LocalMaxima3d
Extracts the local maxima of an image using a three-dimensional neighborhood analysis.
Access to parameter description
This algorithm considers $I(i,j,k)$ as a local maximum of $I$ if the two conditions below are verified
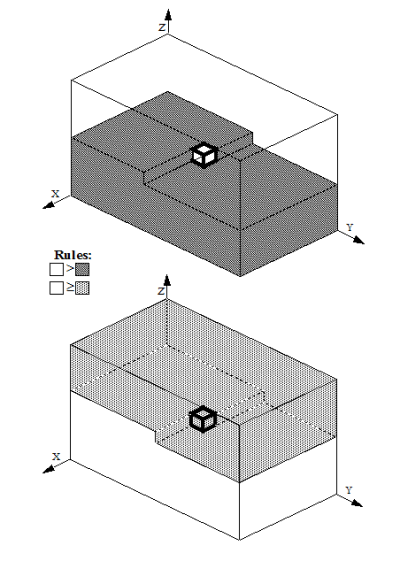
Figure 1. Graphic visualization of the 3D neighborhood analysis
See also
Access to parameter description
This algorithm considers $I(i,j,k)$ as a local maximum of $I$ if the two conditions below are verified
-
1. $I(i,j,k)$ is a local maximum in a $(2p+1)\times(2q+1)$\times(2r+1)$ neighborhood, i.e., considering
$l \in [1,p]$, $m \in [1,q]$, $n \in [1,r]$, s in [-p,p] and t in [-q,q], $I(i,j)$
- $I(i,j,k)>I(i+s,j+t,k-n)$
- $I(i,j,k)>I(i+s,j-m,k)$
- $I(i,j,k)>I(i-l,j,k)$
- $I(i,j,k) \geq I(i+s,j,k)$
- $I(i,j,k) \geq I(i+s,j+t,k+n)$
- $I(i,j,k)>threshold$
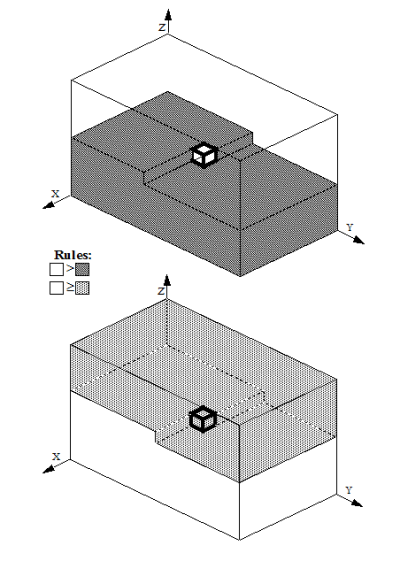
Figure 1. Graphic visualization of the 3D neighborhood analysis
See also
Function Syntax
This function returns outputMeasurement.
// Function prototype
LocalMaximaMsr::Ptr localMaxima3d( std::shared_ptr< iolink::ImageView > inputGrayImage, iolink::Vector3i32 localWindow, double thresholdRange, LocalMaxima3d::Precision precision, int32_t numberOfPatterns, LocalMaximaMsr::Ptr outputMeasurement = NULL );
This function returns outputMeasurement.
// Function prototype. local_maxima_3d( input_gray_image, local_window = [3, 3, 3], threshold_range = 0, precision = LocalMaxima3d.Precision.PIXEL, number_of_patterns = 1, output_measurement = None )
This function returns outputMeasurement.
