RegionalExtrema3d
Computes the regional maxima or minima of a three-dimensional grayscale image and marks them in a binary image.
Access to parameter description
For an introduction:
A regional maximum (resp. minimum) $C$ is a set of connected pixels such that:
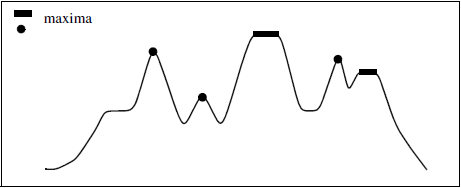
Figure 1. One-dimensional example of a regional maxima detection
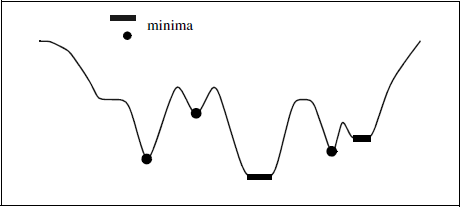
Figure 2. One-dimensional example of a regional minima detection
This algorithm uses a recursive method combined with a geodesic propagation.
To avoid getting too many regions in the output image, the input should be smoothed first with a low-pass filter or with the numerical reconstruction algorithm.
Reference:
P. Soille, Morphological Image Analysis. Principles and Applications, Second Edition, Springer-Verlag, Berlin, pp.201-203, 2003.
See also
Access to parameter description
For an introduction:
- section Mathematical Morphology
- section Geodesic Transformations
A regional maximum (resp. minimum) $C$ is a set of connected pixels such that:
- Pixels belonging to $C$ have the same intensity $I_C$.
- Pixels connected to $C$, but not belonging to $C$ (neighbors), have an intensity strictly lower (resp. greater) than $I_C$.
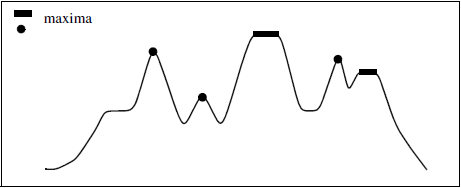
Figure 1. One-dimensional example of a regional maxima detection
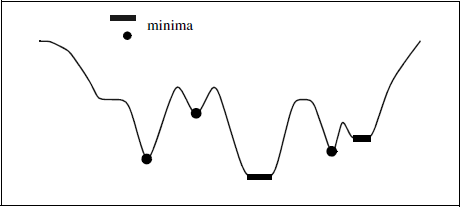
Figure 2. One-dimensional example of a regional minima detection
This algorithm uses a recursive method combined with a geodesic propagation.
To avoid getting too many regions in the output image, the input should be smoothed first with a low-pass filter or with the numerical reconstruction algorithm.
Reference:
P. Soille, Morphological Image Analysis. Principles and Applications, Second Edition, Springer-Verlag, Berlin, pp.201-203, 2003.
See also
Function Syntax
This function returns outputBinaryImage.
// Function prototype
std::shared_ptr< iolink::ImageView > regionalExtrema3d( std::shared_ptr< iolink::ImageView > inputImage, RegionalExtrema3d::ExtremaType extremaType, RegionalExtrema3d::Neighborhood neighborhood, std::shared_ptr< iolink::ImageView > outputBinaryImage = nullptr );
This function returns outputBinaryImage.
// Function prototype. regional_extrema_3d(input_image: idt.ImageType, extrema_type: RegionalExtrema3d.ExtremaType = RegionalExtrema3d.ExtremaType.MAXIMA, neighborhood: RegionalExtrema3d.Neighborhood = RegionalExtrema3d.Neighborhood.CONNECTIVITY_26, output_binary_image: idt.ImageType = None) -> idt.ImageType
This function returns outputBinaryImage.
// Function prototype. public static IOLink.ImageView RegionalExtrema3d( IOLink.ImageView inputImage, RegionalExtrema3d.ExtremaType extremaType = ImageDev.RegionalExtrema3d.ExtremaType.MAXIMA, RegionalExtrema3d.Neighborhood neighborhood = ImageDev.RegionalExtrema3d.Neighborhood.CONNECTIVITY_26, IOLink.ImageView outputBinaryImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||||
---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input grayscale image. | Image | Grayscale | nullptr | ||||||
![]() |
extremaType |
The type of extrema to detect.
|
Enumeration | MAXIMA | |||||||
![]() |
neighborhood |
The 3D neighborhood configuration.
|
Enumeration | CONNECTIVITY_26 | |||||||
![]() |
outputBinaryImage |
The output binary image. Its dimensions are forced to the same values as the input. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |||||||
---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
input_image |
The input grayscale image. | image | Grayscale | None | ||||||
![]() |
extrema_type |
The type of extrema to detect.
|
enumeration | MAXIMA | |||||||
![]() |
neighborhood |
The 3D neighborhood configuration.
|
enumeration | CONNECTIVITY_26 | |||||||
![]() |
output_binary_image |
The output binary image. Its dimensions are forced to the same values as the input. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |||||||
---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input grayscale image. | Image | Grayscale | null | ||||||
![]() |
extremaType |
The type of extrema to detect.
|
Enumeration | MAXIMA | |||||||
![]() |
neighborhood |
The 3D neighborhood configuration.
|
Enumeration | CONNECTIVITY_26 | |||||||
![]() |
outputBinaryImage |
The output binary image. Its dimensions are forced to the same values as the input. | Image | null |
Object Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); RegionalExtrema3d regionalExtrema3dAlgo; regionalExtrema3dAlgo.setInputImage( foam ); regionalExtrema3dAlgo.setExtremaType( RegionalExtrema3d::ExtremaType::MAXIMA ); regionalExtrema3dAlgo.setNeighborhood( RegionalExtrema3d::Neighborhood::CONNECTIVITY_26 ); regionalExtrema3dAlgo.execute(); std::cout << "outputBinaryImage:" << regionalExtrema3dAlgo.outputBinaryImage()->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) regional_extrema_3d_algo = imagedev.RegionalExtrema3d() regional_extrema_3d_algo.input_image = foam regional_extrema_3d_algo.extrema_type = imagedev.RegionalExtrema3d.MAXIMA regional_extrema_3d_algo.neighborhood = imagedev.RegionalExtrema3d.CONNECTIVITY_26 regional_extrema_3d_algo.execute() print("output_binary_image:", str(regional_extrema_3d_algo.output_binary_image))
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); RegionalExtrema3d regionalExtrema3dAlgo = new RegionalExtrema3d { inputImage = foam, extremaType = RegionalExtrema3d.ExtremaType.MAXIMA, neighborhood = RegionalExtrema3d.Neighborhood.CONNECTIVITY_26 }; regionalExtrema3dAlgo.Execute(); Console.WriteLine( "outputBinaryImage:" + regionalExtrema3dAlgo.outputBinaryImage.ToString() );
Function Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto result = regionalExtrema3d( foam, RegionalExtrema3d::ExtremaType::MAXIMA, RegionalExtrema3d::Neighborhood::CONNECTIVITY_26 ); std::cout << "outputBinaryImage:" << result->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) result = imagedev.regional_extrema_3d(foam, imagedev.RegionalExtrema3d.MAXIMA, imagedev.RegionalExtrema3d.CONNECTIVITY_26) print("output_binary_image:", str(result))
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); IOLink.ImageView result = Processing.RegionalExtrema3d( foam, RegionalExtrema3d.ExtremaType.MAXIMA, RegionalExtrema3d.Neighborhood.CONNECTIVITY_26 ); Console.WriteLine( "outputBinaryImage:" + result.ToString() );