OrientedLinesImage2d
Creates a two-dimensional binary image containing a series of parallel oriented lines.
Access to parameter description
OrientedLinesImage2d creates a binary image with a set of parallel lines with a given orientation and separated by a given offset.
This algorithm can be used, for example, to measure the mean thickness of a metal coating into a material section by an intersection method.
The following figures illustrate this kind of process.
As a preliminary step, a binary mask image of the coating is computed.
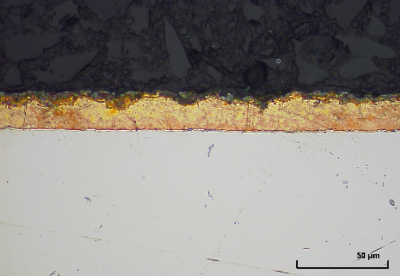
Figure 1. Material color image
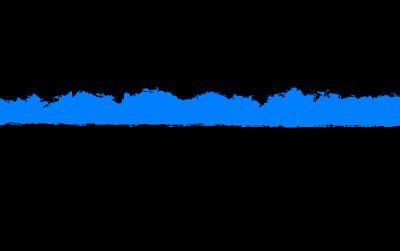
Figure 2. Coating mask segmentation
Then, a binary image composed of vertical lines can be generated with this algorithm.
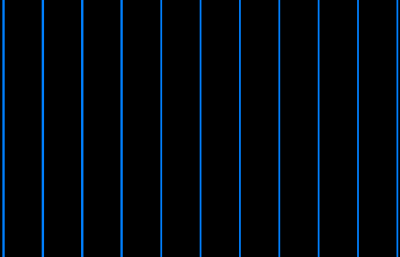
Figure 3. Oriented lines image
The binary intersection of the lines with the coating mask image is performed. The resulting image appears
in red on Figure 4.
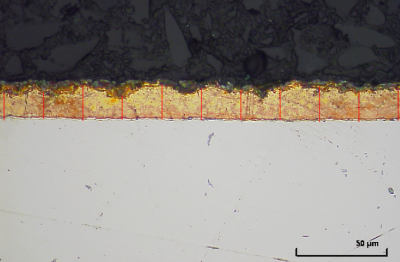
Figure 4. Intersection between coating and lines overlaying the input image
Finally, an approximation of the coating mean thickness can be computed by a quantification algorithm like:
See also
Access to parameter description
OrientedLinesImage2d creates a binary image with a set of parallel lines with a given orientation and separated by a given offset.
This algorithm can be used, for example, to measure the mean thickness of a metal coating into a material section by an intersection method.
The following figures illustrate this kind of process.
As a preliminary step, a binary mask image of the coating is computed.
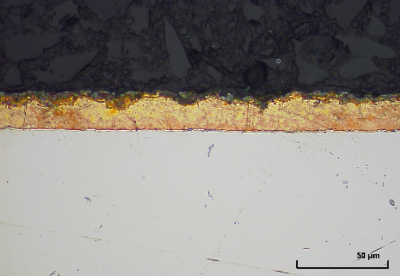
Figure 1. Material color image
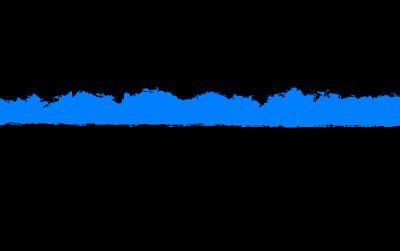
Figure 2. Coating mask segmentation
Then, a binary image composed of vertical lines can be generated with this algorithm.
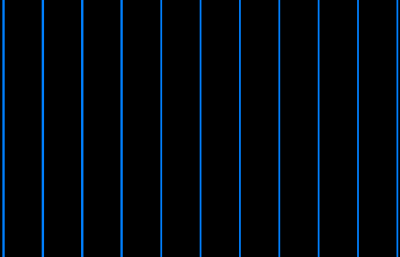
Figure 3. Oriented lines image
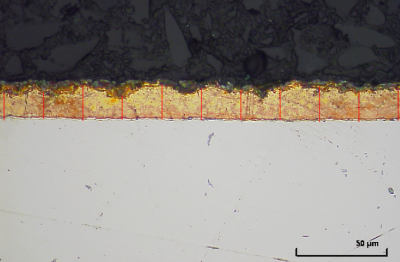
Figure 4. Intersection between coating and lines overlaying the input image
Finally, an approximation of the coating mean thickness can be computed by a quantification algorithm like:
- An individual analysis algorithm with the Length measurement.
