MeasurementToImage
Replaces all labels of the input image by the result of an individual measurement.
Access to parameter description
For an introduction, see:
This algorithm replaces all labels of an input image by the result of an individual measurement. It supports the same individual measurements as any analysis algorithm (LabelAnalysis).
The data type of the output image depends on the selected measurement. It can be 32-bit floating point or integer.
For example, this algorithm can assign the surface value of a given object to all pixels that belong to it.
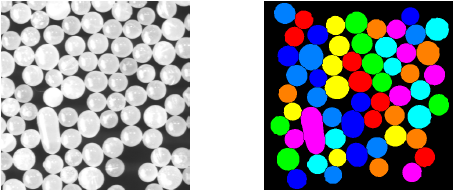
Figure 1 Grayscale and label input images
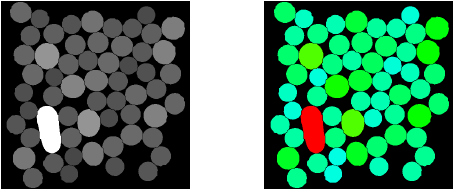
Figure 2 Result of MeasurementToImage command with Area2d as parameter (Left: standard grayscale colormap; Right: rainbow colormap)
See also
Access to parameter description
For an introduction, see:
- section Image Analysis
- Analysis measurements
This algorithm replaces all labels of an input image by the result of an individual measurement. It supports the same individual measurements as any analysis algorithm (LabelAnalysis).
The data type of the output image depends on the selected measurement. It can be 32-bit floating point or integer.
For example, this algorithm can assign the surface value of a given object to all pixels that belong to it.
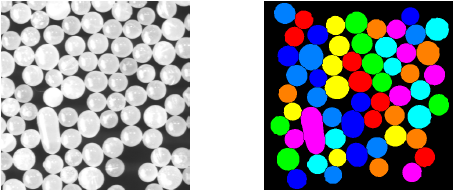
Figure 1 Grayscale and label input images
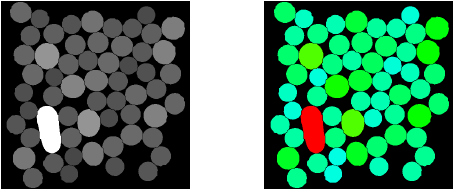
Figure 2 Result of MeasurementToImage command with Area2d as parameter (Left: standard grayscale colormap; Right: rainbow colormap)
See also
Function Syntax
This function returns outputImage.
// Function prototype
std::shared_ptr< iolink::ImageView > measurementToImage( std::shared_ptr< iolink::ImageView > inputLabelImage, std::shared_ptr< iolink::ImageView > inputGrayImage, std::string measurement, std::shared_ptr< iolink::ImageView > outputImage = nullptr );
This function returns outputImage.
// Function prototype. measurement_to_image(input_label_image: idt.ImageType, input_gray_image: idt.ImageType, measurement: str = "", output_image: idt.ImageType = None) -> idt.ImageType
This function returns outputImage.
// Function prototype. public static IOLink.ImageView MeasurementToImage( IOLink.ImageView inputLabelImage, IOLink.ImageView inputGrayImage, string measurement = "", IOLink.ImageView outputImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputLabelImage |
The input label image. | Image | Label | nullptr |
![]() |
inputGrayImage |
The input grayscale image that contains the intensity information. It must have the same dimensions as the label input image. It is only used with measurements requiring an intensity image, and is ignored with other measurements. If it equals null, the label input image will be used as the intensity input image. | Image | Binary, Label or Grayscale | nullptr |
![]() |
measurement |
The measurement defining the output values. | Measurement | "" | |
![]() |
outputImage |
The output image. Its dimensions are forced to the same values as the input. Its data type is forced to 32-bit floating point or integer, depending on the selected measurement. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
input_label_image |
The input label image. | image | Label | None |
![]() |
input_gray_image |
The input grayscale image that contains the intensity information. It must have the same dimensions as the label input image. It is only used with measurements requiring an intensity image, and is ignored with other measurements. If it equals null, the label input image will be used as the intensity input image. | image | Binary, Label or Grayscale | None |
![]() |
measurement |
The measurement defining the output values. | measurement | "" | |
![]() |
output_image |
The output image. Its dimensions are forced to the same values as the input. Its data type is forced to 32-bit floating point or integer, depending on the selected measurement. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputLabelImage |
The input label image. | Image | Label | null |
![]() |
inputGrayImage |
The input grayscale image that contains the intensity information. It must have the same dimensions as the label input image. It is only used with measurements requiring an intensity image, and is ignored with other measurements. If it equals null, the label input image will be used as the intensity input image. | Image | Binary, Label or Grayscale | null |
![]() |
measurement |
The measurement defining the output values. | Measurement | "" | |
![]() |
outputImage |
The output image. Its dimensions are forced to the same values as the input. Its data type is forced to 32-bit floating point or integer, depending on the selected measurement. | Image | null |
Object Examples
auto polystyrene_sep_label = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep_label.vip" ); auto polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); MeasurementToImage measurementToImageAlgo; measurementToImageAlgo.setInputLabelImage( polystyrene_sep_label ); measurementToImageAlgo.setInputGrayImage( polystyrene ); measurementToImageAlgo.setMeasurement( "Area2d" ); measurementToImageAlgo.execute(); std::cout << "outputImage:" << measurementToImageAlgo.outputImage()->toString();
polystyrene_sep_label = imagedev.read_vip_image(imagedev_data.get_image_path("polystyrene_sep_label.vip")) polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) measurement_to_image_algo = imagedev.MeasurementToImage() measurement_to_image_algo.input_label_image = polystyrene_sep_label measurement_to_image_algo.input_gray_image = polystyrene measurement_to_image_algo.measurement = "Area2d" measurement_to_image_algo.execute() print("output_image:", str(measurement_to_image_algo.output_image))
ImageView polystyrene_sep_label = Data.ReadVipImage( @"Data/images/polystyrene_sep_label.vip" ); ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); MeasurementToImage measurementToImageAlgo = new MeasurementToImage { inputLabelImage = polystyrene_sep_label, inputGrayImage = polystyrene, measurement = "Area2d" }; measurementToImageAlgo.Execute(); Console.WriteLine( "outputImage:" + measurementToImageAlgo.outputImage.ToString() );
Function Examples
auto polystyrene_sep_label = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep_label.vip" ); auto polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); auto result = measurementToImage( polystyrene_sep_label, polystyrene, "Area2d" ); std::cout << "outputImage:" << result->toString();
polystyrene_sep_label = imagedev.read_vip_image(imagedev_data.get_image_path("polystyrene_sep_label.vip")) polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) result = imagedev.measurement_to_image(polystyrene_sep_label, polystyrene, "Area2d") print("output_image:", str(result))
ImageView polystyrene_sep_label = Data.ReadVipImage( @"Data/images/polystyrene_sep_label.vip" ); ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); IOLink.ImageView result = Processing.MeasurementToImage( polystyrene_sep_label, polystyrene, "Area2d" ); Console.WriteLine( "outputImage:" + result.ToString() );