RandomSphereImage3d
Creates synthetic spheres in a binary image.
Access to parameter description
The RandomSphereImage3d algorithm creates a binary image containing a random distribution of spheres. The sphere radius range, distance, type of distribution, and number are parameters of the algorithm.
The following image is an example of the result with the default parameters:
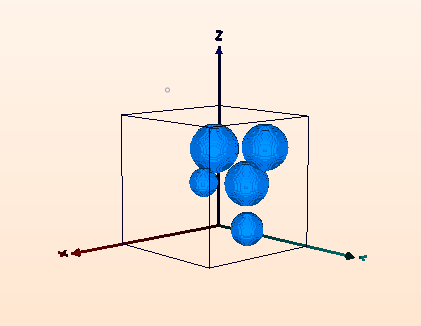
Figure 1. Generation of 5 spheres
See also
Access to parameter description
The RandomSphereImage3d algorithm creates a binary image containing a random distribution of spheres. The sphere radius range, distance, type of distribution, and number are parameters of the algorithm.
The following image is an example of the result with the default parameters:
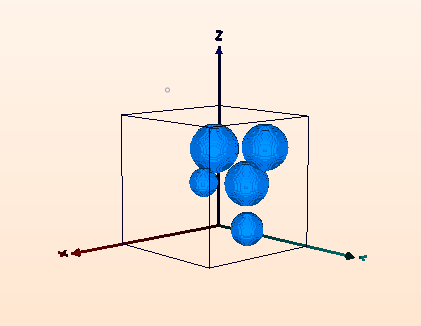
Figure 1. Generation of 5 spheres
See also
Function Syntax
This function returns outputBinaryImage.
// Function prototype
std::shared_ptr< iolink::ImageView > randomSphereImage3d( iolink::Vector3i32 imageSize, int32_t sphereNumber, RandomSphereImage3d::Distribution distribution, iolink::Vector2i32 radiusRange, int32_t distance, std::shared_ptr< iolink::ImageView > outputBinaryImage = NULL );
This function returns outputBinaryImage.
// Function prototype. random_sphere_image_3d( image_size = [100, 100, 100], sphere_number = 5, distribution = RandomSphereImage3d.Distribution.UNIFORM, radius_range = [10, 20], distance = 40, output_binary_image = None )
This function returns outputBinaryImage.
// Function prototype. public static IOLink.ImageView RandomSphereImage3d( int[] imageSize = null, Int32 sphereNumber = 5, RandomSphereImage3d.Distribution distribution = ImageDev.RandomSphereImage3d.Distribution.UNIFORM, int[] radiusRange = null, Int32 distance = 40, IOLink.ImageView outputBinaryImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
imageSize |
The X, Y, and Z size in voxels of the output image. | Vector3i32 | >0 | {100, 100, 100} | ||||
![]() |
sphereNumber |
The number of spheres to generate. | Int32 | >=1 | 5 | ||||
![]() |
distribution |
The distribution type of the sphere radii.
|
Enumeration | UNIFORM | |||||
![]() |
radiusRange |
The minimum and maximum radius in voxels of the spheres (for Uniform distribution) or mean and standard deviation (for gaussian distribution). | Vector2i32 | Any value | {10, 20} | ||||
![]() |
distance |
The minimum distance between two sphere centers. | Int32 | >=0 | 40 | ||||
![]() |
outputBinaryImage |
The output 3D binary image. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
image_size |
The X, Y, and Z size in voxels of the output image. | vector3i32 | >0 | [100, 100, 100] | ||||
![]() |
sphere_number |
The number of spheres to generate. | int32 | >=1 | 5 | ||||
![]() |
distribution |
The distribution type of the sphere radii.
|
enumeration | UNIFORM | |||||
![]() |
radius_range |
The minimum and maximum radius in voxels of the spheres (for Uniform distribution) or mean and standard deviation (for gaussian distribution). | vector2i32 | Any value | [10, 20] | ||||
![]() |
distance |
The minimum distance between two sphere centers. | int32 | >=0 | 40 | ||||
![]() |
output_binary_image |
The output 3D binary image. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
imageSize |
The X, Y, and Z size in voxels of the output image. | Vector3i32 | >0 | {100, 100, 100} | ||||
![]() |
sphereNumber |
The number of spheres to generate. | Int32 | >=1 | 5 | ||||
![]() |
distribution |
The distribution type of the sphere radii.
|
Enumeration | UNIFORM | |||||
![]() |
radiusRange |
The minimum and maximum radius in voxels of the spheres (for Uniform distribution) or mean and standard deviation (for gaussian distribution). | Vector2i32 | Any value | {10, 20} | ||||
![]() |
distance |
The minimum distance between two sphere centers. | Int32 | >=0 | 40 | ||||
![]() |
outputBinaryImage |
The output 3D binary image. | Image | null |
Object Examples
RandomSphereImage3d randomSphereImage3dAlgo; randomSphereImage3dAlgo.setImageSize( {100, 100, 100} ); randomSphereImage3dAlgo.setSphereNumber( 5 ); randomSphereImage3dAlgo.setDistribution( RandomSphereImage3d::Distribution::UNIFORM ); randomSphereImage3dAlgo.setRadiusRange( {10, 20} ); randomSphereImage3dAlgo.setDistance( 40 ); randomSphereImage3dAlgo.execute(); std::cout << "outputBinaryImage:" << randomSphereImage3dAlgo.outputBinaryImage()->toString();
random_sphere_image_3d_algo = imagedev.RandomSphereImage3d() random_sphere_image_3d_algo.image_size = [100, 100, 100] random_sphere_image_3d_algo.sphere_number = 5 random_sphere_image_3d_algo.distribution = imagedev.RandomSphereImage3d.UNIFORM random_sphere_image_3d_algo.radius_range = [10, 20] random_sphere_image_3d_algo.distance = 40 random_sphere_image_3d_algo.execute() print( "output_binary_image:", str( random_sphere_image_3d_algo.output_binary_image ) )
RandomSphereImage3d randomSphereImage3dAlgo = new RandomSphereImage3d { imageSize = new int[]{100, 100, 100}, sphereNumber = 5, distribution = RandomSphereImage3d.Distribution.UNIFORM, radiusRange = new int[]{10, 20}, distance = 40 }; randomSphereImage3dAlgo.Execute(); Console.WriteLine( "outputBinaryImage:" + randomSphereImage3dAlgo.outputBinaryImage.ToString() );
Function Examples
auto result = randomSphereImage3d( {100, 100, 100}, 5, RandomSphereImage3d::Distribution::UNIFORM, {10, 20}, 40 ); std::cout << "outputBinaryImage:" << result->toString();
result = imagedev.random_sphere_image_3d( [100, 100, 100], 5, imagedev.RandomSphereImage3d.UNIFORM, [10, 20], 40 ) print( "output_binary_image:", str( result ) )
IOLink.ImageView result = Processing.RandomSphereImage3d( new int[]{100, 100, 100}, 5, RandomSphereImage3d.Distribution.UNIFORM, new int[]{10, 20}, 40 ); Console.WriteLine( "outputBinaryImage:" + result.ToString() );