TranslateImage3d
Translates a three-dimensional image by a user-defined vector.
Access to parameter description
This algorithm re-assigns the position of the image on the grid according to user-defined vectors. During a translation, there are usually two phenomena: part of the image is out of the window, while another part has no values, as illustrated in Figure 1.
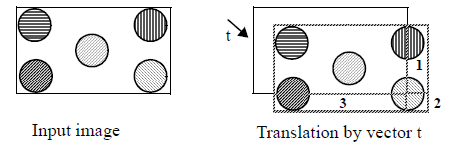
Figure 1. Translation of an image
A translation is denoted by a vector $(t_x,t_y,t_z)$. A pixel M of coordinates (x,y,z) is translated to the new coordinates in the output image as: $$ \left\{\begin{matrix}x'=t_x+x\\y'=t_y+y\\z'=t_z+z\end{matrix}\right. $$ or using matrix notation: $$ \begin{bmatrix}x'\\y'\\z'\end{bmatrix} = \begin{bmatrix}t_x\\t_y\\t_z\end{bmatrix} + \begin{bmatrix}x\\y\\z\end{bmatrix} $$ The output image is a discrete space of finite dimensions where the new X, Y, and Z coordinates may be outside the image.
There are two possible ways to manage data out of the bounding box:
Access to parameter description
This algorithm re-assigns the position of the image on the grid according to user-defined vectors. During a translation, there are usually two phenomena: part of the image is out of the window, while another part has no values, as illustrated in Figure 1.
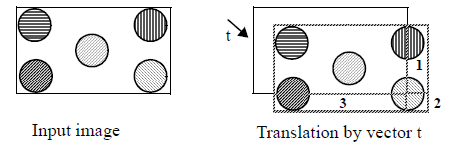
Figure 1. Translation of an image
A translation is denoted by a vector $(t_x,t_y,t_z)$. A pixel M of coordinates (x,y,z) is translated to the new coordinates in the output image as: $$ \left\{\begin{matrix}x'=t_x+x\\y'=t_y+y\\z'=t_z+z\end{matrix}\right. $$ or using matrix notation: $$ \begin{bmatrix}x'\\y'\\z'\end{bmatrix} = \begin{bmatrix}t_x\\t_y\\t_z\end{bmatrix} + \begin{bmatrix}x\\y\\z\end{bmatrix} $$ The output image is a discrete space of finite dimensions where the new X, Y, and Z coordinates may be outside the image.
There are two possible ways to manage data out of the bounding box:
- The information moved outside of the image area is lost, and a padding value is assigned to the blank
area counterpart.
Figure 2. Translation of an image in FIXED mode
- The image is perceived as a cylinder, where the information outside the image area wraps around and is
placed in the blank area.
Figure 3. Translation of an image in LOOPED mode
Function Syntax
This function returns outputImage.
// Function prototype
std::shared_ptr< iolink::ImageView > translateImage3d( std::shared_ptr< iolink::ImageView > inputImage, iolink::Vector3i32 translationVector, TranslateImage3d::BackgroundMode backgroundMode, double paddingValue, std::shared_ptr< iolink::ImageView > outputImage = nullptr );
This function returns outputImage.
// Function prototype. translate_image_3d(input_image: idt.ImageType, translation_vector: Iterable[int] = [100, 100, 100], background_mode: TranslateImage3d.BackgroundMode = TranslateImage3d.BackgroundMode.FIXED, padding_value: float = 1, output_image: idt.ImageType = None) -> idt.ImageType
This function returns outputImage.
// Function prototype. public static IOLink.ImageView TranslateImage3d( IOLink.ImageView inputImage, int[] translationVector = null, TranslateImage3d.BackgroundMode backgroundMode = ImageDev.TranslateImage3d.BackgroundMode.FIXED, double paddingValue = 1, IOLink.ImageView outputImage = null );
Class Syntax
Parameters
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | nullptr | ||||
![]() |
translationVector |
The translation vector in voxels. | Vector3i32 | Any value | {100, 100, 100} | ||||
![]() |
backgroundMode |
The mode for managing outside information.
|
Enumeration | FIXED | |||||
![]() |
paddingValue |
The background value, used only in fixed background mode. | Float64 | Any value | 1 | ||||
![]() |
outputImage |
The output image. Its dimensions and type are forced to the same values as the input. | Image | nullptr |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
input_image |
The input image. | image | Binary, Label, Grayscale or Multispectral | None | ||||
![]() |
translation_vector |
The translation vector in voxels. | vector3i32 | Any value | [100, 100, 100] | ||||
![]() |
background_mode |
The mode for managing outside information.
|
enumeration | FIXED | |||||
![]() |
padding_value |
The background value, used only in fixed background mode. | float64 | Any value | 1 | ||||
![]() |
output_image |
The output image. Its dimensions and type are forced to the same values as the input. | image | None |
Parameter Name | Description | Type | Supported Values | Default Value | |||||
---|---|---|---|---|---|---|---|---|---|
![]() |
inputImage |
The input image. | Image | Binary, Label, Grayscale or Multispectral | null | ||||
![]() |
translationVector |
The translation vector in voxels. | Vector3i32 | Any value | {100, 100, 100} | ||||
![]() |
backgroundMode |
The mode for managing outside information.
|
Enumeration | FIXED | |||||
![]() |
paddingValue |
The background value, used only in fixed background mode. | Float64 | Any value | 1 | ||||
![]() |
outputImage |
The output image. Its dimensions and type are forced to the same values as the input. | Image | null |
Object Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); TranslateImage3d translateImage3dAlgo; translateImage3dAlgo.setInputImage( foam ); translateImage3dAlgo.setTranslationVector( {100, 100, 100} ); translateImage3dAlgo.setBackgroundMode( TranslateImage3d::BackgroundMode::FIXED ); translateImage3dAlgo.setPaddingValue( 1.0 ); translateImage3dAlgo.execute(); std::cout << "outputImage:" << translateImage3dAlgo.outputImage()->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) translate_image_3d_algo = imagedev.TranslateImage3d() translate_image_3d_algo.input_image = foam translate_image_3d_algo.translation_vector = [100, 100, 100] translate_image_3d_algo.background_mode = imagedev.TranslateImage3d.FIXED translate_image_3d_algo.padding_value = 1.0 translate_image_3d_algo.execute() print("output_image:", str(translate_image_3d_algo.output_image))
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); TranslateImage3d translateImage3dAlgo = new TranslateImage3d { inputImage = foam, translationVector = new int[]{100, 100, 100}, backgroundMode = TranslateImage3d.BackgroundMode.FIXED, paddingValue = 1.0 }; translateImage3dAlgo.Execute(); Console.WriteLine( "outputImage:" + translateImage3dAlgo.outputImage.ToString() );
Function Examples
auto foam = readVipImage( std::string( IMAGEDEVDATA_IMAGES_FOLDER ) + "foam.vip" ); auto result = translateImage3d( foam, {100, 100, 100}, TranslateImage3d::BackgroundMode::FIXED, 1.0 ); std::cout << "outputImage:" << result->toString();
foam = imagedev.read_vip_image(imagedev_data.get_image_path("foam.vip")) result = imagedev.translate_image_3d(foam, [100, 100, 100], imagedev.TranslateImage3d.FIXED, 1.0) print("output_image:", str(result))
ImageView foam = Data.ReadVipImage( @"Data/images/foam.vip" ); IOLink.ImageView result = Processing.TranslateImage3d( foam, new int[]{100, 100, 100}, TranslateImage3d.BackgroundMode.FIXED, 1.0 ); Console.WriteLine( "outputImage:" + result.ToString() );