TranslateImage2d
Translates a two-dimensional image by a user-defined vector.
Access to parameter description
This algorithm re-assigns the position of the image on the grid according to user-defined vectors. During a translation, there are usually two phenomena: part of the image is out of the bounding box, while another part has no values, as illustrated in Figure 1.
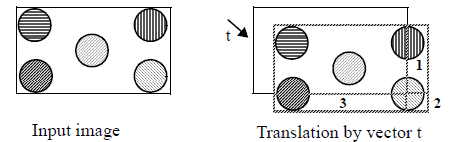
Figure 1. Translation of an image
A translation is denoted by a vector $(t_x,t_y)$. A pixel M of coordinates (x,y) is translated to the new coordinates in the output image as: $$ \left\{\begin{matrix}x'=t_x+x\\y'=t_y+y\end{matrix}\right. $$ or using matrix notation: $$ \begin{bmatrix}x'\\y'\end{bmatrix} = \begin{bmatrix}t_x\\t_y\end{bmatrix} + \begin{bmatrix}x\\y\end{bmatrix} $$ The output image is a discrete space of finite dimensions where the new X and Y coordinates may be outside the image.
There are two possible ways to manage data out of the bounding box:
Access to parameter description
This algorithm re-assigns the position of the image on the grid according to user-defined vectors. During a translation, there are usually two phenomena: part of the image is out of the bounding box, while another part has no values, as illustrated in Figure 1.
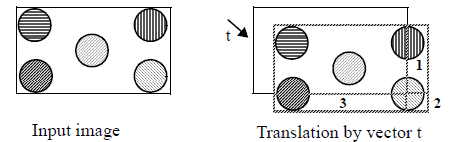
Figure 1. Translation of an image
A translation is denoted by a vector $(t_x,t_y)$. A pixel M of coordinates (x,y) is translated to the new coordinates in the output image as: $$ \left\{\begin{matrix}x'=t_x+x\\y'=t_y+y\end{matrix}\right. $$ or using matrix notation: $$ \begin{bmatrix}x'\\y'\end{bmatrix} = \begin{bmatrix}t_x\\t_y\end{bmatrix} + \begin{bmatrix}x\\y\end{bmatrix} $$ The output image is a discrete space of finite dimensions where the new X and Y coordinates may be outside the image.
There are two possible ways to manage data out of the bounding box:
- The information moved outside of the image area is lost, and a padding value is assigned to the blank
area counterpart.
Figure 2. Translation of an image in FIXED mode - The image is perceived as a cylinder, where the information outside the image area wraps around and is placed in the blank area.
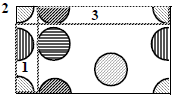
Figure 3. Translation of an image in LOOPED mode
Function Syntax
This function returns outputImage.
// Function prototype
std::shared_ptr< iolink::ImageView > translateImage2d( std::shared_ptr< iolink::ImageView > inputImage, iolink::Vector2i32 translationVector, TranslateImage2d::BackgroundMode backgroundMode, double paddingValue, std::shared_ptr< iolink::ImageView > outputImage = NULL );
This function returns outputImage.
// Function prototype. translate_image_2d( input_image, translation_vector = [100, 100], background_mode = TranslateImage2d.BackgroundMode.FIXED, padding_value = 1, output_image = None )
This function returns outputImage.
// Function prototype. public static IOLink.ImageView TranslateImage2d( IOLink.ImageView inputImage, int[] translationVector = null, TranslateImage2d.BackgroundMode backgroundMode = ImageDev.TranslateImage2d.BackgroundMode.FIXED, double paddingValue = 1, IOLink.ImageView outputImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | nullptr | ||||
![]() |
translationVector |
The translation vector, in pixels. | Vector2i32 | Any value | {100, 100} | ||||
![]() |
backgroundMode |
The mode for managing outside information.
|
Enumeration | FIXED | |||||
![]() |
paddingValue |
The background value, used only in fixed background mode. | Float64 | Any value | 1 | ||||
![]() |
outputImage |
The output image. Its dimensions and type are forced to the same values as the input. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
input_image |
The input image. | image | Binary, Label, Grayscale or Multispectral | None | ||||
![]() |
translation_vector |
The translation vector, in pixels. | vector2i32 | Any value | [100, 100] | ||||
![]() |
background_mode |
The mode for managing outside information.
|
enumeration | FIXED | |||||
![]() |
padding_value |
The background value, used only in fixed background mode. | float64 | Any value | 1 | ||||
![]() |
output_image |
The output image. Its dimensions and type are forced to the same values as the input. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | null | ||||
![]() |
translationVector |
The translation vector, in pixels. | Vector2i32 | Any value | {100, 100} | ||||
![]() |
backgroundMode |
The mode for managing outside information.
|
Enumeration | FIXED | |||||
![]() |
paddingValue |
The background value, used only in fixed background mode. | Float64 | Any value | 1 | ||||
![]() |
outputImage |
The output image. Its dimensions and type are forced to the same values as the input. | Image | null |
Object Examples
std::shared_ptr< iolink::ImageView > polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); TranslateImage2d translateImage2dAlgo; translateImage2dAlgo.setInputImage( polystyrene ); translateImage2dAlgo.setTranslationVector( {100, 100} ); translateImage2dAlgo.setBackgroundMode( TranslateImage2d::BackgroundMode::FIXED ); translateImage2dAlgo.setPaddingValue( 1.0 ); translateImage2dAlgo.execute(); std::cout << "outputImage:" << translateImage2dAlgo.outputImage()->toString();
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) translate_image_2d_algo = imagedev.TranslateImage2d() translate_image_2d_algo.input_image = polystyrene translate_image_2d_algo.translation_vector = [100, 100] translate_image_2d_algo.background_mode = imagedev.TranslateImage2d.FIXED translate_image_2d_algo.padding_value = 1.0 translate_image_2d_algo.execute() print( "output_image:", str( translate_image_2d_algo.output_image ) )
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); TranslateImage2d translateImage2dAlgo = new TranslateImage2d { inputImage = polystyrene, translationVector = new int[]{100, 100}, backgroundMode = TranslateImage2d.BackgroundMode.FIXED, paddingValue = 1.0 }; translateImage2dAlgo.Execute(); Console.WriteLine( "outputImage:" + translateImage2dAlgo.outputImage.ToString() );
Function Examples
std::shared_ptr< iolink::ImageView > polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); auto result = translateImage2d( polystyrene, {100, 100}, TranslateImage2d::BackgroundMode::FIXED, 1.0 ); std::cout << "outputImage:" << result->toString();
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) result = imagedev.translate_image_2d( polystyrene, [100, 100], imagedev.TranslateImage2d.FIXED, 1.0 ) print( "output_image:", str( result ) )
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); IOLink.ImageView result = Processing.TranslateImage2d( polystyrene, new int[]{100, 100}, TranslateImage2d.BackgroundMode.FIXED, 1.0 ); Console.WriteLine( "outputImage:" + result.ToString() );