OrientedLinesImage3d
Creates a three-dimensional binary image containing a series of parallel oriented lines.
Access to parameter description
OrientedLinesImage3d creates a binary image with a set of parallel lines with a given orientation and separated by a given offset.
This module creates a binary image with oriented 3D lines. The orientation of these lines is defined by two angles: the polar angle ϕ (inclination from Z axis) and the azimuthal angle θ (orientation from X axis on the XY orthogonal projection).
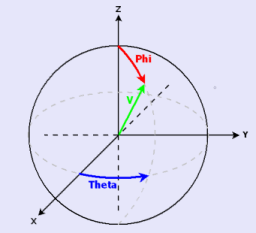
Figure 1. Mathematics convention for spherical coordinates
Considering a plane that is orthogonal to this orientation, each line crosses this plane into a single point defining a rectangular grid regularly spaced and oriented by a third angle α, as shown in Figure 2.
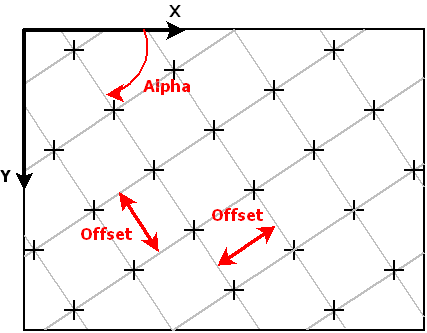
Figure 2. Orientation of the lines grid
See also
Access to parameter description
OrientedLinesImage3d creates a binary image with a set of parallel lines with a given orientation and separated by a given offset.
This module creates a binary image with oriented 3D lines. The orientation of these lines is defined by two angles: the polar angle ϕ (inclination from Z axis) and the azimuthal angle θ (orientation from X axis on the XY orthogonal projection).
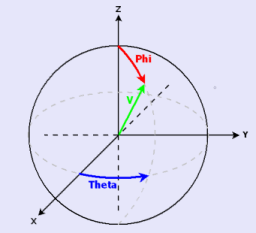
Figure 1. Mathematics convention for spherical coordinates
Considering a plane that is orthogonal to this orientation, each line crosses this plane into a single point defining a rectangular grid regularly spaced and oriented by a third angle α, as shown in Figure 2.
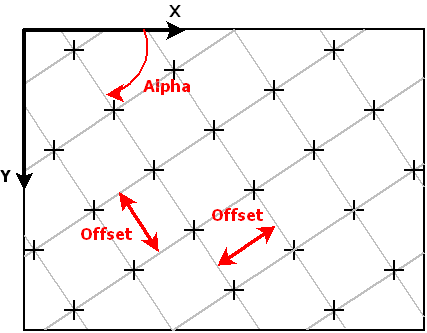
Figure 2. Orientation of the lines grid
See also
Function Syntax
This function returns outputBinaryImage.
// Function prototype
std::shared_ptr< iolink::ImageView > orientedLinesImage3d( int32_t imageSizeX, int32_t imageSizeY, int32_t imageSizeZ, double thetaAngle, double phiAngle, double alphaAngle, double offset, std::shared_ptr< iolink::ImageView > outputBinaryImage = nullptr );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
imageSizeX |
The X size in voxels of the output image. | Int32 | >=1 | 100 |
![]() |
imageSizeY |
The Y size in voxels of the output image. | Int32 | >=1 | 100 |
![]() |
imageSizeZ |
The Z size in voxels of the output image. | Int32 | >=1 | 100 |
![]() |
thetaAngle |
The polar angle in degrees of lines orientation. | Float64 | Any value | 0 |
![]() |
phiAngle |
The azimuthal angle in degrees of lines orientation. | Float64 | Any value | 0 |
![]() |
alphaAngle |
The alpha angle in degrees defining the grid orientation in a plane orthogonal to the lines direction. | Float64 | Any value | 0 |
![]() |
offset |
The distance in voxels between two lines. | Float64 | >=1 | 30 |
![]() |
outputBinaryImage |
The output binary image. | Image | nullptr |
Object Examples
OrientedLinesImage3d orientedLinesImage3dAlgo; orientedLinesImage3dAlgo.setImageSizeX( 100 ); orientedLinesImage3dAlgo.setImageSizeY( 100 ); orientedLinesImage3dAlgo.setImageSizeZ( 100 ); orientedLinesImage3dAlgo.setThetaAngle( 0.0 ); orientedLinesImage3dAlgo.setPhiAngle( 0.0 ); orientedLinesImage3dAlgo.setAlphaAngle( 0.0 ); orientedLinesImage3dAlgo.setOffset( 30.0 ); orientedLinesImage3dAlgo.execute(); std::cout << "outputBinaryImage:" << orientedLinesImage3dAlgo.outputBinaryImage()->toString();
Function Examples
auto result = orientedLinesImage3d( 100, 100, 100, 0.0, 0.0, 0.0, 30.0 ); std::cout << "outputBinaryImage:" << result->toString();