CudaErosion2d
Performs a two-dimensional erosion using a structuring element matching with a square or a cross. The calculations are performed on the GPU.
Access to parameter description
This command is experimental, his signature may be modified between now and his final version.
For an introduction:
This algorithm uses a basic structuring element with either 8 neighbors, or 4 neighbors, according to the neighborhood parameter.
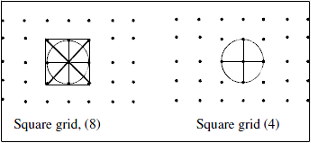
Figure 1. Structuring elements
See also
Access to parameter description
This command is experimental, his signature may be modified between now and his final version.
For an introduction:
- section Mathematical Morphology
- section Introduction To Erosion
This algorithm uses a basic structuring element with either 8 neighbors, or 4 neighbors, according to the neighborhood parameter.
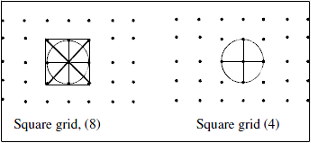
Figure 1. Structuring elements
Function Syntax
This function returns outputImage.
// Function prototype
std::shared_ptr< iolink::ImageView > cudaErosion2d( std::shared_ptr< iolink::ImageView > inputImage, uint32_t kernelRadius, CudaErosion2d::Neighborhood neighborhood, CudaErosion2d::TilingMode tilingMode, iolink::Vector2u32 tileSize, CudaContext::Ptr cudaContext, std::shared_ptr< iolink::ImageView > outputImage = nullptr );
This function returns outputImage.
// Function prototype. cuda_erosion_2d(input_image: idt.ImageType, kernel_radius: int = 3, neighborhood: CudaErosion2d.Neighborhood = CudaErosion2d.Neighborhood.CONNECTIVITY_8, tiling_mode: CudaErosion2d.TilingMode = CudaErosion2d.TilingMode.NONE, tile_size: Iterable[int] = [1024, 1024], cuda_context: Union[CudaContext, None] = None, output_image: idt.ImageType = None) -> idt.ImageType
This function returns outputImage.
// Function prototype. public static IOLink.ImageView CudaErosion2d( IOLink.ImageView inputImage, UInt32 kernelRadius = 3, CudaErosion2d.Neighborhood neighborhood = ImageDev.CudaErosion2d.Neighborhood.CONNECTIVITY_8, CudaErosion2d.TilingMode tilingMode = ImageDev.CudaErosion2d.TilingMode.NONE, uint[] tileSize = null, Data.CudaContext cudaContext = null, IOLink.ImageView outputImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input image. The image type can be integer or float. | Image | Binary, Label, Grayscale or Multispectral | nullptr | ||||
![]() |
kernelRadius |
The number of iterations (the half size of the structuring element, in pixels). A square structuring element always has an odd side length (3x3, 5x5, etc.) which is defined by twice the kernel radius + 1. | UInt32 | >=1 | 3 | ||||
![]() |
neighborhood |
The 2D neighborhood configuration.
|
Enumeration | CONNECTIVITY_8 | |||||
![]() |
tilingMode |
The way to manage the GPU memory.
|
Enumeration | NONE | |||||
![]() |
tileSize |
The tile width and height in pixels. This parameter is used only in USER_DEFINED tiling mode. | Vector2u32 | >=3 | {1024, 1024} | ||||
![]() |
cudaContext |
CUDA context information. | CudaContext | nullptr | |||||
![]() |
outputImage |
The output image. Its dimensions and type are forced to the same values as the input image. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
input_image |
The input image. The image type can be integer or float. | image | Binary, Label, Grayscale or Multispectral | None | ||||
![]() |
kernel_radius |
The number of iterations (the half size of the structuring element, in pixels). A square structuring element always has an odd side length (3x3, 5x5, etc.) which is defined by twice the kernel radius + 1. | uint32 | >=1 | 3 | ||||
![]() |
neighborhood |
The 2D neighborhood configuration.
|
enumeration | CONNECTIVITY_8 | |||||
![]() |
tiling_mode |
The way to manage the GPU memory.
