UltimateErosion
Computes the ultimate eroded set of a binary image.
Access to parameter description
For an introduction:
Then each erosion step is reconstructed into the previous erosion step ($Y_i$ image below).
The difference between the eroded image $X_i$ and the reconstructed image $Y_i$ is the centroid of objects that disappeared at the erosion step number $i$.
This algorithm enables counting the number of convex particles, even if some of them are touching each other, as long as the pseudo-centre of each particle is outside of other particles.
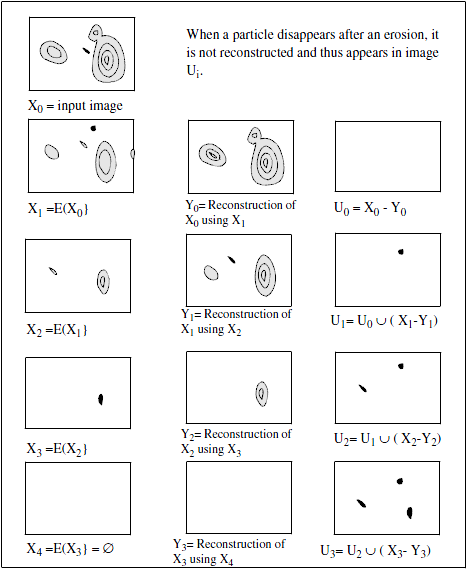
Figure 1. Ultimate erosion on a basic example
See also
Access to parameter description
For an introduction:
- section Mathematical Morphology
- section Geodesic Transformations
Then each erosion step is reconstructed into the previous erosion step ($Y_i$ image below).
The difference between the eroded image $X_i$ and the reconstructed image $Y_i$ is the centroid of objects that disappeared at the erosion step number $i$.
This algorithm enables counting the number of convex particles, even if some of them are touching each other, as long as the pseudo-centre of each particle is outside of other particles.
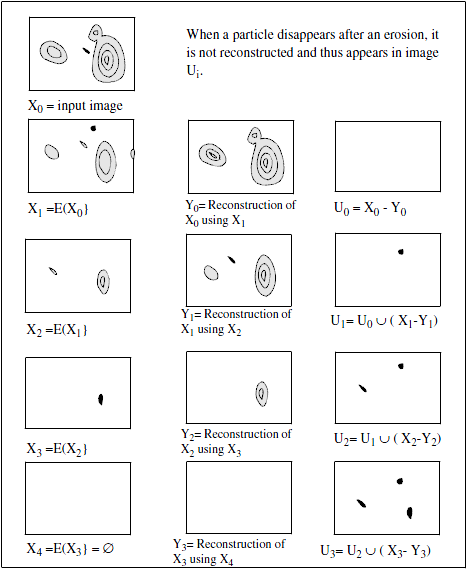
Figure 1. Ultimate erosion on a basic example
See also
Function Syntax
This function returns outputBinaryImage.
// Function prototype
std::shared_ptr< iolink::ImageView > ultimateErosion( std::shared_ptr< iolink::ImageView > inputBinaryImage, UltimateErosion::Neighborhood neighborhood, std::shared_ptr< iolink::ImageView > outputBinaryImage = NULL );
This function returns outputBinaryImage.
// Function prototype. ultimate_erosion( input_binary_image, neighborhood = UltimateErosion.Neighborhood.CONNECTIVITY_26, output_binary_image = None )
This function returns outputBinaryImage.
// Function prototype. public static IOLink.ImageView UltimateErosion( IOLink.ImageView inputBinaryImage, UltimateErosion.Neighborhood neighborhood = ImageDev.UltimateErosion.Neighborhood.CONNECTIVITY_26, IOLink.ImageView outputBinaryImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||||
---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
inputBinaryImage |
The binary input image. | Image | Binary | nullptr | ||||||
![]() |
neighborhood |
The 3D neighborhood configuration. This parameter is ignored with a 2D input image.
|
Enumeration | CONNECTIVITY_26 | |||||||
![]() |
outputBinaryImage |
The binary output image. Its dimensions and type are forced to the same values as the input. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |||||||
---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
input_binary_image |
The binary input image. | image | Binary | None | ||||||
![]() |
neighborhood |
The 3D neighborhood configuration. This parameter is ignored with a 2D input image.
|
enumeration | CONNECTIVITY_26 | |||||||
![]() |
output_binary_image |
The binary output image. Its dimensions and type are forced to the same values as the input. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |||||||
---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
inputBinaryImage |
The binary input image. | Image | Binary | null | ||||||
![]() |
neighborhood |
The 3D neighborhood configuration. This parameter is ignored with a 2D input image.
|
Enumeration | CONNECTIVITY_26 | |||||||
![]() |
outputBinaryImage |
The binary output image. Its dimensions and type are forced to the same values as the input. | Image | null |
Object Examples
auto foam_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam_sep.vip" ); UltimateErosion ultimateErosionAlgo; ultimateErosionAlgo.setInputBinaryImage( foam_sep ); ultimateErosionAlgo.setNeighborhood( UltimateErosion::Neighborhood::CONNECTIVITY_26 ); ultimateErosionAlgo.execute(); std::cout << "outputBinaryImage:" << ultimateErosionAlgo.outputBinaryImage()->toString();
foam_sep = imagedev.read_vip_image(imagedev_data.get_image_path("foam_sep.vip")) ultimate_erosion_algo = imagedev.UltimateErosion() ultimate_erosion_algo.input_binary_image = foam_sep ultimate_erosion_algo.neighborhood = imagedev.UltimateErosion.CONNECTIVITY_26 ultimate_erosion_algo.execute() print( "output_binary_image:", str( ultimate_erosion_algo.output_binary_image ) )
ImageView foam_sep = Data.ReadVipImage( @"Data/images/foam_sep.vip" ); UltimateErosion ultimateErosionAlgo = new UltimateErosion { inputBinaryImage = foam_sep, neighborhood = UltimateErosion.Neighborhood.CONNECTIVITY_26 }; ultimateErosionAlgo.Execute(); Console.WriteLine( "outputBinaryImage:" + ultimateErosionAlgo.outputBinaryImage.ToString() );
Function Examples
auto foam_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam_sep.vip" ); auto result = ultimateErosion( foam_sep, UltimateErosion::Neighborhood::CONNECTIVITY_26 ); std::cout << "outputBinaryImage:" << result->toString();
foam_sep = imagedev.read_vip_image(imagedev_data.get_image_path("foam_sep.vip")) result = imagedev.ultimate_erosion( foam_sep, imagedev.UltimateErosion.CONNECTIVITY_26 ) print( "output_binary_image:", str( result ) )
ImageView foam_sep = Data.ReadVipImage( @"Data/images/foam_sep.vip" ); IOLink.ImageView result = Processing.UltimateErosion( foam_sep, UltimateErosion.Neighborhood.CONNECTIVITY_26 ); Console.WriteLine( "outputBinaryImage:" + result.ToString() );