BinaryCorrelation2d
Performs a logical correlation between a binary two-dimensional image and a binary kernel.
Access to parameter description
For an introduction: section Image Correlation.
The binary correlation $O$ between image $I$ and kernel $K$ is defined as: $$ O(n,m)=O_1(n,m)+O_2(n,m) $$ $$ O_1(n,m)=\sum_{i=1}^{kx} \sum_{j=1}^{ky} \left( K(i,j)\wedge I(n+i-\frac{kx}{2},m+j-\frac{ky}{2}) \right) $$ $$ O_2(n,m)=\sum_{i=1}^{kx} \sum_{j=1}^{ky} \overline{\left( K(i,j)\vee I(n+i-\frac{kx}{2},m+j- \frac{ky}{2}) \right)} $$ where:
At the end of the process, the correlation image is normalized between -1 and 1.
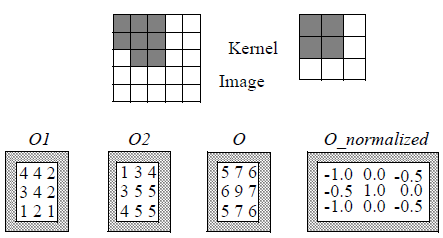
Figure 1 Example of BinaryCorrelation2d
When a part of the kernel lies beyond the edge of the image, the correlation is not computed on the border. In such cases, the values are set to -1E10.
See also
Access to parameter description
For an introduction: section Image Correlation.
The binary correlation $O$ between image $I$ and kernel $K$ is defined as: $$ O(n,m)=O_1(n,m)+O_2(n,m) $$ $$ O_1(n,m)=\sum_{i=1}^{kx} \sum_{j=1}^{ky} \left( K(i,j)\wedge I(n+i-\frac{kx}{2},m+j-\frac{ky}{2}) \right) $$ $$ O_2(n,m)=\sum_{i=1}^{kx} \sum_{j=1}^{ky} \overline{\left( K(i,j)\vee I(n+i-\frac{kx}{2},m+j- \frac{ky}{2}) \right)} $$ where:
- $A \wedge B \Leftrightarrow A~AND~B$
- $A \vee B \Leftrightarrow A~OR~B$
- $ \overline{A} \Leftrightarrow NOT~A $
At the end of the process, the correlation image is normalized between -1 and 1.
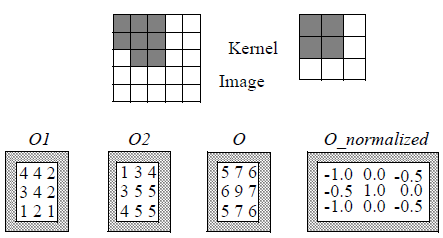
Figure 1 Example of BinaryCorrelation2d
When a part of the kernel lies beyond the edge of the image, the correlation is not computed on the border. In such cases, the values are set to -1E10.
See also
Function Syntax
This function returns a BinaryCorrelation2dOutput structure containing outputImage and outputMeasurement.
// Output structure of the binaryCorrelation2d function. struct BinaryCorrelation2dOutput { /// The output correlation image. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. std::shared_ptr< iolink::ImageView > outputImage; /// The correlation matching results. CorrelationMsr::Ptr outputMeasurement; }; // Function prototype
BinaryCorrelation2dOutput binaryCorrelation2d( std::shared_ptr< iolink::ImageView > inputBinaryImage, std::shared_ptr< iolink::ImageView > inputKernelImage, BinaryCorrelation2d::OffsetMode offsetMode, std::shared_ptr< iolink::ImageView > outputImage = NULL, CorrelationMsr::Ptr outputMeasurement = NULL );
This function returns a tuple containing output_image and output_measurement.
// Function prototype. binary_correlation_2d( input_binary_image, input_kernel_image, offset_mode = BinaryCorrelation2d.OffsetMode.OFFSET_1, output_image = None, output_measurement = None )
This function returns a BinaryCorrelation2dOutput structure containing outputImage and outputMeasurement.
/// Output structure of the BinaryCorrelation2d function. public struct BinaryCorrelation2dOutput { /// /// The output correlation image. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. /// public IOLink.ImageView outputImage; /// The correlation matching results. public CorrelationMsr outputMeasurement; }; // Function prototype. public static BinaryCorrelation2dOutput BinaryCorrelation2d( IOLink.ImageView inputBinaryImage, IOLink.ImageView inputKernelImage, BinaryCorrelation2d.OffsetMode offsetMode = ImageDev.BinaryCorrelation2d.OffsetMode.OFFSET_1, IOLink.ImageView outputImage = null, CorrelationMsr outputMeasurement = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
inputBinaryImage |
The input binary image. | Image | Binary | nullptr | ||||||||
![]() |
inputKernelImage |
The correlation kernel. | Image | Binary | nullptr | ||||||||
![]() |
offsetMode |
The calculation offset, in pixels. The greater this value, computation is faster but detection is less precise.