|
enumeration | NONE | |||||
![]() |
tile_size |
The tile width and height in pixels. This parameter is used only in USER_DEFINED tiling mode. | vector2u32 | >=3 | [1024, 1024] | ||||
![]() |
cuda_context |
CUDA context information. | cuda_context | None | |||||
![]() |
output_image |
The output image. Its dimensions and type are forced to the same values as the input image. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input image. The image type can be integer or float. | Image | Binary, Label, Grayscale or Multispectral | null | ||||
![]() |
kernelRadius |
The number of iterations (the half size of the structuring element, in pixels). A square structuring element always has an odd side length (3x3, 5x5, etc.) which is defined by twice the kernel radius + 1. | UInt32 | >=1 | 3 | ||||
![]() |
neighborhood |
The 2D neighborhood configuration.
|
Enumeration | CONNECTIVITY_8 | |||||
![]() |
tilingMode |
The way to manage the GPU memory.
|
Enumeration | NONE | |||||
![]() |
tileSize |
The tile width and height in pixels. This parameter is used only in USER_DEFINED tiling mode. | Vector2u32 | >=3 | {1024, 1024} | ||||
![]() |
cudaContext |
CUDA context information. | CudaContext | null | |||||
![]() |
outputImage |
The output image. Its dimensions and type are forced to the same values as the input image. | Image | null |
Object Examples
auto polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); CudaErosion2d cudaErosion2dAlgo; cudaErosion2dAlgo.setInputImage( polystyrene ); cudaErosion2dAlgo.setKernelRadius( 3 ); cudaErosion2dAlgo.setNeighborhood( CudaErosion2d::Neighborhood::CONNECTIVITY_8 ); cudaErosion2dAlgo.setTilingMode( CudaErosion2d::TilingMode::NONE ); cudaErosion2dAlgo.setTileSize( {264, 264} ); cudaErosion2dAlgo.setCudaContext( nullptr ); cudaErosion2dAlgo.setOutputImage( iolink::ImageViewFactory::allocate( iolink::VectorXu64( { 1, 1 } ), iolink::DataTypeId::UINT8 ) ); cudaErosion2dAlgo.execute(); std::cout << "outputImage:" << cudaErosion2dAlgo.outputImage()->toString();
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) cuda_erosion_2d_algo = imagedev.CudaErosion2d() cuda_erosion_2d_algo.input_image = polystyrene cuda_erosion_2d_algo.kernel_radius = 3 cuda_erosion_2d_algo.neighborhood = imagedev.CudaErosion2d.CONNECTIVITY_8 cuda_erosion_2d_algo.tiling_mode = imagedev.CudaErosion2d.NONE cuda_erosion_2d_algo.tile_size = [264, 264] cuda_erosion_2d_algo.cuda_context = None cuda_erosion_2d_algo.execute() print("output_image:", str(cuda_erosion_2d_algo.output_image))
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); CudaErosion2d cudaErosion2dAlgo = new CudaErosion2d { inputImage = polystyrene, kernelRadius = 3, neighborhood = CudaErosion2d.Neighborhood.CONNECTIVITY_8, tilingMode = CudaErosion2d.TilingMode.NONE, tileSize = new uint[]{264, 264}, cudaContext = null }; cudaErosion2dAlgo.Execute(); Console.WriteLine( "outputImage:" + cudaErosion2dAlgo.outputImage.ToString() );
Function Examples
auto polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); auto result = cudaErosion2d( polystyrene, 3, CudaErosion2d::Neighborhood::CONNECTIVITY_8, CudaErosion2d::TilingMode::NONE, {264, 264}, nullptr , iolink::ImageViewFactory::allocate( iolink::VectorXu64( { 1, 1 } ), iolink::DataTypeId::UINT8 )); std::cout << "outputImage:" << result->toString();
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) result = imagedev.cuda_erosion_2d(polystyrene, 3, imagedev.CudaErosion2d.CONNECTIVITY_8, imagedev.CudaErosion2d.NONE, [264, 264], None) print("output_image:", str(result))
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); IOLink.ImageView result = Processing.CudaErosion2d( polystyrene, 3, CudaErosion2d.Neighborhood.CONNECTIVITY_8, CudaErosion2d.TilingMode.NONE, new uint[]{264, 264}, null ); Console.WriteLine( "outputImage:" + result.ToString() );