Rotate2d
Applies a rotation of a given angle on a two-dimensional image arround a given point or the center of the image.
Access to parameter description
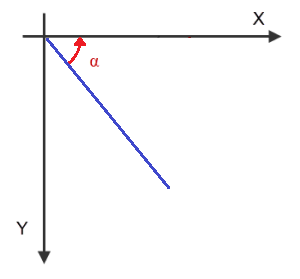
Figure 1. The angle corresponds to this convention for defining the orientation
Access to parameter description
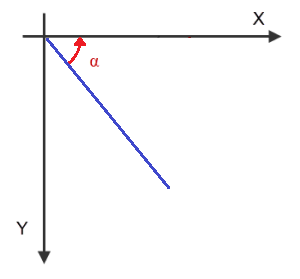
Figure 1. The angle corresponds to this convention for defining the orientation
Function Syntax
This function returns outputImage.
// Function prototype
std::shared_ptr< iolink::ImageView > rotate2d( std::shared_ptr< iolink::ImageView > inputImage, double rotationAngle, Rotate2d::CenterMode centerMode, iolink::Vector2d rotationCenter, Rotate2d::InterpolationType interpolationType, bool outputResizing, double paddingValue, std::shared_ptr< iolink::ImageView > outputImage = NULL );
This function returns outputImage.
// Function prototype. rotate_2d( input_image, rotation_angle = 90, center_mode = Rotate2d.CenterMode.IMAGE_CENTER, rotation_center = [0, 0], interpolation_type = Rotate2d.InterpolationType.NEAREST_NEIGHBOR, output_resizing = True, padding_value = 0, output_image = None )
This function returns outputImage.
// Function prototype. public static IOLink.ImageView Rotate2d( IOLink.ImageView inputImage, double rotationAngle = 90, Rotate2d.CenterMode centerMode = ImageDev.Rotate2d.CenterMode.IMAGE_CENTER, double[] rotationCenter = null, Rotate2d.InterpolationType interpolationType = ImageDev.Rotate2d.InterpolationType.NEAREST_NEIGHBOR, bool outputResizing = true, double paddingValue = 0, IOLink.ImageView outputImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | nullptr | ||||
![]() |
rotationAngle |
The angle of the rotation in degrees. | Float64 | Any value | 90 | ||||
![]() |
centerMode |
The way to define the rotation center
|
Enumeration | IMAGE_CENTER | |||||
![]() |
rotationCenter |
The rotation center coordinates. This parameter is ignored in IMAGE_CENTER mode. | Vector2d | Any value | {0.f, 0.f} | ||||
![]() |
interpolationType |
The interpolation mode. Method used to calculate the intensity of each pixel in the result image.
|
Enumeration | NEAREST_NEIGHBOR | |||||
![]() |
outputResizing |
Resize the output image to make all input data visible in the output image if set to True. Preserve the input dimension if set to False. | Bool | true | |||||
![]() |
paddingValue |
The background value for pixels with no correpondant point in the input image. | Float64 | Any value | 0 | ||||
![]() |
outputImage |
The output image. Its type is forced to the same values as the input. Its dimensions are identical to or greater than the input image, according to the outputResizing parameter. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
input_image |
The input image. | image | Binary, Label, Grayscale or Multispectral | None | ||||
![]() |
rotation_angle |
The angle of the rotation in degrees. | float64 | Any value | 90 | ||||
![]() |
center_mode |
The way to define the rotation center
|
enumeration | IMAGE_CENTER | |||||
![]() |
rotation_center |
The rotation center coordinates. This parameter is ignored in IMAGE_CENTER mode. | vector2d | Any value | [0, 0] | ||||
![]() |
interpolation_type |
The interpolation mode. Method used to calculate the intensity of each pixel in the result image.
