ReconstructionFromMarkers3d
ReconstructionFromMarkers3d rebuilds a binary image starting from markers.
Access to parameter description
For an introduction:
Successive dilations are performed on the markers, each dilation being followed by an intersection with the original image until convergence (idempotence).
This iterative process is called a geodesic dilation.
It guarantees that a dilated marker will not merge with a particle other than the one it marks.
Geodesic dilation is different from a large dilation of a given size followed by an intersection with the original image because a dilated marker may hit close particles that are not marked for reconstruction. This difference is illustrated in Figure 1.
For example, given an erosion that eliminated the small particles of an image, this algorithm can rebuild the original shapes of the remaining particles if the eroded set is used as the markers. This sequence is called an opening by reconstruction.
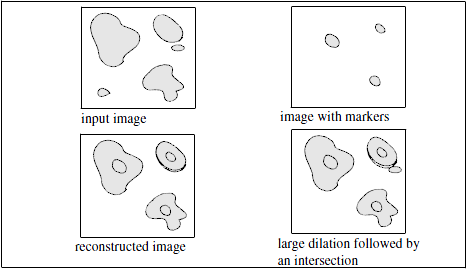
Figure 1. Comparison between reconstruction from markers and dilation/intersection
See also
Access to parameter description
For an introduction:
- section Mathematical Morphology
- section Geodesic Transformations
Successive dilations are performed on the markers, each dilation being followed by an intersection with the original image until convergence (idempotence).
This iterative process is called a geodesic dilation.
It guarantees that a dilated marker will not merge with a particle other than the one it marks.
Geodesic dilation is different from a large dilation of a given size followed by an intersection with the original image because a dilated marker may hit close particles that are not marked for reconstruction. This difference is illustrated in Figure 1.
For example, given an erosion that eliminated the small particles of an image, this algorithm can rebuild the original shapes of the remaining particles if the eroded set is used as the markers. This sequence is called an opening by reconstruction.
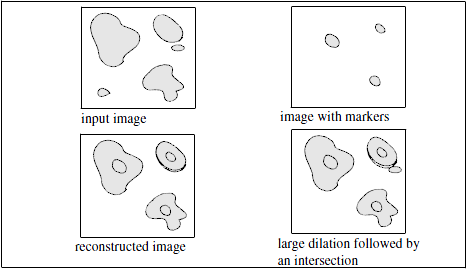
Figure 1. Comparison between reconstruction from markers and dilation/intersection
See also
Function Syntax
This function returns outputImage.
// Function prototype
std::shared_ptr< iolink::ImageView > reconstructionFromMarkers3d( std::shared_ptr< iolink::ImageView > inputImage, std::shared_ptr< iolink::ImageView > inputMarkerImage, ReconstructionFromMarkers3d::Neighborhood neighborhood, std::shared_ptr< iolink::ImageView > outputImage = NULL );
This function returns outputImage.
// Function prototype. reconstruction_from_markers_3d( input_image, input_marker_image, neighborhood = ReconstructionFromMarkers3d.Neighborhood.CONNECTIVITY_26, output_image = None )
This function returns outputImage.
// Function prototype. public static IOLink.ImageView ReconstructionFromMarkers3d( IOLink.ImageView inputImage, IOLink.ImageView inputMarkerImage, ReconstructionFromMarkers3d.Neighborhood neighborhood = ImageDev.ReconstructionFromMarkers3d.Neighborhood.CONNECTIVITY_26, IOLink.ImageView outputImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||||
---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The binary mask image constraining reconstruction. | Image | Binary | nullptr | ||||||
![]() |
inputMarkerImage |
The binary marker image containing seeds for reconstruction. It must have the same dimensions and type as the input image. | Image | Binary | nullptr | ||||||
![]() |
neighborhood |
The 3D neighborhood configuration.
|
Enumeration | CONNECTIVITY_26 | |||||||
![]() |
outputImage |
The binary output image. Its dimensions and type are forced to the same values as the input. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |||||||
---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
input_image |
The binary mask image constraining reconstruction. | image | Binary | None | ||||||
![]() |
input_marker_image |
The binary marker image containing seeds for reconstruction. It must have the same dimensions and type as the input image. | image | Binary | None | ||||||
![]() |
neighborhood |
The 3D neighborhood configuration.
|
enumeration | CONNECTIVITY_26 | |||||||
![]() |
output_image |
The binary output image. Its dimensions and type are forced to the same values as the input. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |||||||
---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The binary mask image constraining reconstruction. | Image | Binary | null | ||||||
![]() |
inputMarkerImage |
The binary marker image containing seeds for reconstruction. It must have the same dimensions and type as the input image. | Image | Binary | null | ||||||
![]() |
neighborhood |
The 3D neighborhood configuration.
|
Enumeration | CONNECTIVITY_26 | |||||||
![]() |
outputImage |
The binary output image. Its dimensions and type are forced to the same values as the input. | Image | null |
Object Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); ReconstructionFromMarkers3d reconstructionFromMarkers3dAlgo; reconstructionFromMarkers3dAlgo.setInputImage( polystyrene_sep ); reconstructionFromMarkers3dAlgo.setInputMarkerImage( polystyrene_sep ); reconstructionFromMarkers3dAlgo.setNeighborhood( ReconstructionFromMarkers3d::Neighborhood::CONNECTIVITY_26 ); reconstructionFromMarkers3dAlgo.execute(); std::cout << "outputImage:" << reconstructionFromMarkers3dAlgo.outputImage()->toString();
polystyrene_sep = imagedev.read_vip_image(imagedev_data.get_image_path("polystyrene_sep.vip")) reconstruction_from_markers_3d_algo = imagedev.ReconstructionFromMarkers3d() reconstruction_from_markers_3d_algo.input_image = polystyrene_sep reconstruction_from_markers_3d_algo.input_marker_image = polystyrene_sep reconstruction_from_markers_3d_algo.neighborhood = imagedev.ReconstructionFromMarkers3d.CONNECTIVITY_26 reconstruction_from_markers_3d_algo.execute() print( "output_image:", str( reconstruction_from_markers_3d_algo.output_image ) )
ImageView polystyrene_sep = Data.ReadVipImage( @"Data/images/polystyrene_sep.vip" ); ReconstructionFromMarkers3d reconstructionFromMarkers3dAlgo = new ReconstructionFromMarkers3d { inputImage = polystyrene_sep, inputMarkerImage = polystyrene_sep, neighborhood = ReconstructionFromMarkers3d.Neighborhood.CONNECTIVITY_26 }; reconstructionFromMarkers3dAlgo.Execute(); Console.WriteLine( "outputImage:" + reconstructionFromMarkers3dAlgo.outputImage.ToString() );
Function Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); auto result = reconstructionFromMarkers3d( polystyrene_sep, polystyrene_sep, ReconstructionFromMarkers3d::Neighborhood::CONNECTIVITY_26 ); std::cout << "outputImage:" << result->toString();
polystyrene_sep = imagedev.read_vip_image(imagedev_data.get_image_path("polystyrene_sep.vip")) result = imagedev.reconstruction_from_markers_3d( polystyrene_sep, polystyrene_sep, imagedev.ReconstructionFromMarkers3d.CONNECTIVITY_26 ) print( "output_image:", str( result ) )
ImageView polystyrene_sep = Data.ReadVipImage( @"Data/images/polystyrene_sep.vip" ); IOLink.ImageView result = Processing.ReconstructionFromMarkers3d( polystyrene_sep, polystyrene_sep, ReconstructionFromMarkers3d.Neighborhood.CONNECTIVITY_26 ); Console.WriteLine( "outputImage:" + result.ToString() );