// Function prototype. public static LocalMaximaMsr LocalMaxima3d( IOLink.ImageView inputGrayImage, int[] localWindow = null, double thresholdRange = 0, LocalMaxima3d.Precision precision = ImageDev.LocalMaxima3d.Precision.PIXEL, Int32 numberOfPatterns = 1, LocalMaximaMsr outputMeasurement = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputGrayImage |
The input grayscale image | Image | Binary, Label, Grayscale or Multispectral | nullptr | ||||
![]() |
localWindow |
The neighborhood size in pixels, along the X, Y, and Z directions. | Vector3i32 | >=0 | {3, 3, 3} | ||||
![]() |
thresholdRange |
The threshold value higher than which maxima are retained. | Float64 | >=0 | 0 | ||||
![]() |
precision |
The precision of the maxima localization.
|
Enumeration | PIXEL | |||||
![]() |
numberOfPatterns |
The maximum number of patterns to be detected. | Int32 | >=1 | 1 | ||||
![]() |
outputMeasurement |
The result object containing the maxima positions. | LocalMaximaMsr | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
input_gray_image |
The input grayscale image | image | Binary, Label, Grayscale or Multispectral | None | ||||
![]() |
local_window |
The neighborhood size in pixels, along the X, Y, and Z directions. | vector3i32 | >=0 | [3, 3, 3] | ||||
![]() |
threshold_range |
The threshold value higher than which maxima are retained. | float64 | >=0 | 0 | ||||
![]() |
precision |
The precision of the maxima localization.
|
enumeration | PIXEL | |||||
![]() |
number_of_patterns |
The maximum number of patterns to be detected. | int32 | >=1 | 1 | ||||
![]() |
output_measurement |
The result object containing the maxima positions. | LocalMaximaMsr | None |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputGrayImage |
The input grayscale image | Image | Binary, Label, Grayscale or Multispectral | null | ||||
![]() |
localWindow |
The neighborhood size in pixels, along the X, Y, and Z directions. | Vector3i32 | >=0 | {3, 3, 3} | ||||
![]() |
thresholdRange |
The threshold value higher than which maxima are retained. | Float64 | >=0 | 0 | ||||
![]() |
precision |
The precision of the maxima localization.
|
Enumeration | PIXEL | |||||
![]() |
numberOfPatterns |
The maximum number of patterns to be detected. | Int32 | >=1 | 1 | ||||
![]() |
outputMeasurement |
The result object containing the maxima positions. | LocalMaximaMsr | null |
Object Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); LocalMaxima3d localMaxima3dAlgo; localMaxima3dAlgo.setInputGrayImage( foam ); localMaxima3dAlgo.setLocalWindow( {3, 3, 3} ); localMaxima3dAlgo.setThresholdRange( 0 ); localMaxima3dAlgo.setPrecision( LocalMaxima3d::Precision::PIXEL ); localMaxima3dAlgo.setNumberOfPatterns( 1 ); localMaxima3dAlgo.execute(); std::cout << "value: " << localMaxima3dAlgo.outputMeasurement()->value( ) ;
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) local_maxima_3d_algo = imagedev.LocalMaxima3d() local_maxima_3d_algo.input_gray_image = foam local_maxima_3d_algo.local_window = [3, 3, 3] local_maxima_3d_algo.threshold_range = 0 local_maxima_3d_algo.precision = imagedev.LocalMaxima3d.PIXEL local_maxima_3d_algo.number_of_patterns = 1 local_maxima_3d_algo.execute() print( "value: ", str( local_maxima_3d_algo.output_measurement.value( ) ) )
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); LocalMaxima3d localMaxima3dAlgo = new LocalMaxima3d { inputGrayImage = foam, localWindow = new int[]{3, 3, 3}, thresholdRange = 0, precision = LocalMaxima3d.Precision.PIXEL, numberOfPatterns = 1 }; localMaxima3dAlgo.Execute(); Console.WriteLine( "value: " + localMaxima3dAlgo.outputMeasurement.value( ) );
Function Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto result = localMaxima3d( foam, {3, 3, 3}, 0, LocalMaxima3d::Precision::PIXEL, 1 ); std::cout << "value: " << result->value( ) ;
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) result = imagedev.local_maxima_3d( foam, [3, 3, 3], 0, imagedev.LocalMaxima3d.PIXEL, 1 ) print( "value: ", str( result.value( ) ) )
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); LocalMaximaMsr result = Processing.LocalMaxima3d( foam, new int[]{3, 3, 3}, 0, LocalMaxima3d.Precision.PIXEL, 1 ); Console.WriteLine( "value: " + result.value( ) );