|
enumeration | NEAREST_NEIGHBOR | |||||
![]() |
output_resizing |
Resize the output image to make all input data visible in the output image if set to True. Preserve the input dimension if set to False. | bool | True | |||||
![]() |
padding_value |
The background value for pixels with no correpondant point in the input image. | float64 | Any value | 0 | ||||
![]() |
output_image |
The output image. Its type is forced to the same values as the input. Its dimensions are identical to or greater than the input image, according to the outputResizing parameter. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | null | ||||
![]() |
rotationAngle |
The angle of the rotation in degrees. | Float64 | Any value | 90 | ||||
![]() |
centerMode |
The way to define the rotation center
|
Enumeration | IMAGE_CENTER | |||||
![]() |
rotationCenter |
The rotation center coordinates. This parameter is ignored in IMAGE_CENTER mode. | Vector2d | Any value | {0f, 0f} | ||||
![]() |
interpolationType |
The interpolation mode. Method used to calculate the intensity of each pixel in the result image.
|
Enumeration | NEAREST_NEIGHBOR | |||||
![]() |
outputResizing |
Resize the output image to make all input data visible in the output image if set to True. Preserve the input dimension if set to False. | Bool | true | |||||
![]() |
paddingValue |
The background value for pixels with no correpondant point in the input image. | Float64 | Any value | 0 | ||||
![]() |
outputImage |
The output image. Its type is forced to the same values as the input. Its dimensions are identical to or greater than the input image, according to the outputResizing parameter. | Image | null |
Object Examples
std::shared_ptr< iolink::ImageView > polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); Rotate2d rotate2dAlgo; rotate2dAlgo.setInputImage( polystyrene ); rotate2dAlgo.setRotationAngle( 40.5 ); rotate2dAlgo.setCenterMode( Rotate2d::CenterMode::IMAGE_CENTER ); rotate2dAlgo.setRotationCenter( {15.5, 32} ); rotate2dAlgo.setInterpolationType( Rotate2d::InterpolationType::NEAREST_NEIGHBOR ); rotate2dAlgo.setOutputResizing( true ); rotate2dAlgo.setPaddingValue( 0 ); rotate2dAlgo.execute(); std::cout << "outputImage:" << rotate2dAlgo.outputImage()->toString();
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) rotate_2d_algo = imagedev.Rotate2d() rotate_2d_algo.input_image = polystyrene rotate_2d_algo.rotation_angle = 40.5 rotate_2d_algo.center_mode = imagedev.Rotate2d.IMAGE_CENTER rotate_2d_algo.rotation_center = [15.5, 32] rotate_2d_algo.interpolation_type = imagedev.Rotate2d.NEAREST_NEIGHBOR rotate_2d_algo.output_resizing = True rotate_2d_algo.padding_value = 0 rotate_2d_algo.execute() print( "output_image:", str( rotate_2d_algo.output_image ) )
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); Rotate2d rotate2dAlgo = new Rotate2d { inputImage = polystyrene, rotationAngle = 40.5, centerMode = Rotate2d.CenterMode.IMAGE_CENTER, rotationCenter = new double[]{15.5, 32}, interpolationType = Rotate2d.InterpolationType.NEAREST_NEIGHBOR, outputResizing = true, paddingValue = 0 }; rotate2dAlgo.Execute(); Console.WriteLine( "outputImage:" + rotate2dAlgo.outputImage.ToString() );
Function Examples
std::shared_ptr< iolink::ImageView > polystyrene = ioformat::readImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "polystyrene.tif" ); auto result = rotate2d( polystyrene, 40.5, Rotate2d::CenterMode::IMAGE_CENTER, {15.5, 32}, Rotate2d::InterpolationType::NEAREST_NEIGHBOR, true, 0 ); std::cout << "outputImage:" << result->toString();
polystyrene = ioformat.read_image(imagedev_data.get_image_path("polystyrene.tif")) result = imagedev.rotate_2d( polystyrene, 40.5, imagedev.Rotate2d.IMAGE_CENTER, [15.5, 32], imagedev.Rotate2d.NEAREST_NEIGHBOR, True, 0 ) print( "output_image:", str( result ) )
ImageView polystyrene = ViewIO.ReadImage( @"Data/images/polystyrene.tif" ); IOLink.ImageView result = Processing.Rotate2d( polystyrene, 40.5, Rotate2d.CenterMode.IMAGE_CENTER, new double[]{15.5, 32}, Rotate2d.InterpolationType.NEAREST_NEIGHBOR, true, 0 ); Console.WriteLine( "outputImage:" + result.ToString() );