
This function returns outputColorImage.
// Function prototype. public static IOLink.ImageView BayerToRgb2d( IOLink.ImageView inputGrayImage, BayerToRgb2d.FilterMode filterMode = ImageDev.BayerToRgb2d.FilterMode.GR_BG, BayerToRgb2d.InterpolationMode interpolationMode = ImageDev.BayerToRgb2d.InterpolationMode.BILINEAR, IOLink.ImageView outputColorImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
inputGrayImage |
The Bayer grayscale input image. | Image | Grayscale | nullptr | ||||||||
![]() |
filterMode |
The Bayer filter pattern.
|
Enumeration | GR_BG | |||||||||
![]() |
interpolationMode |
The interpolation algorithm for demosaicing.
|
Enumeration | BILINEAR | |||||||||
![]() |
outputColorImage |
The color output image. Its spatial dimensions and type are forced to the same values as the input. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
input_gray_image |
The Bayer grayscale input image. | image | Grayscale | None | ||||||||
![]() |
filter_mode |
The Bayer filter pattern.
|
enumeration | GR_BG | |||||||||
![]() |
interpolation_mode |
The interpolation algorithm for demosaicing.
|
enumeration | BILINEAR | |||||||||
![]() |
output_color_image |
The color output image. Its spatial dimensions and type are forced to the same values as the input. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
inputGrayImage |
The Bayer grayscale input image. | Image | Grayscale | null | ||||||||
![]() |
filterMode |
The Bayer filter pattern.
|
Enumeration | GR_BG | |||||||||
![]() |
interpolationMode |
The interpolation algorithm for demosaicing.
|
Enumeration | BILINEAR | |||||||||
![]() |
outputColorImage |
The color output image. Its spatial dimensions and type are forced to the same values as the input. | Image | null |
Object Examples
auto polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); BayerToRgb2d bayerToRgb2dAlgo; bayerToRgb2dAlgo.setInputGrayImage( polystyrene ); bayerToRgb2dAlgo.setFilterMode( BayerToRgb2d::FilterMode::GR_BG ); bayerToRgb2dAlgo.setInterpolationMode( BayerToRgb2d::InterpolationMode::BILINEAR ); bayerToRgb2dAlgo.execute(); std::cout << "outputColorImage:" << bayerToRgb2dAlgo.outputColorImage()->toString();
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) bayer_to_rgb_2d_algo = imagedev.BayerToRgb2d() bayer_to_rgb_2d_algo.input_gray_image = polystyrene bayer_to_rgb_2d_algo.filter_mode = imagedev.BayerToRgb2d.GR_BG bayer_to_rgb_2d_algo.interpolation_mode = imagedev.BayerToRgb2d.BILINEAR bayer_to_rgb_2d_algo.execute() print("output_color_image:", str(bayer_to_rgb_2d_algo.output_color_image))
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); BayerToRgb2d bayerToRgb2dAlgo = new BayerToRgb2d { inputGrayImage = polystyrene, filterMode = BayerToRgb2d.FilterMode.GR_BG, interpolationMode = BayerToRgb2d.InterpolationMode.BILINEAR }; bayerToRgb2dAlgo.Execute(); Console.WriteLine( "outputColorImage:" + bayerToRgb2dAlgo.outputColorImage.ToString() );
Function Examples
auto polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); auto result = bayerToRgb2d( polystyrene, BayerToRgb2d::FilterMode::GR_BG, BayerToRgb2d::InterpolationMode::BILINEAR ); std::cout << "outputColorImage:" << result->toString();
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) result = imagedev.bayer_to_rgb_2d(polystyrene, imagedev.BayerToRgb2d.GR_BG, imagedev.BayerToRgb2d.BILINEAR) print("output_color_image:", str(result))
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); IOLink.ImageView result = Processing.BayerToRgb2d( polystyrene, BayerToRgb2d.FilterMode.GR_BG, BayerToRgb2d.InterpolationMode.BILINEAR ); Console.WriteLine( "outputColorImage:" + result.ToString() );