ReconstructionFromMarkers2d
Rebuilds a binary image starting from markers.
Access to parameter description
For an introduction:
Successive dilations are performed on the markers, each dilation being followed by an intersection with the original image until convergence (idempotence).
This iterative process is called a geodesic dilation.
It guarantees that a dilated marker will not merge with a particle other than the one it marks.
Geodesic dilation is different from a large dilation of a given size followed by an intersection with the original image because a dilated marker may hit close particles that are not marked for reconstruction. This difference is illustrated in Figure 1.
For example, given an erosion which eliminated the small particles of an image, this algorithm can rebuild the original shapes of the remaining particles if the eroded set is used as the markers. This sequence is called an opening by reconstruction.
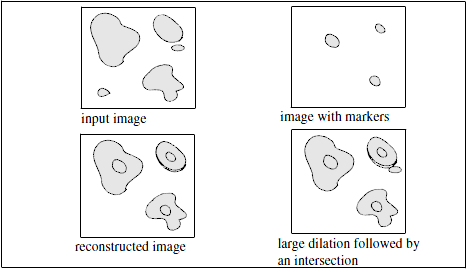
Figure 1. Comparison between reconstruction from markers and dilation/intersection
See also
Access to parameter description
For an introduction:
- section Mathematical Morphology
- section Geodesic Transformations
Successive dilations are performed on the markers, each dilation being followed by an intersection with the original image until convergence (idempotence).
This iterative process is called a geodesic dilation.
It guarantees that a dilated marker will not merge with a particle other than the one it marks.
Geodesic dilation is different from a large dilation of a given size followed by an intersection with the original image because a dilated marker may hit close particles that are not marked for reconstruction. This difference is illustrated in Figure 1.
For example, given an erosion which eliminated the small particles of an image, this algorithm can rebuild the original shapes of the remaining particles if the eroded set is used as the markers. This sequence is called an opening by reconstruction.
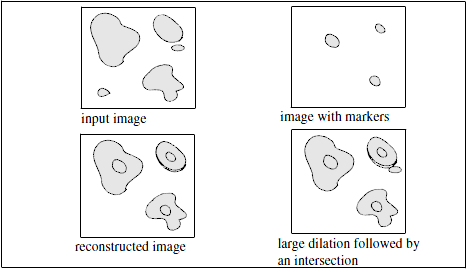
Figure 1. Comparison between reconstruction from markers and dilation/intersection
See also
Function Syntax
This function returns outputImage.
// Function prototype
std::shared_ptr< iolink::ImageView > reconstructionFromMarkers2d( std::shared_ptr< iolink::ImageView > inputImage, std::shared_ptr< iolink::ImageView > inputMarkerImage, ReconstructionFromMarkers2d::Neighborhood neighborhood, std::shared_ptr< iolink::ImageView > outputImage = nullptr );
This function returns outputImage.
// Function prototype. reconstruction_from_markers_2d(input_image: idt.ImageType, input_marker_image: idt.ImageType, neighborhood: ReconstructionFromMarkers2d.Neighborhood = ReconstructionFromMarkers2d.Neighborhood.CONNECTIVITY_8, output_image: idt.ImageType = None) -> idt.ImageType
This function returns outputImage.
// Function prototype. public static IOLink.ImageView ReconstructionFromMarkers2d( IOLink.ImageView inputImage, IOLink.ImageView inputMarkerImage, ReconstructionFromMarkers2d.Neighborhood neighborhood = ImageDev.ReconstructionFromMarkers2d.Neighborhood.CONNECTIVITY_8, IOLink.ImageView outputImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The binary mask image constraining reconstruction. | Image | Binary | nullptr | ||||
![]() |
inputMarkerImage |
The binary marker image containing seeds for reconstruction. It must have the same dimensions and type as the input image. | Image | Binary | nullptr | ||||
![]() |
neighborhood |
The 2D neighborhood configuration for performing dilations or erosions.
