SieveLabeling
Produces a new label image by assigning a same label to objects belonging to a same class of a user-defined measurement.
Access to parameter description
As an introduction:
This algorithm produces a new label image by grouping objects of the input label or binary image. The objects are then assigned to bins using a measurement and a sieve array. The output labels are set accordingly.
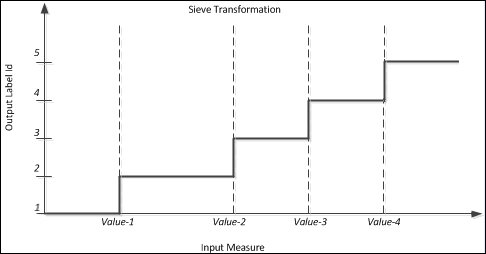
Figure 1. Label grouping based on a sieve set
First, a user-defined measurement is computed for each object of the input image. Then, each object of the input image is assigned to an output label depending on the value of this measurement. The Figure 1 illustrates the label assignment procedure:
Figure 2. Sieve labeling applied on a binary image with the Area2d measurement.
See also
Access to parameter description
As an introduction:
This algorithm produces a new label image by grouping objects of the input label or binary image. The objects are then assigned to bins using a measurement and a sieve array. The output labels are set accordingly.
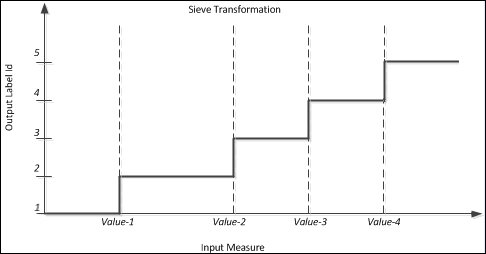
Figure 1. Label grouping based on a sieve set
First, a user-defined measurement is computed for each object of the input image. Then, each object of the input image is assigned to an output label depending on the value of this measurement. The Figure 1 illustrates the label assignment procedure:
- An object which measurement falls between 2 sieve values is assigned to a corresponding label. For instance, all objects between value-1 and value-2 are assigned to the label 2.
- Objects which measurement is less than the first sieve value are assigned to 1.
- Objects which measurement is greater than the last sieve value are assigned to the greatest label value (the number of sieve bounds + 1).
- If N sieve values have been provided as input, the output image can contain up to N+1 different labels.
![]() |
![]() |
See also
Function Syntax
This function returns outputLabelImage.
// Function prototype
std::shared_ptr< iolink::ImageView > sieveLabeling( std::shared_ptr< iolink::ImageView > inputObjectImage, std::shared_ptr< iolink::ImageView > inputIntensityImage, std::vector<double> sieveArray, std::string measurement, std::shared_ptr< iolink::ImageView > outputLabelImage = nullptr );
This function returns outputLabelImage.
// Function prototype. sieve_labeling(input_object_image: idt.ImageType, input_intensity_image: idt.ImageType, sieve_array: Union[Sequence[int], Sequence[float]] = [10, 100, 1000, 10000], measurement: str = "", output_label_image: idt.ImageType = None) -> idt.ImageType
This function returns outputLabelImage.