|
Enumeration | OFFSET_1 | |||||||||
![]() |
outputImage |
The output correlation image. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. | Image | nullptr | |||||||||
![]() |
outputMeasurement |
The correlation matching results. | CorrelationMsr | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
input_binary_image |
The input binary image. | image | Binary | None | ||||||||
![]() |
input_kernel_image |
The correlation kernel. | image | Binary | None | ||||||||
![]() |
offset_mode |
The calculation offset, in pixels. The greater this value, computation is faster but detection is less precise.
|
enumeration | OFFSET_1 | |||||||||
![]() |
output_image |
The output correlation image. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. | image | None | |||||||||
![]() |
output_measurement |
The correlation matching results. | CorrelationMsr | None |
Parameter Name | Description | Type | Supported Values | Default Value | |||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
![]() |
inputBinaryImage |
The input binary image. | Image | Binary | null | ||||||||
![]() |
inputKernelImage |
The correlation kernel. | Image | Binary | null | ||||||||
![]() |
offsetMode |
The calculation offset, in pixels. The greater this value, computation is faster but detection is less precise.
|
Enumeration | OFFSET_1 | |||||||||
![]() |
outputImage |
The output correlation image. Its dimensions are forced to the same values as the input. Its data type is forced to floating point. | Image | null | |||||||||
![]() |
outputMeasurement |
The correlation matching results. | CorrelationMsr | null |
Object Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); BinaryCorrelation2d binaryCorrelation2dAlgo; binaryCorrelation2dAlgo.setInputBinaryImage( polystyrene_sep ); binaryCorrelation2dAlgo.setInputKernelImage( polystyrene_sep ); binaryCorrelation2dAlgo.setOffsetMode( BinaryCorrelation2d::OffsetMode::OFFSET_1 ); binaryCorrelation2dAlgo.execute(); std::cout << "outputImage:" << binaryCorrelation2dAlgo.outputImage()->toString(); std::cout << "minComputed: " << binaryCorrelation2dAlgo.outputMeasurement()->minComputed( 0 ) ;
polystyrene_sep = imagedev.read_vip_image(imagedev_data.get_image_path("polystyrene_sep.vip")) binary_correlation_2d_algo = imagedev.BinaryCorrelation2d() binary_correlation_2d_algo.input_binary_image = polystyrene_sep binary_correlation_2d_algo.input_kernel_image = polystyrene_sep binary_correlation_2d_algo.offset_mode = imagedev.BinaryCorrelation2d.OFFSET_1 binary_correlation_2d_algo.execute() print( "output_image:", str( binary_correlation_2d_algo.output_image ) ) print( "minComputed: ", str( binary_correlation_2d_algo.output_measurement.min_computed( 0 ) ) )
ImageView polystyrene_sep = Data.ReadVipImage( @"Data/images/polystyrene_sep.vip" ); BinaryCorrelation2d binaryCorrelation2dAlgo = new BinaryCorrelation2d { inputBinaryImage = polystyrene_sep, inputKernelImage = polystyrene_sep, offsetMode = BinaryCorrelation2d.OffsetMode.OFFSET_1 }; binaryCorrelation2dAlgo.Execute(); Console.WriteLine( "outputImage:" + binaryCorrelation2dAlgo.outputImage.ToString() ); Console.WriteLine( "minComputed: " + binaryCorrelation2dAlgo.outputMeasurement.minComputed( 0 ) );
Function Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); auto result = binaryCorrelation2d( polystyrene_sep, polystyrene_sep, BinaryCorrelation2d::OffsetMode::OFFSET_1 ); std::cout << "outputImage:" << result.outputImage->toString(); std::cout << "minComputed: " << result.outputMeasurement->minComputed( 0 ) ;
polystyrene_sep = imagedev.read_vip_image(imagedev_data.get_image_path("polystyrene_sep.vip")) result_output_image, result_output_measurement = imagedev.binary_correlation_2d( polystyrene_sep, polystyrene_sep, imagedev.BinaryCorrelation2d.OFFSET_1 ) print( "output_image:", str( result_output_image ) ) print( "minComputed: ", str( result_output_measurement.min_computed( 0 ) ) )
ImageView polystyrene_sep = Data.ReadVipImage( @"Data/images/polystyrene_sep.vip" ); Processing.BinaryCorrelation2dOutput result = Processing.BinaryCorrelation2d( polystyrene_sep, polystyrene_sep, BinaryCorrelation2d.OffsetMode.OFFSET_1 ); Console.WriteLine( "outputImage:" + result.outputImage.ToString() ); Console.WriteLine( "minComputed: " + result.outputMeasurement.minComputed( 0 ) );