RescaleIntensity
Performs a linear scaling of the gray level values of an image.
Access to parameter description
This algorithm performs a linear scaling of the gray level values of an image to a user-specified interval. It can be applied to images with a gray level range other than [0, 255], and for short or floating point images.
In Figure 1, the input gray levels of interest are in the user-specified interval [a, b], and the output range is the interval [c, d].
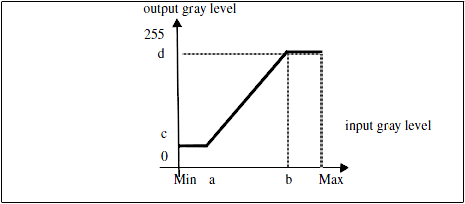
Figure 1. The intensity rescaling transformation
The input image is I, and the output image is O. Let [a, b] be the input range and [c, d] the output range, then:
$$ \begin{array}{ll} O(n,m)=c & ~\mbox{if $I(n,m) \leq a$}\\ O(n,m)=\left(\frac{d-c}{b-a}\right)(I(n,m)-a)+c & ~\mbox{if $a < I(n,m) \leq b$}\\ O(n,m)=d & ~\mbox{if $I(n,m) > b$}\end{array} $$ The type of the output image is set by an enumerate as detailed in the Data Type Management section.
See also
See related examples
Access to parameter description
This algorithm performs a linear scaling of the gray level values of an image to a user-specified interval. It can be applied to images with a gray level range other than [0, 255], and for short or floating point images.
In Figure 1, the input gray levels of interest are in the user-specified interval [a, b], and the output range is the interval [c, d].
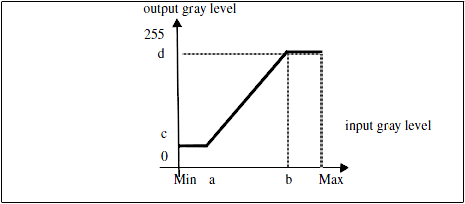
Figure 1. The intensity rescaling transformation
The input image is I, and the output image is O. Let [a, b] be the input range and [c, d] the output range, then:
$$ \begin{array}{ll} O(n,m)=c & ~\mbox{if $I(n,m) \leq a$}\\ O(n,m)=\left(\frac{d-c}{b-a}\right)(I(n,m)-a)+c & ~\mbox{if $a < I(n,m) \leq b$}\\ O(n,m)=d & ~\mbox{if $I(n,m) > b$}\end{array} $$ The type of the output image is set by an enumerate as detailed in the Data Type Management section.
See also
See related examples
Function Syntax
This function returns outputImage.
// Function prototype
std::shared_ptr< iolink::ImageView > rescaleIntensity( std::shared_ptr< iolink::ImageView > inputImage, RescaleIntensity::OutputType outputType, RescaleIntensity::RangeMode rangeMode, iolink::Vector2d percentageRange, iolink::Vector2d intensityInputRange, iolink::Vector2d intensityOutputRange, std::shared_ptr< iolink::ImageView > outputImage = nullptr );
This function returns outputImage.
// Function prototype. rescale_intensity(input_image: idt.ImageType, output_type: RescaleIntensity.OutputType = RescaleIntensity.OutputType.SAME_AS_INPUT, range_mode: RescaleIntensity.RangeMode = RescaleIntensity.RangeMode.MIN_MAX, percentage_range: Union[Iterable[int], Iterable[float]] = [2, 98], intensity_input_range: Union[Iterable[int], Iterable[float]] = [0, 255], intensity_output_range: Union[Iterable[int], Iterable[float]] = [0, 255], output_image: idt.ImageType = None) -> idt.ImageType
This function returns outputImage.
// Function prototype. public static IOLink.ImageView RescaleIntensity( IOLink.ImageView inputImage, RescaleIntensity.OutputType outputType = ImageDev.RescaleIntensity.OutputType.SAME_AS_INPUT, RescaleIntensity.RangeMode rangeMode = ImageDev.RescaleIntensity.RangeMode.MIN_MAX, double[] percentageRange = null, double[] intensityInputRange = null, double[] intensityOutputRange = null, IOLink.ImageView outputImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input image to normalize. | Image | Binary, Label, Grayscale or Multispectral | nullptr | ||||||||||||||||||
![]() |
outputType |
The output image data type.
|
Enumeration | SAME_AS_INPUT | |||||||||||||||||||
![]() |
rangeMode |
The way to define the input intensity range.
|
Enumeration | MIN_MAX | |||||||||||||||||||
![]() |
percentageRange |
The low and high histogram percentile to use when the range mode is set to PERCENTILE (in percent). This parameter is ignored with other range modes. | Vector2d | [0, 100] | {2.f, 98.f} | ||||||||||||||||||
![]() |
intensityInputRange |
The input intensity range [a, b] to use when the range mode is set to OTHER. This parameter is ignored with other range modes. | Vector2d | Any value | {0.f, 255.f} | ||||||||||||||||||
![]() |
intensityOutputRange |
The output intensity range. | Vector2d | Any value | {0.f, 255.f} | ||||||||||||||||||
![]() |
outputImage |
The output image. Its dimensions are forced to the same values as the input. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
input_image |
The input image to normalize. | image | Binary, Label, Grayscale or Multispectral | None | ||||||||||||||||||
![]() |
output_type |
The output image data type.