// Function prototype. public static IOLink.ImageView SieveLabeling( IOLink.ImageView inputObjectImage, IOLink.ImageView inputIntensityImage, double[] sieveArray = null, string measurement = "", IOLink.ImageView outputLabelImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputObjectImage |
The input binary or label image. | Image | Binary or Label | nullptr |
![]() |
inputIntensityImage |
The input grayscale image that contains the intensity information. It must have the same dimensions as the label input image. It is only used with measurements requiring an intensity image, and is ignored with other measurements. If it equals null, the label input image will be used as the intensity input image. | Image | Binary, Label, Grayscale or Multispectral | nullptr |
![]() |
sieveArray |
The array defining the bounds values of the sieve to apply. | Sieve | {10, 100, 1000, 10000} | |
![]() |
measurement |
The native measurement on which the sieve is applied. | Measurement | "" | |
![]() |
outputLabelImage |
The output label image. Its dimensions are forced to the same values as the input. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
input_object_image |
The input binary or label image. | image | Binary or Label | None |
![]() |
input_intensity_image |
The input grayscale image that contains the intensity information. It must have the same dimensions as the label input image. It is only used with measurements requiring an intensity image, and is ignored with other measurements. If it equals null, the label input image will be used as the intensity input image. | image | Binary, Label, Grayscale or Multispectral | None |
![]() |
sieve_array |
The array defining the bounds values of the sieve to apply. | sieve | [10, 100, 1000, 10000] | |
![]() |
measurement |
The native measurement on which the sieve is applied. | measurement | "" | |
![]() |
output_label_image |
The output label image. Its dimensions are forced to the same values as the input. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputObjectImage |
The input binary or label image. | Image | Binary or Label | null |
![]() |
inputIntensityImage |
The input grayscale image that contains the intensity information. It must have the same dimensions as the label input image. It is only used with measurements requiring an intensity image, and is ignored with other measurements. If it equals null, the label input image will be used as the intensity input image. | Image | Binary, Label, Grayscale or Multispectral | null |
![]() |
sieveArray |
The array defining the bounds values of the sieve to apply. | Sieve | {10, 100, 1000, 10000} | |
![]() |
measurement |
The native measurement on which the sieve is applied. | Measurement | "" | |
![]() |
outputLabelImage |
The output label image. Its dimensions are forced to the same values as the input. | Image | null |
Object Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); auto polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); SieveLabeling sieveLabelingAlgo; sieveLabelingAlgo.setInputObjectImage( polystyrene_sep ); sieveLabelingAlgo.setInputIntensityImage( polystyrene ); sieveLabelingAlgo.setSieveArray( {1800, 2000, 2300, 2600, 3000} ); sieveLabelingAlgo.setMeasurement( "Area" ); sieveLabelingAlgo.execute(); std::cout << "outputLabelImage:" << sieveLabelingAlgo.outputLabelImage()->toString();
polystyrene_sep = imagedev.read_vip_image(imagedev_data.get_image_path("polystyrene_sep.vip")) polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) sieve_labeling_algo = imagedev.SieveLabeling() sieve_labeling_algo.input_object_image = polystyrene_sep sieve_labeling_algo.input_intensity_image = polystyrene sieve_labeling_algo.sieve_array = [1800, 2000, 2300, 2600, 3000] sieve_labeling_algo.measurement = "Area" sieve_labeling_algo.execute() print("output_label_image:", str(sieve_labeling_algo.output_label_image))
ImageView polystyrene_sep = Data.ReadVipImage( @"Data/images/polystyrene_sep.vip" ); ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); SieveLabeling sieveLabelingAlgo = new SieveLabeling { inputObjectImage = polystyrene_sep, inputIntensityImage = polystyrene, sieveArray = new double[]{1800, 2000, 2300, 2600, 3000}, measurement = "Area" }; sieveLabelingAlgo.Execute(); Console.WriteLine( "outputLabelImage:" + sieveLabelingAlgo.outputLabelImage.ToString() );
Function Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); auto polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); auto result = sieveLabeling( polystyrene_sep, polystyrene, {1800, 2000, 2300, 2600, 3000}, "Area" ); std::cout << "outputLabelImage:" << result->toString();
polystyrene_sep = imagedev.read_vip_image(imagedev_data.get_image_path("polystyrene_sep.vip")) polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) result = imagedev.sieve_labeling(polystyrene_sep, polystyrene, [1800, 2000, 2300, 2600, 3000], "Area") print("output_label_image:", str(result))
ImageView polystyrene_sep = Data.ReadVipImage( @"Data/images/polystyrene_sep.vip" ); ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); IOLink.ImageView result = Processing.SieveLabeling( polystyrene_sep, polystyrene, new double[]{1800, 2000, 2300, 2600, 3000}, "Area" ); Console.WriteLine( "outputLabelImage:" + result.ToString() );