HistogramEqualization
Enhances the contrast of an image by performing an histogram equalization.
Access to parameter description
This algorithm performs an histogram equalization of image $I$ onto image $O$. For example, if an image contains $N$ pixels and uses $M$ gray values, there is an average of $N/M$ pixels per gray level. An image is correctly contrasted if the number of pixels is close to this mean value for each gray level value.
The aim of histogram equalization is to transform the histogram to make it uniform. In Figure 1-a, the histogram is very condensed, and the image is poorly contrasted.
In Figure 1-b, the histogram is more widely distributed, and the image has better contrast.
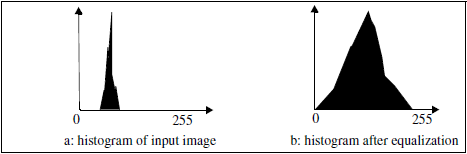
Figure 1. Histogram equalization
See also
Access to parameter description
This algorithm performs an histogram equalization of image $I$ onto image $O$. For example, if an image contains $N$ pixels and uses $M$ gray values, there is an average of $N/M$ pixels per gray level. An image is correctly contrasted if the number of pixels is close to this mean value for each gray level value.
The aim of histogram equalization is to transform the histogram to make it uniform. In Figure 1-a, the histogram is very condensed, and the image is poorly contrasted.
In Figure 1-b, the histogram is more widely distributed, and the image has better contrast.
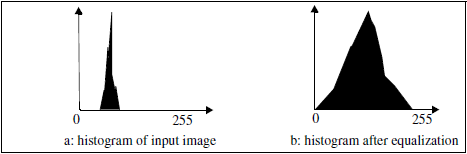
Figure 1. Histogram equalization
See also
Function Syntax
This function returns outputImage.
// Function prototype
std::shared_ptr< iolink::ImageView > histogramEqualization( std::shared_ptr< iolink::ImageView > inputImage, HistogramEqualization::RangeMode rangeMode, iolink::Vector2i32 intensityInputRange, iolink::Vector2i32 intensityOutputRange, std::shared_ptr< iolink::ImageView > outputImage = NULL );
This function returns outputImage.
// Function prototype. histogram_equalization( input_image, range_mode = HistogramEqualization.RangeMode.MIN_MAX, intensity_input_range = [0, 255], intensity_output_range = [0, 255], output_image = None )
This function returns outputImage.
// Function prototype. public static IOLink.ImageView HistogramEqualization( IOLink.ImageView inputImage, HistogramEqualization.RangeMode rangeMode = ImageDev.HistogramEqualization.RangeMode.MIN_MAX, int[] intensityInputRange = null, int[] intensityOutputRange = null, IOLink.ImageView outputImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input image to enhance. | Image | Binary, Label or Grayscale | nullptr | ||||
![]() |
rangeMode |
The way to define the input intensity range.
|
Enumeration | MIN_MAX | |||||
![]() |
intensityInputRange |
The input intensity range [a, b] to use when the range mode is set to OTHER. This parameter is ignored with the MIN_MAX range mode. | Vector2i32 | >=0 | {0, 255} | ||||
![]() |
intensityOutputRange |
The output intensity range. | Vector2i32 | >=0 | {0, 255} | ||||
![]() |
outputImage |
The output image. Its dimensions and type are forced to the same values as the input. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
input_image |
The input image to enhance. | image | Binary, Label or Grayscale | None | ||||
![]() |
range_mode |
The way to define the input intensity range.
|
enumeration | MIN_MAX | |||||
![]() |
intensity_input_range |
The input intensity range [a, b] to use when the range mode is set to OTHER. This parameter is ignored with the MIN_MAX range mode. | vector2i32 | >=0 | [0, 255] | ||||
![]() |
intensity_output_range |
The output intensity range. | vector2i32 | >=0 | [0, 255] | ||||
![]() |
output_image |
The output image. Its dimensions and type are forced to the same values as the input. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input image to enhance. | Image | Binary, Label or Grayscale | null | ||||
![]() |
rangeMode |
The way to define the input intensity range.
|
Enumeration | MIN_MAX | |||||
![]() |
intensityInputRange |
The input intensity range [a, b] to use when the range mode is set to OTHER. This parameter is ignored with the MIN_MAX range mode. | Vector2i32 | >=0 | {0, 255} | ||||
![]() |
intensityOutputRange |
The output intensity range. | Vector2i32 | >=0 | {0, 255} | ||||
![]() |
outputImage |
The output image. Its dimensions and type are forced to the same values as the input. | Image | null |
Object Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); HistogramEqualization histogramEqualizationAlgo; histogramEqualizationAlgo.setInputImage( foam ); histogramEqualizationAlgo.setRangeMode( HistogramEqualization::RangeMode::MIN_MAX ); histogramEqualizationAlgo.setIntensityInputRange( {0, 255} ); histogramEqualizationAlgo.setIntensityOutputRange( {0, 255} ); histogramEqualizationAlgo.execute(); std::cout << "outputImage:" << histogramEqualizationAlgo.outputImage()->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) histogram_equalization_algo = imagedev.HistogramEqualization() histogram_equalization_algo.input_image = foam histogram_equalization_algo.range_mode = imagedev.HistogramEqualization.MIN_MAX histogram_equalization_algo.intensity_input_range = [0, 255] histogram_equalization_algo.intensity_output_range = [0, 255] histogram_equalization_algo.execute() print( "output_image:", str( histogram_equalization_algo.output_image ) )
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); HistogramEqualization histogramEqualizationAlgo = new HistogramEqualization { inputImage = foam, rangeMode = HistogramEqualization.RangeMode.MIN_MAX, intensityInputRange = new int[]{0, 255}, intensityOutputRange = new int[]{0, 255} }; histogramEqualizationAlgo.Execute(); Console.WriteLine( "outputImage:" + histogramEqualizationAlgo.outputImage.ToString() );
Function Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto result = histogramEqualization( foam, HistogramEqualization::RangeMode::MIN_MAX, {0, 255}, {0, 255} ); std::cout << "outputImage:" << result->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) result = imagedev.histogram_equalization( foam, imagedev.HistogramEqualization.MIN_MAX, [0, 255], [0, 255] ) print( "output_image:", str( result ) )
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); IOLink.ImageView result = Processing.HistogramEqualization( foam, HistogramEqualization.RangeMode.MIN_MAX, new int[]{0, 255}, new int[]{0, 255} ); Console.WriteLine( "outputImage:" + result.ToString() );