DistanceMap2d
Computes the distance map of objects in a two-dimensional binary image.
Access to parameter description
A distance map, also known as distance transform, is a representation of an image where each pixel value corresponds to its distance to the nearest boundary pixel in a given metric.
Two metrics are commonly used:
In the case of the Chessboard metric, the distance is given by: $$ I=d_ C(x,\delta(X)) $$ Where: $$ d_C((i,j),(h,k))=max(|h-i|,|k-j|) $$ The weight used for each neighbor configuration can be user defined. Some optimizations are automatically performed when the weights set correspond to a Chamfer or a Chessboard distance map.
Distance maps are computed on binary images either on object pixels (foreground) or on the background.
The output image can have either a 16-bit signed integer or a 32-bit float type.
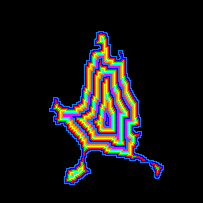
Figure 1. Output image for chessboard distance transform in inside mode
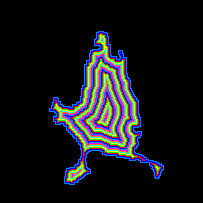
Figure 2. Output image for chamfer distance transform in inside mode
Note: This algorithm does not take into account the input image calibration. The output values are systematically indicating distance in pixel units.
See also
Access to parameter description
A distance map, also known as distance transform, is a representation of an image where each pixel value corresponds to its distance to the nearest boundary pixel in a given metric.
Two metrics are commonly used:
- The Chessboard distance, also known as Chebyshev distance, where all neighbor pixels are considered as a same distance of 1 from a given pixel.
- The Chamfer distance where edge neighbors are considered as a distance of 1 and corner neighbors are considered with a $\sqrt{2}$ weight in order to better approximate a Euclidean distance.
In the case of the Chessboard metric, the distance is given by: $$ I=d_ C(x,\delta(X)) $$ Where: $$ d_C((i,j),(h,k))=max(|h-i|,|k-j|) $$ The weight used for each neighbor configuration can be user defined. Some optimizations are automatically performed when the weights set correspond to a Chamfer or a Chessboard distance map.
Distance maps are computed on binary images either on object pixels (foreground) or on the background.
The output image can have either a 16-bit signed integer or a 32-bit float type.
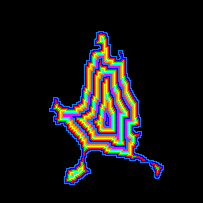
Figure 1. Output image for chessboard distance transform in inside mode
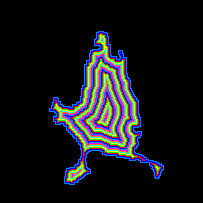
Figure 2. Output image for chamfer distance transform in inside mode
Note: This algorithm does not take into account the input image calibration. The output values are systematically indicating distance in pixel units.
See also
Function Syntax
This function returns outputMapImage.
// Function prototype
std::shared_ptr< iolink::ImageView > distanceMap2d( std::shared_ptr< iolink::ImageView > inputBinaryImage, DistanceMap2d::MappingMode mappingMode, DistanceMap2d::BorderCondition borderCondition, double edgeDistance, double cornerDistance, DistanceMap2d::OutputType outputType, std::shared_ptr< iolink::ImageView > outputMapImage = NULL );
This function returns outputMapImage.
// Function prototype. distance_map_2d( input_binary_image, mapping_mode = DistanceMap2d.MappingMode.INSIDE, border_condition = DistanceMap2d.BorderCondition.MIRROR, edge_distance = 1, corner_distance = 1.41421, output_type = DistanceMap2d.OutputType.SIGNED_INTEGER_16_BIT, output_map_image = None )
This function returns outputMapImage.
// Function prototype. public static IOLink.ImageView DistanceMap2d( IOLink.ImageView inputBinaryImage, DistanceMap2d.MappingMode mappingMode = ImageDev.DistanceMap2d.MappingMode.INSIDE, DistanceMap2d.BorderCondition borderCondition = ImageDev.DistanceMap2d.BorderCondition.MIRROR, double edgeDistance = 1, double cornerDistance = 1.41421, DistanceMap2d.OutputType outputType = ImageDev.DistanceMap2d.OutputType.SIGNED_INTEGER_16_BIT, IOLink.ImageView outputMapImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputBinaryImage |
The binary input image.
Background is represented by zero values while objects are represented by one values. |
Image | Binary | nullptr | ||||
![]() |
mappingMode |
The mode defining where the distance field is computed.
|
Enumeration | INSIDE | |||||
![]() |
borderCondition |
The mode defining the border conditions.
|
Enumeration | MIRROR | |||||
![]() |
edgeDistance |
The distance weight between 2 pixels having an edge connection.
The value must be strictly positive. |
Float64 | >0 | 1 | ||||
![]() |
cornerDistance |
The distance weight between 2 pixels having a corner connection.
The value must be greater than or equal to edgeDistance. |
Float64 | Any value | 1.41421 | ||||
![]() |
outputType |
The output image type to provide.
|
Enumeration | SIGNED_INTEGER_16_BIT | |||||
![]() |
outputMapImage |
The output distance map image.
The distance map of the shape represented by the input image. Each voxel is assigned to a value corresponding to the distance to the nearest object boundary. Its dimensions are forced to the same values as the input. Its data type is defined by the outputType parameter. |
Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
input_binary_image |
The binary input image.
