ColorAntialiasing2d
Reduces color artifacts with a demosaicing algorithm.
Access to parameter description
This algorithm reduces the color artifacts. These artifacts can occur when a color image is acquired with a camera equipped with a single sensor array.
Each individual sensor is able to capture only a single color because of the arrangement of color films or dyes between the sensor and the lens.
A demosaicing algorithm is a method for reconstructing a full three-color representation of color images by estimating the missing pixel components in each color plane.
The algorithm repeats several times the following process:
The number of times that the process is iterated is also user-defined.
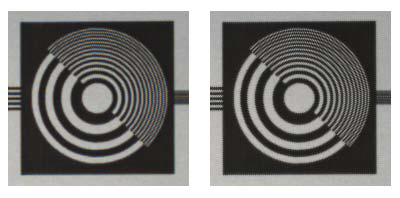
Figure 1. Antialiasing effect.
See also
Access to parameter description
This algorithm reduces the color artifacts. These artifacts can occur when a color image is acquired with a camera equipped with a single sensor array.
Each individual sensor is able to capture only a single color because of the arrangement of color films or dyes between the sensor and the lens.
A demosaicing algorithm is a method for reconstructing a full three-color representation of color images by estimating the missing pixel components in each color plane.
The algorithm repeats several times the following process:
- $ R = mean(R - G) + G $
- $ B = mean(B - G) + G $
- $ G = (mean(G - R) + mean(G - B) + R + B) $
The number of times that the process is iterated is also user-defined.
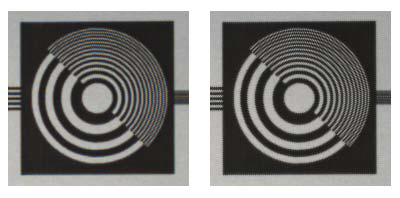
Figure 1. Antialiasing effect.
See also
Function Syntax
This function returns outputColorImage.
// Function prototype
std::shared_ptr< iolink::ImageView > colorAntialiasing2d( std::shared_ptr< iolink::ImageView > inputColorImage, int32_t kernelSize, int32_t numberOfIterations, std::shared_ptr< iolink::ImageView > outputColorImage = nullptr );
This function returns outputColorImage.
// Function prototype. color_antialiasing_2d(input_color_image: idt.ImageType, kernel_size: int = 3, number_of_iterations: int = 1, output_color_image: idt.ImageType = None) -> idt.ImageType
This function returns outputColorImage.
// Function prototype. public static IOLink.ImageView ColorAntialiasing2d( IOLink.ImageView inputColorImage, Int32 kernelSize = 3, Int32 numberOfIterations = 1, IOLink.ImageView outputColorImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputColorImage |
The color input image. | Image | Multispectral | nullptr |
![]() |
kernelSize |
The size of the kernel (the side in pixels of the square window). | Int32 | >=1 | 3 |
![]() |
numberOfIterations |
The number of iterations of the demosaicing process (must be a positive integer). | Int32 | >=1 | 1 |
![]() |
outputColorImage |
The color output image. Its dimensions and type are forced to the same values as the input. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
input_color_image |
The color input image. | image | Multispectral | None |
![]() |
kernel_size |
The size of the kernel (the side in pixels of the square window). | int32 | >=1 | 3 |
![]() |
number_of_iterations |
The number of iterations of the demosaicing process (must be a positive integer). | int32 | >=1 | 1 |
![]() |
output_color_image |
The color output image. Its dimensions and type are forced to the same values as the input. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputColorImage |
The color input image. | Image | Multispectral | null |
![]() |
kernelSize |
The size of the kernel (the side in pixels of the square window). | Int32 | >=1 | 3 |
![]() |
numberOfIterations |
The number of iterations of the demosaicing process (must be a positive integer). | Int32 | >=1 | 1 |
![]() |
outputColorImage |
The color output image. Its dimensions and type are forced to the same values as the input. | Image | null |
Object Examples
auto ateneub = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "ateneub.jpg" ); ColorAntialiasing2d colorAntialiasing2dAlgo; colorAntialiasing2dAlgo.setInputColorImage( ateneub ); colorAntialiasing2dAlgo.setKernelSize( 3 ); colorAntialiasing2dAlgo.setNumberOfIterations( 1 ); colorAntialiasing2dAlgo.execute(); std::cout << "outputColorImage:" << colorAntialiasing2dAlgo.outputColorImage()->toString();
ateneub = ioformat.read_image(imagedev_data.get_image_path("ateneub.jpg")) color_antialiasing_2d_algo = imagedev.ColorAntialiasing2d() color_antialiasing_2d_algo.input_color_image = ateneub color_antialiasing_2d_algo.kernel_size = 3 color_antialiasing_2d_algo.number_of_iterations = 1 color_antialiasing_2d_algo.execute() print("output_color_image:", str(color_antialiasing_2d_algo.output_color_image))
ImageView ateneub = ViewIO.ReadImage( @"Data/images/ateneub.jpg" ); ColorAntialiasing2d colorAntialiasing2dAlgo = new ColorAntialiasing2d { inputColorImage = ateneub, kernelSize = 3, numberOfIterations = 1 }; colorAntialiasing2dAlgo.Execute(); Console.WriteLine( "outputColorImage:" + colorAntialiasing2dAlgo.outputColorImage.ToString() );
Function Examples
auto ateneub = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "ateneub.jpg" ); auto result = colorAntialiasing2d( ateneub, 3, 1 ); std::cout << "outputColorImage:" << result->toString();
ateneub = ioformat.read_image(imagedev_data.get_image_path("ateneub.jpg")) result = imagedev.color_antialiasing_2d(ateneub, 3, 1) print("output_color_image:", str(result))
ImageView ateneub = ViewIO.ReadImage( @"Data/images/ateneub.jpg" ); IOLink.ImageView result = Processing.ColorAntialiasing2d( ateneub, 3, 1 ); Console.WriteLine( "outputColorImage:" + result.ToString() );