FillHoles3d
Fills the holes inside particles of a three-dimensional binary image.
Access to parameter description
For an introduction:
Holes inside objects are filled using a numerical reconstruction applied on the complement of the input image, from markers made of voxels of the image frame.
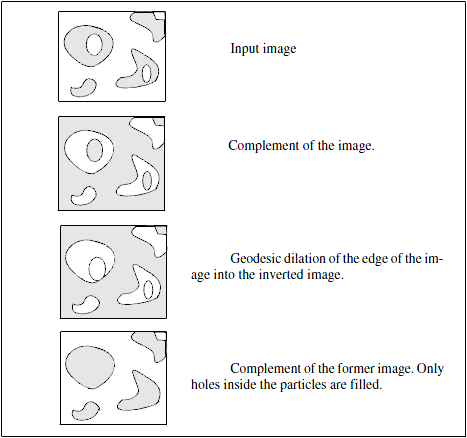
Figure 1. Illustration of the hole filling algorithm
Note: Since the reconstruction is applied on the input image complement, selecting a 6-neighbor connectivity fills holes connected to the background with a 26-neighbor connectivity.
See also
Access to parameter description
For an introduction:
- section Mathematical Morphology
- section Reconstruction From Markers
Holes inside objects are filled using a numerical reconstruction applied on the complement of the input image, from markers made of voxels of the image frame.
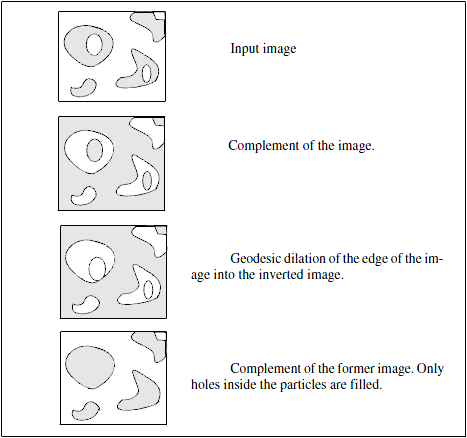
Figure 1. Illustration of the hole filling algorithm
Note: Since the reconstruction is applied on the input image complement, selecting a 6-neighbor connectivity fills holes connected to the background with a 26-neighbor connectivity.
See also
Function Syntax
This function returns outputObjectImage.
// Function prototype
std::shared_ptr< iolink::ImageView > fillHoles3d( std::shared_ptr< iolink::ImageView > inputObjectImage, FillHoles3d::Neighborhood neighborhood, std::shared_ptr< iolink::ImageView > outputObjectImage = nullptr );
This function returns outputObjectImage.
// Function prototype. fill_holes_3d(input_object_image: idt.ImageType, neighborhood: FillHoles3d.Neighborhood = FillHoles3d.Neighborhood.CONNECTIVITY_26, output_object_image: idt.ImageType = None) -> idt.ImageType
This function returns outputObjectImage.
// Function prototype. public static IOLink.ImageView FillHoles3d( IOLink.ImageView inputObjectImage, FillHoles3d.Neighborhood neighborhood = ImageDev.FillHoles3d.Neighborhood.CONNECTIVITY_26, IOLink.ImageView outputObjectImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||||
---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
inputObjectImage |
The 3D binary input image. | Image | Binary or Label | nullptr | ||||||
![]() |
neighborhood |
The 3D neighborhood configuration.
|
Enumeration | CONNECTIVITY_26 | |||||||
![]() |
outputObjectImage |
The binary output image. Its dimensions are forced to the same values as the input. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |||||||
---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
input_object_image |
The 3D binary input image. | image | Binary or Label | None | ||||||
![]() |
neighborhood |
The 3D neighborhood configuration.
|
enumeration | CONNECTIVITY_26 | |||||||
![]() |
output_object_image |
The binary output image. Its dimensions are forced to the same values as the input. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |||||||
---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
inputObjectImage |
The 3D binary input image. | Image | Binary or Label | null | ||||||
![]() |
neighborhood |
The 3D neighborhood configuration.
|
Enumeration | CONNECTIVITY_26 | |||||||
![]() |
outputObjectImage |
The binary output image. Its dimensions are forced to the same values as the input. | Image | null |
Object Examples
auto foam_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam_sep.vip" ); FillHoles3d fillHoles3dAlgo; fillHoles3dAlgo.setInputObjectImage( foam_sep ); fillHoles3dAlgo.setNeighborhood( FillHoles3d::Neighborhood::CONNECTIVITY_26 ); fillHoles3dAlgo.execute(); std::cout << "outputObjectImage:" << fillHoles3dAlgo.outputObjectImage()->toString();
foam_sep = imagedev.read_vip_image(imagedev_data.get_image_path("foam_sep.vip")) fill_holes_3d_algo = imagedev.FillHoles3d() fill_holes_3d_algo.input_object_image = foam_sep fill_holes_3d_algo.neighborhood = imagedev.FillHoles3d.CONNECTIVITY_26 fill_holes_3d_algo.execute() print("output_object_image:", str(fill_holes_3d_algo.output_object_image))
ImageView foam_sep = Data.ReadVipImage( @"Data/images/foam_sep.vip" ); FillHoles3d fillHoles3dAlgo = new FillHoles3d { inputObjectImage = foam_sep, neighborhood = FillHoles3d.Neighborhood.CONNECTIVITY_26 }; fillHoles3dAlgo.Execute(); Console.WriteLine( "outputObjectImage:" + fillHoles3dAlgo.outputObjectImage.ToString() );
Function Examples
auto foam_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam_sep.vip" ); auto result = fillHoles3d( foam_sep, FillHoles3d::Neighborhood::CONNECTIVITY_26 ); std::cout << "outputObjectImage:" << result->toString();
foam_sep = imagedev.read_vip_image(imagedev_data.get_image_path("foam_sep.vip")) result = imagedev.fill_holes_3d(foam_sep, imagedev.FillHoles3d.CONNECTIVITY_26) print("output_object_image:", str(result))
ImageView foam_sep = Data.ReadVipImage( @"Data/images/foam_sep.vip" ); IOLink.ImageView result = Processing.FillHoles3d( foam_sep, FillHoles3d.Neighborhood.CONNECTIVITY_26 ); Console.WriteLine( "outputObjectImage:" + result.ToString() );