GeodesicDistanceMap
Computes a Chamfer distance map considering a mask of forbidden areas.
Access to parameter description
This algorithm computes a Chamfer distance map, like the DistanceMap2d and DistanceMap3d algorithms, but uses a mask to define some forbidden areas.
This algorithm is particularly useful when obstacles are present in the image and should not be taken into account in the distance map.
Use case: Measure of porosity distance.
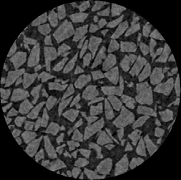
Figure 1. Initial image
Figure 2. Mask of the object (left) and mask of the material (right)
Figure 3. Distance map of the porosity displayed with a rainbow LUT
(taken the outside of object as the starting point of the distance map)
Note: This algorithm does not take into account the input image calibration. The output pixel values are systematically indicating distance in pixel units.
See also
Access to parameter description
This algorithm computes a Chamfer distance map, like the DistanceMap2d and DistanceMap3d algorithms, but uses a mask to define some forbidden areas.
This algorithm is particularly useful when obstacles are present in the image and should not be taken into account in the distance map.
Use case: Measure of porosity distance.
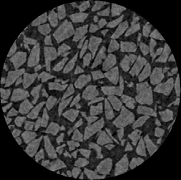
Figure 1. Initial image
![]() |
![]() |
![]() |
![]() |
(taken the outside of object as the starting point of the distance map)
Note: This algorithm does not take into account the input image calibration. The output pixel values are systematically indicating distance in pixel units.
See also
Function Syntax
This function returns outputMapImage.
// Function prototype
std::shared_ptr< iolink::ImageView > geodesicDistanceMap( std::shared_ptr< iolink::ImageView > inputBinaryImage, std::shared_ptr< iolink::ImageView > inputMaskImage, std::shared_ptr< iolink::ImageView > outputMapImage = NULL );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |
---|---|---|---|---|---|
![]() |
inputBinaryImage |
The binary input image. | Image | Binary | nullptr |
![]() |
inputMaskImage |
The binary image for the forbidden area. Distances are computed in regions having a 0 value in this image. It must have same dimensions and type as the input image. | Image | Binary | nullptr |
![]() |
outputMapImage |
The output distance map image. Its dimensions are forced to the same values as the input. Its type is signed 16-bit integer. | Image | nullptr |
Object Examples
auto foam_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam_sep.vip" ); GeodesicDistanceMap geodesicDistanceMapAlgo; geodesicDistanceMapAlgo.setInputBinaryImage( foam_sep ); geodesicDistanceMapAlgo.setInputMaskImage( foam_sep ); geodesicDistanceMapAlgo.execute(); std::cout << "outputMapImage:" << geodesicDistanceMapAlgo.outputMapImage()->toString();
Function Examples
auto foam_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam_sep.vip" ); auto result = geodesicDistanceMap( foam_sep, foam_sep ); std::cout << "outputMapImage:" << result->toString();