FlowInpainting
Reconstructs missing areas of an image with an inpainting algorithm.
Access to parameter description
The FlowInpainting algorithm performs inpainting operations in an image in order to reconstuct some missing or corrupted data, designated by a binary mask. It consists of locally extrapolating the searched data in the input image.
These techniques can be used, for example, for reducing strong ring artifacts in computed tomograpy images.
Figure 1. Inpainting inputs: (left) input image, (right) binary mask
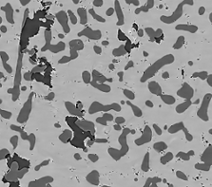
Figure 2. Inpainting result image
Reference:
D.Tschumperle, R.Deriche. "Vector-Valued Image Regularization with PDE's: A Common Framework for Different Applications", Rapport de recherche no 4657, INRIA Sophia-Antipolis, Dec. 2002.
See also
Access to parameter description
The FlowInpainting algorithm performs inpainting operations in an image in order to reconstuct some missing or corrupted data, designated by a binary mask. It consists of locally extrapolating the searched data in the input image.
These techniques can be used, for example, for reducing strong ring artifacts in computed tomograpy images.
![]() |
![]() |
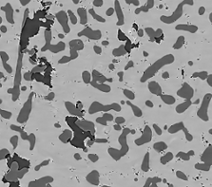
Figure 2. Inpainting result image
Reference:
D.Tschumperle, R.Deriche. "Vector-Valued Image Regularization with PDE's: A Common Framework for Different Applications", Rapport de recherche no 4657, INRIA Sophia-Antipolis, Dec. 2002.
See also
Function Syntax
This function returns outputImage.
// Function prototype
std::shared_ptr< iolink::ImageView > flowInpainting( std::shared_ptr< iolink::ImageView > inputImage, std::shared_ptr< iolink::ImageView > inputMaskImage, int32_t numberOfIterations, double standardDeviation, std::shared_ptr< iolink::ImageView > outputImage = NULL );
This function returns outputImage.
// Function prototype. flow_inpainting( input_image, input_mask_image, number_of_iterations = 10, standard_deviation = 1, output_image = None )
This function returns outputImage.
// Function prototype. public static IOLink.ImageView FlowInpainting( IOLink.ImageView inputImage, IOLink.ImageView inputMaskImage, Int32 numberOfIterations = 10, double standardDeviation = 1, IOLink.ImageView outputImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | nullptr |
![]() |
inputMaskImage |
The input binary image designating the area to reconstruct. It must have same dimensions as the input image. | Image | Binary | nullptr |
![]() |
numberOfIterations |
The number of iterations (must be a positive integer). | Int32 | >=1 | 10 |
![]() |
standardDeviation |
The standard deviation in pixel units. | Float64 | >0 | 1 |
![]() |
outputImage |
The output image. Its dimensions, type, and calibration are forced to the same values as the input. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
input_image |
The input image. | image | Binary, Label, Grayscale or Multispectral | None |
![]() |
input_mask_image |
The input binary image designating the area to reconstruct. It must have same dimensions as the input image. | image | Binary | None |
![]() |
number_of_iterations |
The number of iterations (must be a positive integer). | int32 | >=1 | 10 |
![]() |
standard_deviation |
The standard deviation in pixel units. | float64 | >0 | 1 |
![]() |
output_image |
The output image. Its dimensions, type, and calibration are forced to the same values as the input. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | null |
![]() |
inputMaskImage |
The input binary image designating the area to reconstruct. It must have same dimensions as the input image. | Image | Binary | null |
![]() |
numberOfIterations |
The number of iterations (must be a positive integer). | Int32 | >=1 | 10 |
![]() |
standardDeviation |
The standard deviation in pixel units. | Float64 | >0 | 1 |
![]() |
outputImage |
The output image. Its dimensions, type, and calibration are forced to the same values as the input. | Image | null |
Object Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto foam_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam_sep.vip" ); FlowInpainting flowInpaintingAlgo; flowInpaintingAlgo.setInputImage( foam ); flowInpaintingAlgo.setInputMaskImage( foam_sep ); flowInpaintingAlgo.setNumberOfIterations( 10 ); flowInpaintingAlgo.setStandardDeviation( 1.0 ); flowInpaintingAlgo.execute(); std::cout << "outputImage:" << flowInpaintingAlgo.outputImage()->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) foam_sep = imagedev.read_vip_image(imagedev_data.get_image_path("foam_sep.vip")) flow_inpainting_algo = imagedev.FlowInpainting() flow_inpainting_algo.input_image = foam flow_inpainting_algo.input_mask_image = foam_sep flow_inpainting_algo.number_of_iterations = 10 flow_inpainting_algo.standard_deviation = 1.0 flow_inpainting_algo.execute() print( "output_image:", str( flow_inpainting_algo.output_image ) )
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); ImageView foam_sep = Data.ReadVipImage( @"Data/images/foam_sep.vip" ); FlowInpainting flowInpaintingAlgo = new FlowInpainting { inputImage = foam, inputMaskImage = foam_sep, numberOfIterations = 10, standardDeviation = 1.0 }; flowInpaintingAlgo.Execute(); Console.WriteLine( "outputImage:" + flowInpaintingAlgo.outputImage.ToString() );
Function Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto foam_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam_sep.vip" ); auto result = flowInpainting( foam, foam_sep, 10, 1.0 ); std::cout << "outputImage:" << result->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) foam_sep = imagedev.read_vip_image(imagedev_data.get_image_path("foam_sep.vip")) result = imagedev.flow_inpainting( foam, foam_sep, 10, 1.0 ) print( "output_image:", str( result ) )
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); ImageView foam_sep = Data.ReadVipImage( @"Data/images/foam_sep.vip" ); IOLink.ImageView result = Processing.FlowInpainting( foam, foam_sep, 10, 1.0 ); Console.WriteLine( "outputImage:" + result.ToString() );