|
Enumeration | CONNECTIVITY_8 | |||||
![]() |
outputImage |
The binary output image. Its dimensions and type are forced to the same values as the input. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
input_image |
The binary mask image constraining reconstruction. | image | Binary | None | ||||
![]() |
input_marker_image |
The binary marker image containing seeds for reconstruction. It must have the same dimensions and type as the input image. | image | Binary | None | ||||
![]() |
neighborhood |
The 2D neighborhood configuration for performing dilations or erosions.
|
enumeration | CONNECTIVITY_8 | |||||
![]() |
output_image |
The binary output image. Its dimensions and type are forced to the same values as the input. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The binary mask image constraining reconstruction. | Image | Binary | null | ||||
![]() |
inputMarkerImage |
The binary marker image containing seeds for reconstruction. It must have the same dimensions and type as the input image. | Image | Binary | null | ||||
![]() |
neighborhood |
The 2D neighborhood configuration for performing dilations or erosions.
|
Enumeration | CONNECTIVITY_8 | |||||
![]() |
outputImage |
The binary output image. Its dimensions and type are forced to the same values as the input. | Image | null |
Object Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); ReconstructionFromMarkers2d reconstructionFromMarkers2dAlgo; reconstructionFromMarkers2dAlgo.setInputImage( polystyrene_sep ); reconstructionFromMarkers2dAlgo.setInputMarkerImage( polystyrene_sep ); reconstructionFromMarkers2dAlgo.setNeighborhood( ReconstructionFromMarkers2d::Neighborhood::CONNECTIVITY_8 ); reconstructionFromMarkers2dAlgo.execute(); std::cout << "outputImage:" << reconstructionFromMarkers2dAlgo.outputImage()->toString();
polystyrene_sep = imagedev.read_vip_image(imagedev_data.get_image_path("polystyrene_sep.vip")) reconstruction_from_markers_2d_algo = imagedev.ReconstructionFromMarkers2d() reconstruction_from_markers_2d_algo.input_image = polystyrene_sep reconstruction_from_markers_2d_algo.input_marker_image = polystyrene_sep reconstruction_from_markers_2d_algo.neighborhood = imagedev.ReconstructionFromMarkers2d.CONNECTIVITY_8 reconstruction_from_markers_2d_algo.execute() print("output_image:", str(reconstruction_from_markers_2d_algo.output_image))
ImageView polystyrene_sep = Data.ReadVipImage( @"Data/images/polystyrene_sep.vip" ); ReconstructionFromMarkers2d reconstructionFromMarkers2dAlgo = new ReconstructionFromMarkers2d { inputImage = polystyrene_sep, inputMarkerImage = polystyrene_sep, neighborhood = ReconstructionFromMarkers2d.Neighborhood.CONNECTIVITY_8 }; reconstructionFromMarkers2dAlgo.Execute(); Console.WriteLine( "outputImage:" + reconstructionFromMarkers2dAlgo.outputImage.ToString() );
Function Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); auto result = reconstructionFromMarkers2d( polystyrene_sep, polystyrene_sep, ReconstructionFromMarkers2d::Neighborhood::CONNECTIVITY_8 ); std::cout << "outputImage:" << result->toString();
polystyrene_sep = imagedev.read_vip_image(imagedev_data.get_image_path("polystyrene_sep.vip")) result = imagedev.reconstruction_from_markers_2d(polystyrene_sep, polystyrene_sep, imagedev.ReconstructionFromMarkers2d.CONNECTIVITY_8) print("output_image:", str(result))
ImageView polystyrene_sep = Data.ReadVipImage( @"Data/images/polystyrene_sep.vip" ); IOLink.ImageView result = Processing.ReconstructionFromMarkers2d( polystyrene_sep, polystyrene_sep, ReconstructionFromMarkers2d.Neighborhood.CONNECTIVITY_8 ); Console.WriteLine( "outputImage:" + result.ToString() );