|
enumeration | SAME_AS_INPUT | |||||||||||||||||||
![]() |
range_mode |
The way to define the input intensity range.
|
enumeration | MIN_MAX | |||||||||||||||||||
![]() |
percentage_range |
The low and high histogram percentile to use when the range mode is set to PERCENTILE (in percent). This parameter is ignored with other range modes. | vector2d | [0, 100] | [2, 98] | ||||||||||||||||||
![]() |
intensity_input_range |
The input intensity range [a, b] to use when the range mode is set to OTHER. This parameter is ignored with other range modes. | vector2d | Any value | [0, 255] | ||||||||||||||||||
![]() |
intensity_output_range |
The output intensity range. | vector2d | Any value | [0, 255] | ||||||||||||||||||
![]() |
output_image |
The output image. Its dimensions are forced to the same values as the input. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input image to normalize. | Image | Binary, Label, Grayscale or Multispectral | null | ||||||||||||||||||
![]() |
outputType |
The output image data type.
|
Enumeration | SAME_AS_INPUT | |||||||||||||||||||
![]() |
rangeMode |
The way to define the input intensity range.
|
Enumeration | MIN_MAX | |||||||||||||||||||
![]() |
percentageRange |
The low and high histogram percentile to use when the range mode is set to PERCENTILE (in percent). This parameter is ignored with other range modes. | Vector2d | [0, 100] | {2f, 98f} | ||||||||||||||||||
![]() |
intensityInputRange |
The input intensity range [a, b] to use when the range mode is set to OTHER. This parameter is ignored with other range modes. | Vector2d | Any value | {0f, 255f} | ||||||||||||||||||
![]() |
intensityOutputRange |
The output intensity range. | Vector2d | Any value | {0f, 255f} | ||||||||||||||||||
![]() |
outputImage |
The output image. Its dimensions are forced to the same values as the input. | Image | null |
Object Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); RescaleIntensity rescaleIntensityAlgo; rescaleIntensityAlgo.setInputImage( foam ); rescaleIntensityAlgo.setOutputType( RescaleIntensity::OutputType::SAME_AS_INPUT ); rescaleIntensityAlgo.setRangeMode( RescaleIntensity::RangeMode::MIN_MAX ); rescaleIntensityAlgo.setPercentageRange( {2, 98} ); rescaleIntensityAlgo.setIntensityInputRange( {0, 255} ); rescaleIntensityAlgo.setIntensityOutputRange( {0, 255} ); rescaleIntensityAlgo.execute(); std::cout << "outputImage:" << rescaleIntensityAlgo.outputImage()->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) rescale_intensity_algo = imagedev.RescaleIntensity() rescale_intensity_algo.input_image = foam rescale_intensity_algo.output_type = imagedev.RescaleIntensity.SAME_AS_INPUT rescale_intensity_algo.range_mode = imagedev.RescaleIntensity.MIN_MAX rescale_intensity_algo.percentage_range = [2, 98] rescale_intensity_algo.intensity_input_range = [0, 255] rescale_intensity_algo.intensity_output_range = [0, 255] rescale_intensity_algo.execute() print("output_image:", str(rescale_intensity_algo.output_image))
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); RescaleIntensity rescaleIntensityAlgo = new RescaleIntensity { inputImage = foam, outputType = RescaleIntensity.OutputType.SAME_AS_INPUT, rangeMode = RescaleIntensity.RangeMode.MIN_MAX, percentageRange = new double[]{2, 98}, intensityInputRange = new double[]{0, 255}, intensityOutputRange = new double[]{0, 255} }; rescaleIntensityAlgo.Execute(); Console.WriteLine( "outputImage:" + rescaleIntensityAlgo.outputImage.ToString() );
Function Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto result = rescaleIntensity( foam, RescaleIntensity::OutputType::SAME_AS_INPUT, RescaleIntensity::RangeMode::MIN_MAX, {2, 98}, {0, 255}, {0, 255} ); std::cout << "outputImage:" << result->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) result = imagedev.rescale_intensity(foam, imagedev.RescaleIntensity.SAME_AS_INPUT, imagedev.RescaleIntensity.MIN_MAX, [2, 98], [0, 255], [0, 255]) print("output_image:", str(result))
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); IOLink.ImageView result = Processing.RescaleIntensity( foam, RescaleIntensity.OutputType.SAME_AS_INPUT, RescaleIntensity.RangeMode.MIN_MAX, new double[]{2, 98}, new double[]{0, 255}, new double[]{0, 255} ); Console.WriteLine( "outputImage:" + result.ToString() );