- A global analysis algorithm measuring the area weighted by the number of lines and the pixel size.
See also
Function Syntax
This function returns outputBinaryImage.
// Function prototype
std::shared_ptr< iolink::ImageView > orientedLinesImage2d( int32_t imageSizeX, int32_t imageSizeY, double orientationAngle, double offset, std::shared_ptr< iolink::ImageView > outputBinaryImage = nullptr );
This function returns outputBinaryImage.
// Function prototype. oriented_lines_image_2d(image_size_x: int = 1024, image_size_y: int = 1024, orientation_angle: float = 10, offset: float = 100, output_binary_image: idt.ImageType = None) -> idt.ImageType
This function returns outputBinaryImage.
// Function prototype. public static IOLink.ImageView OrientedLinesImage2d( Int32 imageSizeX = 1024, Int32 imageSizeY = 1024, double orientationAngle = 10, double offset = 100, IOLink.ImageView outputBinaryImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
imageSizeX |
The X size in pixels of the output image. | Int32 | >=1 | 1024 |
![]() |
imageSizeY |
The Y size in pixels of the output image. | Int32 | >=1 | 1024 |
![]() |
orientationAngle |
The lines orientation in degrees from the X axis. | Float64 | Any value | 10 |
![]() |
offset |
The distance in pixels between two lines. | Float64 | >=1 | 100 |
![]() |
outputBinaryImage |
The output binary image. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
image_size_x |
The X size in pixels of the output image. | int32 | >=1 | 1024 |
![]() |
image_size_y |
The Y size in pixels of the output image. | int32 | >=1 | 1024 |
![]() |
orientation_angle |
The lines orientation in degrees from the X axis. | float64 | Any value | 10 |
![]() |
offset |
The distance in pixels between two lines. | float64 | >=1 | 100 |
![]() |
output_binary_image |
The output binary image. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
imageSizeX |
The X size in pixels of the output image. | Int32 | >=1 | 1024 |
![]() |
imageSizeY |
The Y size in pixels of the output image. | Int32 | >=1 | 1024 |
![]() |
orientationAngle |
The lines orientation in degrees from the X axis. | Float64 | Any value | 10 |
![]() |
offset |
The distance in pixels between two lines. | Float64 | >=1 | 100 |
![]() |
outputBinaryImage |
The output binary image. | Image | null |
Object Examples
OrientedLinesImage2d orientedLinesImage2dAlgo; orientedLinesImage2dAlgo.setImageSizeX( 1024 ); orientedLinesImage2dAlgo.setImageSizeY( 1024 ); orientedLinesImage2dAlgo.setOrientationAngle( 10.0 ); orientedLinesImage2dAlgo.setOffset( 100.0 ); orientedLinesImage2dAlgo.execute(); std::cout << "outputBinaryImage:" << orientedLinesImage2dAlgo.outputBinaryImage()->toString();
oriented_lines_image_2d_algo = imagedev.OrientedLinesImage2d() oriented_lines_image_2d_algo.image_size_x = 1024 oriented_lines_image_2d_algo.image_size_y = 1024 oriented_lines_image_2d_algo.orientation_angle = 10.0 oriented_lines_image_2d_algo.offset = 100.0 oriented_lines_image_2d_algo.execute() print("output_binary_image:", str(oriented_lines_image_2d_algo.output_binary_image))
OrientedLinesImage2d orientedLinesImage2dAlgo = new OrientedLinesImage2d { imageSizeX = 1024, imageSizeY = 1024, orientationAngle = 10.0, offset = 100.0 }; orientedLinesImage2dAlgo.Execute(); Console.WriteLine( "outputBinaryImage:" + orientedLinesImage2dAlgo.outputBinaryImage.ToString() );
Function Examples
auto result = orientedLinesImage2d( 1024, 1024, 10.0, 100.0 ); std::cout << "outputBinaryImage:" << result->toString();
result = imagedev.oriented_lines_image_2d(1024, 1024, 10.0, 100.0) print("output_binary_image:", str(result))
IOLink.ImageView result = Processing.OrientedLinesImage2d( 1024, 1024, 10.0, 100.0 ); Console.WriteLine( "outputBinaryImage:" + result.ToString() );