Background is represented by zero values while objects are represented by one values. |
image | Binary | None | ||||
![]() |
mapping_mode |
The mode defining where the distance field is computed.
|
enumeration | INSIDE | |||||
![]() |
border_condition |
The mode defining the border conditions.
|
enumeration | MIRROR | |||||
![]() |
edge_distance |
The distance weight between 2 pixels having an edge connection.
The value must be strictly positive. |
float64 | >0 | 1 | ||||
![]() |
corner_distance |
The distance weight between 2 pixels having a corner connection.
The value must be greater than or equal to edgeDistance. |
float64 | Any value | 1.41421 | ||||
![]() |
output_type |
The output image type to provide.
|
enumeration | SIGNED_INTEGER_16_BIT | |||||
![]() |
output_map_image |
The output distance map image.
The distance map of the shape represented by the input image. Each voxel is assigned to a value corresponding to the distance to the nearest object boundary. Its dimensions are forced to the same values as the input. Its data type is defined by the outputType parameter. |
image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputBinaryImage |
The binary input image.
Background is represented by zero values while objects are represented by one values. |
Image | Binary | null | ||||
![]() |
mappingMode |
The mode defining where the distance field is computed.
|
Enumeration | INSIDE | |||||
![]() |
borderCondition |
The mode defining the border conditions.
|
Enumeration | MIRROR | |||||
![]() |
edgeDistance |
The distance weight between 2 pixels having an edge connection.
The value must be strictly positive. |
Float64 | >0 | 1 | ||||
![]() |
cornerDistance |
The distance weight between 2 pixels having a corner connection.
The value must be greater than or equal to edgeDistance. |
Float64 | Any value | 1.41421 | ||||
![]() |
outputType |
The output image type to provide.
|
Enumeration | SIGNED_INTEGER_16_BIT | |||||
![]() |
outputMapImage |
The output distance map image.
The distance map of the shape represented by the input image. Each voxel is assigned to a value corresponding to the distance to the nearest object boundary. Its dimensions are forced to the same values as the input. Its data type is defined by the outputType parameter. |
Image | null |
Object Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); DistanceMap2d distanceMap2dAlgo; distanceMap2dAlgo.setInputBinaryImage( polystyrene_sep ); distanceMap2dAlgo.setMappingMode( DistanceMap2d::MappingMode::INSIDE ); distanceMap2dAlgo.setBorderCondition( DistanceMap2d::BorderCondition::MIRROR ); distanceMap2dAlgo.setEdgeDistance( 1 ); distanceMap2dAlgo.setCornerDistance( 1.4142135623730951 ); distanceMap2dAlgo.setOutputType( DistanceMap2d::OutputType::SIGNED_INTEGER_16_BIT ); distanceMap2dAlgo.execute(); std::cout << "outputMapImage:" << distanceMap2dAlgo.outputMapImage()->toString();
polystyrene_sep = imagedev.read_vip_image(imagedev_data.get_image_path("polystyrene_sep.vip")) distance_map_2d_algo = imagedev.DistanceMap2d() distance_map_2d_algo.input_binary_image = polystyrene_sep distance_map_2d_algo.mapping_mode = imagedev.DistanceMap2d.INSIDE distance_map_2d_algo.border_condition = imagedev.DistanceMap2d.MIRROR distance_map_2d_algo.edge_distance = 1 distance_map_2d_algo.corner_distance = 1.4142135623730951 distance_map_2d_algo.output_type = imagedev.DistanceMap2d.SIGNED_INTEGER_16_BIT distance_map_2d_algo.execute() print( "output_map_image:", str( distance_map_2d_algo.output_map_image ) )
ImageView polystyrene_sep = Data.ReadVipImage( @"Data/images/polystyrene_sep.vip" ); DistanceMap2d distanceMap2dAlgo = new DistanceMap2d { inputBinaryImage = polystyrene_sep, mappingMode = DistanceMap2d.MappingMode.INSIDE, borderCondition = DistanceMap2d.BorderCondition.MIRROR, edgeDistance = 1, cornerDistance = 1.4142135623730951, outputType = DistanceMap2d.OutputType.SIGNED_INTEGER_16_BIT }; distanceMap2dAlgo.Execute(); Console.WriteLine( "outputMapImage:" + distanceMap2dAlgo.outputMapImage.ToString() );
Function Examples
auto polystyrene_sep = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene_sep.vip" ); auto result = distanceMap2d( polystyrene_sep, DistanceMap2d::MappingMode::INSIDE, DistanceMap2d::BorderCondition::MIRROR, 1, 1.4142135623730951, DistanceMap2d::OutputType::SIGNED_INTEGER_16_BIT ); std::cout << "outputMapImage:" << result->toString();
polystyrene_sep = imagedev.read_vip_image(imagedev_data.get_image_path("polystyrene_sep.vip")) result = imagedev.distance_map_2d( polystyrene_sep, imagedev.DistanceMap2d.INSIDE, imagedev.DistanceMap2d.MIRROR, 1, 1.4142135623730951, imagedev.DistanceMap2d.SIGNED_INTEGER_16_BIT ) print( "output_map_image:", str( result ) )
ImageView polystyrene_sep = Data.ReadVipImage( @"Data/images/polystyrene_sep.vip" ); IOLink.ImageView result = Processing.DistanceMap2d( polystyrene_sep, DistanceMap2d.MappingMode.INSIDE, DistanceMap2d.BorderCondition.MIRROR, 1, 1.4142135623730951, DistanceMap2d.OutputType.SIGNED_INTEGER_16_BIT ); Console.WriteLine( "outputMapImage:" + result.ToString() );